Sign in to follow this
Followers
0
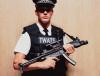
Script efficiency
By
Double Doppler, in ARMA 2 & OA : MISSIONS - Editing & Scripting
By
Double Doppler, in ARMA 2 & OA : MISSIONS - Editing & Scripting