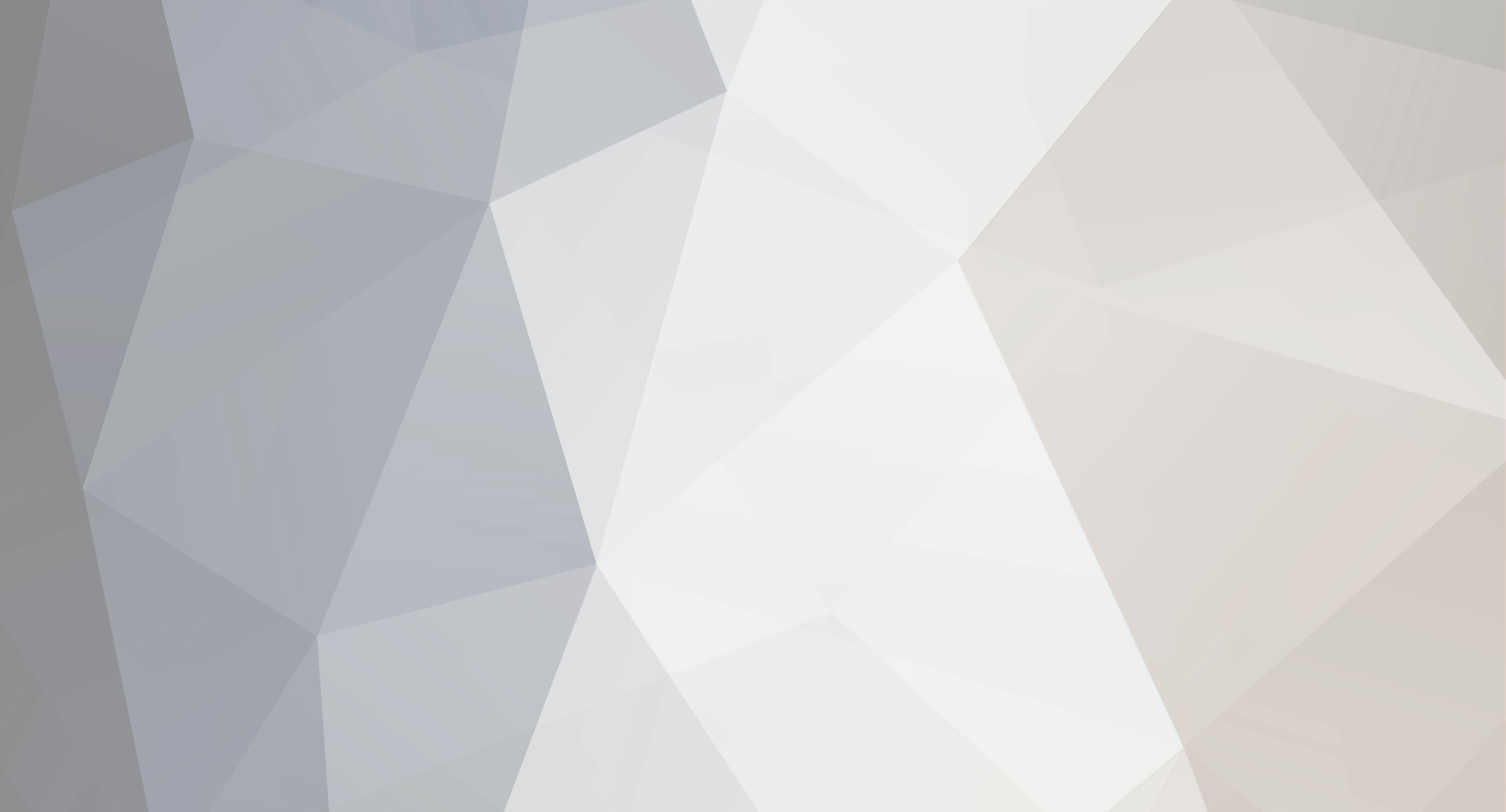

rcdxph
Member-
Content Count
20 -
Joined
-
Last visited
-
Medals
Community Reputation
10 GoodAbout rcdxph
-
Rank
Private First Class
-
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
well, what is the progress? -
The MM5 (short for Fifth-Generation Penn State/NCAR Mesoscale Model) should be used into the ARMA3.
