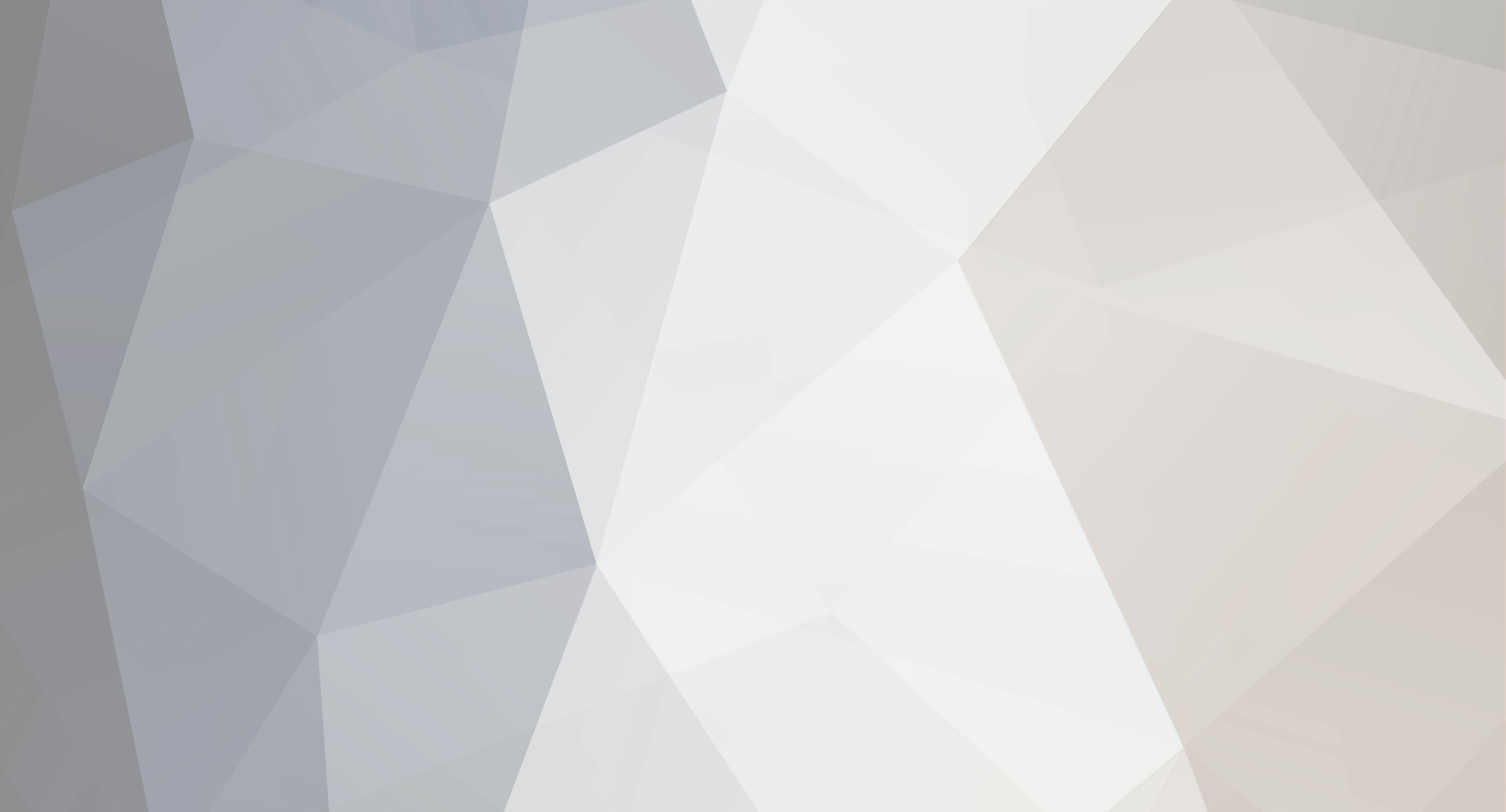

maca134
Member-
Content Count
22 -
Joined
-
Last visited
-
Medals
-
maca134 started following Arma DllExport - C\# Extensions, Arma (De)Serializer - C\# Extensions and 3DEN Better Inventory
-
csharp Arma (De)Serializer - C# Extensions
maca134 posted a topic in ARMA 3 - ADDONS & MODS: COMPLETE
This is a package that contains a JsonConvertor for the Newtonsoft.Json package in C#. It's purpose is to convert between C# data types and ARMA data types. If you have used Newtonsoft.Json you will be familar with mapping C# properties to Json properties and vise versa. You can download it here https://www.nuget.org/packages/Maca134.Arma.Serializer/ or Maca134.Arma.Serializer in Nuget. Also source is on Github https://github.com/maca134/Maca134.Arma.Serializer. Here is a very basic example: public class TestClass { public string Var1 { get; set; } public string[] Var1A { get; set; } public int Var2 { get; set; } public float Var3 { get; set; } public bool Var4 { get; set; } public TestClassInner Var5 { get; set; } public List<string> Var1B { get; set; } } public class TestClassInner { public string Var1 { get; set; } public int Var2 { get; set; } public float Var3 { get; set; } public bool Var4 { get; set; } } var testData = new TestClass { Var1 = "he\"llo", Var1A = new[] {"1", "2", "3"}, Var1B = new List<string> { "1", "2", "3" }, Var2 = 1, Var3 = 1.2f, Var4 = false, Var5 = new TestClassInner { Var1 = "h\"ello", Var2 = 1, Var3 = 1.2f, Var4 = false, } }; /* Returns: ["he""llo",["1","2","3"],1,1.2,false,["h""ello",1,1.2,false],["1","2","3"]] */ var str = ArmaArrayConvert.SerializeObject(testData); /* Returns the reconstructed object */ var obj = ArmaArrayConvert.DeserializeObject<TestClass>(str); I fairly sure I have covered all the types but there maybe missing bits. -
After using RGiesecke's DllExport package for years for getting C# ARMA extensions working, I wanted to know exactly how it worked and in the process ended up writing a DllExport package specific for ARMA's exports. You can download it here https://www.nuget.org/packages/Maca134.Arma.DllExport/ or Maca134.Arma.DllExport in Nuget. Also source is on Github https://github.com/maca134/Maca134.Arma.DllExport. Here is the old way of doing it (64bit): [DllExport("RVExtension", CallingConvention = System.Runtime.InteropServices.CallingConvention.Winapi)] public static void RVExtension(StringBuilder output, int outputSize, [MarshalAs(UnmanagedType.LPStr)] string function) { output.Append(function); } With the new package it simplifies the code into: [ArmaDllExport] public static string Invoke(string function, int outputSize) { return function; } The package will also switch the export name depending on bit'ness of the DLL.
