Sign in to follow this
Followers
0
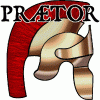
Performance Profiling your Functions
By
wolffy.au, in ARMA 2 & OA : MISSIONS - Editing & Scripting
By
wolffy.au, in ARMA 2 & OA : MISSIONS - Editing & Scripting