-
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
Well what's news? ^_^ï¼ -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
well,guys: i find some codes using for record the events during the simulation ,the codes are created by the sofeware multigen vega, it is named the "vgVCR",hope to help you! it is c++ codes created by VC++6.0 ! /* ============================================================================ $Revision: 1.13 $ $Date: 1996/12/06 04:44:20 $ ============================================================================ PARADIGM SIMULATION INCORPORATED Copyright 1996 by Paradigm Simulation, Inc. No part of this source code may be reproduced or distrubuted in any form or by any means, or stored in a database or retrieval system, without the prior written consent of Paradigm Simulation, Inc. ============================================================================ */ #include <stdio.h> /* definition of printf */ #include <stdlib.h> /* definition of exit */ #include <vg.h> /* include file for Vega */ #include <pf.h> /* include file for Performer */ #include <vgfx.h> /* Vega special effects public hdr */ #include "vgvcr.h" /* public vegaVCR header file */ #define VCR_FILE "./default01.vcr" /* * Function Prototypes */ static void processKey( vgWindow *win ); static void createVCR( void ); static void TimeDrawCallback( vgCommon *chan, void *udata ); static void handleKey( int key ); static void toggleProp( void *handle, int what ); vgVCR *vcr; /* current VCR enabled for keyboard input */ vgScene *scene; vgEnv *env; float tod; vgMotion *mot; vgChannel *chan; vgGfx *gfx; float cw, ch; /*************************************************************************** * * main() * * Example of a writing code to create and use VCRs. vcrAPI.c * demonstrates a keyboard input only application. This sample * also demonstrates VegaVCR's ability to record objects that are * dynamically added to and removed from a scene. The blimp object * is dynamically added to and removed from the scene. * ***************************************************************************/ void main ( int argc , char *argv[] ) { vgWindow *win; vgObserver *main_obs; vgObject *blimp; vgPosition *pos; /* temporary position */ vgMat mat; /* work matrix */ unsigned added = FALSE; unsigned deleted = FALSE; double addTime; double delTime; if ( argc < 2 ) { printf ( "syntax : %s <config file>\n" , argv[0] ); exit ( -1 ); } /* Initialize, define, and configure the system */ vgInitSys(); vgInitFx(); /* initialize special effects */ vgInitVCR(); /* initialize VCR */ vgDefineSys( argv[1] ); vgConfigSys(); win = vgGetWin( 0 ); blimp = vgFindObj( "blimp" ); scene = vgGetScene( 0 ); env = vgGetEnv( 0 ); main_obs = vgFindObserv( "main" ); /* locate the main observer */ tod = vgGetProp( env, VGENV_TOD ); mot = vgGetMot( 0 ); chan = vgGetChan( 0 ); gfx = vgGetGfx( 0 ); /* * Create and enable VCR 0 for input */ createVCR(); addTime = vgGetFrameTime() + 20.0; /* add blimp 50 secs from now */ delTime = vgGetFrameTime() + 30.0; /* del blimp 65 secs from now */ pos = vgNewPos(); vgIdentMat( mat ); /* the real-time loop */ while ( 1 ) { if ( !