Sign in to follow this
Followers
0
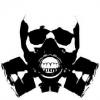
Accessing elements of an array effeciently
By
iceman77, in ARMA 2 & OA : MISSIONS - Editing & Scripting
By
iceman77, in ARMA 2 & OA : MISSIONS - Editing & Scripting