Sign in to follow this
Followers
0
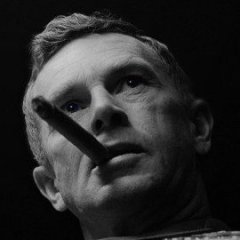
Haul Cargo
By
Alleged Accomplice, in ARMA 3 - MISSION EDITING & SCRIPTING
By
Alleged Accomplice, in ARMA 3 - MISSION EDITING & SCRIPTING