Sign in to follow this
Followers
0
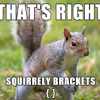
Which is more efficient - addAction condition or trigger condition?
By
Heeeere's johnny!, in ARMA 3 - MISSION EDITING & SCRIPTING
By
Heeeere's johnny!, in ARMA 3 - MISSION EDITING & SCRIPTING