-
For some video work I am doing at the moment, I wrote a small addon for 3DEN to make it easier editing the inventory of vehicles/containers. Download it on Steam Workshop: http://steamcommunity.com/workshop/filedetails/?id=1124993203 The mod does not add any additional dependencies to the mission as it uses the existing ammo attribute that is used by vanilla ARMA.
-
[BETA] Open Chernarus Project - Plug AND Play - All Map Support - Dynamic Simulation
maca134 replied to DirtySanchez's topic in ARMA 3 - ADDONS & MODS: COMPLETE
Full of bugs, not bin'ed, over a gig in size and using SQF to replace buildings. Here is another version of the buildings that have been properly setup with LODs, AI paths etc -
OCAP - Op Capture And Playback (AAR/Replay)
maca134 replied to Gudsawn's topic in ARMA 3 - ADDONS & MODS: COMPLETE
Thankyou very much :D -
OCAP - Op Capture And Playback (AAR/Replay)
maca134 replied to Gudsawn's topic in ARMA 3 - ADDONS & MODS: COMPLETE
A small drop of credit would of been nice, considering you copy-pasted from my project. https://github.com/mistergoodson/OCAP/blob/master/extension/OCAPExporter/OCAPExporter/Class1.cs#L64 https://github.com/maca134/ARMAExtCS/blob/master/ARMAExtCS/DllEntry.cs#L13 -
OCAP - Op Capture And Playback (AAR/Replay)
maca134 replied to Gudsawn's topic in ARMA 3 - ADDONS & MODS: COMPLETE
Nice work, extension look good too ;) -
maca134 started following ARMA C\# & JS (NodeJS) Extension - Compile on the fly
-
Need help writing an extension in C# for A3
maca134 replied to d0nar's topic in ARMA 3 - ADDONS - CONFIGS & SCRIPTING
Hey take a look at this code here https://github.com/maca134/armaext/blob/master/project/armaextconsole/ARMAExt.cs this is what i use to test extensions before loading it into arma. Also this project https://github.com/maca134/armaext can be helpful testing code, without having to recompile and restart arma each time you make a change. -
ARMA C# & JS (NodeJS) Extension - Compile on the fly
maca134 posted a topic in ARMA 3 - ADDONS & MODS: COMPLETE
Download This is an extension/mod that will allow you to compile/run C# on the fly to ease rapid development of a c# ARMA extension The mod has only 2 functions: Load some c# or javascript, returns a pointer _pointer = [_path_to_cs] call ARMAEXT_fnc_load Run the script and return the results _result = [_pointer, _args] call ARMAEXT_fnc_run; The c# has to implement the follow pattern so its as close as possible to the actual DllExport: class Startup { public static stirng Invoke(string input) { return "Hello World"; } } All paths starting .\ will be relative to ARMACS.dll Additional libraries can be loaded as follows: #r ".\lib.dll" or #r "c:\path\to\lib.dll or #r "System.Data.dll" Output is set to 10k If you use this on clients, DISABLE BATTLEYE! This work is licensed under Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International License. -
Are you allowed to use these markers on BIS approved monetized servers?
-
Regarding launching i found that commandline, calling arma2oa_be gets you to the lobby then instantly get Session Lost but if you join via Steam URL, all is good ArmA2OA_BE.exe 0 0 -noSplash -noPause -connect=192.223.24.226 -port=2302 "-mod=C:\SteamLibrary\steamapps\common\Arma 2;Expansion;C:\mods\@DayzOverwatch" Result: Join lobby then instantly get "Session Lost" steam://run/33930//-noSplash -noPause -connect=192.223.24.226 -port=2302 "-mod=C:\SteamLibrary\steamapps\common\Arma 2;Expansion;C:\mods\@DayzOverwatch" URL encoded: steam://run/33930//-noSplash%20-noPause%20-connect%3D192.223.24.226%20-port%3D2302%20%22-mod%3DG%3A%5CSteamLibrary%5Csteamapps%5Ccommon%5CArma%202%3BExpansion%3BU%3A%5Cmods%5C%40DayzOverwatch%22 Result: Join lobby fine
-
Im also getting this problem with a community launcher
-
MBCon - Server RCON tool (BEC replacement)
maca134 replied to maca134's topic in ARMA 3 - COMMUNITY MADE UTILITIES
I will check it out, i personally use fire daemon to restart. Also is the plugin in debug mode? -
MBCon - Server RCON tool (BEC replacement)
maca134 replied to maca134's topic in ARMA 3 - COMMUNITY MADE UTILITIES
Cheers foxhound :) -
nRadio - Stream Internet Radio
maca134 replied to maca134's topic in ARMA 3 - ADDONS & MODS: COMPLETE
IT might be just that the latency on the stream is shit. I will see about some buffering options.