((int) vgGetProp( vcr, VGVCR_STATE ) & VGVCR_PLAY) ) { if ( !added && (vgGetFrameTime() > addTime) ) { if ( blimp) { vgAddSceneObj( scene, blimp ); added = TRUE; } else vgNotify( VG_NOTICE, VG_APP, "Blimp object could not be found\n" ); } if ( added && !deleted && (vgGetFrameTime() > delTime) ) { deleted = TRUE; vgRemSceneObj( scene, blimp ); } } vgSyncFrame (); vgFrame (); processKey( win ); } vgExit( 0 ); } /* * Keyboard only input for this sample - to make sure users can indeed * have just keyboard input if they so desire */ enum Key { RECORD = VGWIN_ALTR, PLAY = VGWIN_ALTP, STOP = VGWIN_ALTS, PAUSE = VGWIN_ALTX, REWIND = VGWIN_ALTW, FF = VGWIN_ALTF }; /*************************************************************************** * * processKey() * ***************************************************************************/ void processKey( vgWindow *win ) { int key = vgGetWinKey( win ); if ( key ) { if ( key <= '9' && key > '0' ) /* 1-9 */ { unsigned i = key - '0' - 1; /* key 1 corresponds to VCR 0 */ if ( vgGetVCR( i ) ) vcr = vgGetVCR( i ); return; } if ( vcr ) switch ( (int) vgGetProp( vcr, VGVCR_STATE ) ) { case VGVCR_RECORD: if ( key == STOP ) vgVCRStop( vcr ); else if ( key == PLAY ) { vgVCRStop( vcr ); vgVCRPlay( vcr ); } else if ( key == PAUSE ) vgVCRPause( vcr ); else handleKey( key ); break; case VGVCR_PLAY: case VGVCR_FF: if ( key == STOP ) vgVCRStop( vcr ); else if ( key == PAUSE ) vgVCRPause( vcr ); else if ( key == FF ) vgVCRFF( vcr ); else if ( key == REWIND ) vgVCRRewind( vcr ); else if ( key == PLAY ) vgVCRPlay( vcr ); /* disable FF */ else handleKey( key ); break; case VGVCR_STOP: if ( key == RECORD ) vgVCRRecord( vcr ); else if ( key == PLAY ) vgVCRPlay( vcr ); else if ( key == REWIND ) vgVCRRewind( vcr ); else handleKey( key ); break; default: /* VGVCR_PAUSE */ if ( (((int) vgGetProp( vcr, VGVCR_STATE ) & VGVCR_RECORD) && (key == PAUSE || key == RECORD)) || (((int) vgGetProp( vcr, VGVCR_STATE ) & VGVCR_PLAY) && (key == PAUSE || key == PLAY)) ) vgVCRPause( vcr ); else if ( key == STOP ) vgVCRStop( vcr ); else handleKey( key ); break; } } } /*************************************************************************** * * createVCR() * ***************************************************************************/ static void createVCR() { if ( (vcr = vgNewVCR()) ) { vgSystem *sys = vgGetSys(); vgName( vcr, "CNN" ); vgVCRInFileName( vcr, VCR_FILE ); vgVCROutFileName( vcr, VCR_FILE ); vgProp( vcr, VGVCR_INTERPOLATE, VG_OFF ); vgProp( vcr, VGVCR_FREQUENCY, 15 ); /* 15 Hz */ vgProp( vcr, VGVCR_VCRLIST, VGVCR_ENV ); /* all env */ vgVCRRegisterInstance( vcr, vgFindObserv( "main" ), NULL ); vgVCRRegisterInstance( vcr, vgFindPlyr( "car" ), NULL ); /* * Record the parts of the car, but do not record the parts of the blimp */ vgVCRRegisterInstance( vcr, vgFindObj( "car" ), (void *) VGVCR_PARTS ); vgVCRRegisterInstance( vcr, vgFindObj( "blimp" ), NULL ); vgMakeVCR( vcr ); vgVCRRegisterInstance( vcr, vgFindFx( "smoke" ), NULL ); vgVCRRegisterInstance( vcr, vgFindFx( "fire" ), NULL ); #ifndef IRISGL /* calculations for OpenGL only */ { int wl, wr, wb, wt; float cl, cr, cb, ct; vgWindow *win; win = vgGetChanWin( chan ); vgGetWinSize( win, &wl, &wr, &wb, &wt ); vgGetChanViewport( chan, &cl, &cr, &cb, &ct ); cw = (cr - cl) * (wr - wl); ch = (ct - cb) * (wt - wb); } #endif vgAddFunc( chan, VGCHAN_POSTDRAW, TimeDrawCallback, NULL ); } } #define kBoxL 10 #define kBoxB 10 static char *states[] = { "REWIND", /* 0x000 */ "STOP", /* 0x001 */ "RECORD", /* 0x002 */ "", "PLAY", /* 0x004 */ "FAST FORWARD", /* 0x005 */ "", "", "PAUSE", /* 0x008 */ "", "PAUSE", /* 0x00A pause from rec */ "", "PAUSE", /* 0x00B pause from play */ "PAUSE" /* 0x00B pause from ff */ }; /*************************************************************************** * * TimeDrawCallback() * * This is the channel post draw callback which will draw the * hud on top of the channel which has already been drawn * ***************************************************************************/ #ifdef IRISGL void TimeDrawCallback( vgCommon *chan, void *udata ) { char str[VG_MAXSTRING]; char name[VG_MAXSTRING]; float time; int hour, min, sec; Matrix mv, mp; /* * Convert time (in seconds) into hours, minutes and seconds */ time = vgVCRGetElapsedTime( vcr ); hour = (int) time/3600.0; time -= hour*3600.0; min = (int) time/60.0; time -= min*60.0; sec = (int) time; vgGetName( vcr, name ); sprintf( str, "\"VegaVCR - %s\" Time: %.2d:%.2d:%.2d %s", name, hour, min, sec, states[(int)vgGetProp( vcr, VGVCR_STATE )] ); /* * pushstate: * Save drawing state, so when the callback relinquishes control * of application, application will know how to carry on. */ pfPushState(); pfBasicState ( ); pfDisable( PFEN_TEXTURE ); pfDisable( PFEN_FOG ); pfDisable( PFEN_LIGHTING ); pfTransparency( PFTR_FAST ); zbuffer( FALSE ); /* disable zbuffer */ /* * GL/OpenGL function calls to draw hud */ mmode( MPROJECTION ); getmatrix( mp ); mmode( MVIEWING ); getmatrix( mv ); ortho2 ( 0, 1280, 0, 1024 ); /* make viewing matrix ortho */ mmode( MVIEWING ); pfPushIdentMatrix(); RGBcolor( 255, 0, 0); /* color is red */ vgFontSize( 30, 40 ); /* set char size */ vgFontPos ( kBoxL + 20, kBoxB + 24, 0 ); /* draw string here */ vgDrawFont( str ); /* draw string */ /* * popstate: * restore drawing state basically put it back the way it was */ zbuffer( TRUE ); pfPopMatrix(); /* restore matrix */ pfPopState(); /* restore state */ mmode( MPROJECTION ); loadmatrix( mp ); mmode( MVIEWING ); loadmatrix( mv ); } #else void TimeDrawCallback( vgCommon *chan, void *udata ) { char str[VG_MAXSTRING]; char name[VG_MAXSTRING]; float time; int hour, min, sec; int orgmmode; /* original mat mode */ int zbuf; /* * Convert time (in seconds) into hours, minutes and seconds */ time = vgVCRGetElapsedTime( vcr ); hour = (int) time/3600.0; time -= hour*3600.0; min = (int) time/60.0; time -= min*60.0; sec = (int) time; vgGetName( vcr, name ); sprintf( str, "\"VegaVCR - %s\" Time: %.2d:%.2d:%.2d %s", name, hour, min, sec, states[(int)vgGetProp( vcr, VGVCR_STATE )] ); pfPushState(); pfBasicState(); zbuf = glIsEnabled( GL_DEPTH_TEST ); if ( zbuf ) glDisable( GL_DEPTH_TEST ); glGetIntegerv( GL_MATRIX_MODE, &orgmmode ); glMatrixMode( GL_PROJECTION ); glPushMatrix(); glLoadIdentity(); gluOrtho2D( 0.0, cw - 1.0, 0.0, ch - 1.1 ); glMatrixMode( GL_MODELVIEW ); glPushMatrix(); glLoadIdentity(); glColor3f( 1.0, 0.0, 0.0 ); vgFontSize( 20, 30 ); /* set char size */ vgFontPos ( kBoxL + 20, kBoxB + 24, 0 ); /* draw string here */ vgDrawFont( str ); /* draw string */ /* * popstate: * restore drawing state basically put it back the way it was */ glPopMatrix(); glMatrixMode( GL_PROJECTION ); glPopMatrix(); glMatrixMode( orgmmode ); if ( zbuf ) glEnable( GL_DEPTH_TEST ); pfPopState(); } #endif /*************************************************************************** * * handleKey() * * Assumptions: * * env, chan, gfx are non-NULL * ***************************************************************************/ void handleKey( int key ) { switch ( key ) { case 'D': tod += .01; if ( tod > 1.0 ) tod = 1.0; vgProp( env, VGENV_TOD, tod ); break; case 'd': tod -= .01; if ( tod < 0.0 ) tod = 0.0; vgProp( env, VGENV_TOD, tod ); break; case 'f': toggleProp( gfx, VGGFX_FOG ); break; case 'j': toggleProp( chan, VGCHAN_RENDER ); break; case 'l': toggleProp( gfx, VGGFX_LIGHTING ); break; case 'm': /* Toggle motion model type */ if ( mot ) { long m = (int) vgGetProp( mot, VGMOT_MODEL ); if ( ++m > VGMOT_WARP ) m = VGMOT_SPIN; vgProp( mot, VGMOT_MODEL, (float) m ); } break; case 'P': if ( mot ) { vgPosition *tpos = vgNewPos(); float x, y, z, h, p, r; vgGetPos( (vgCPos *) mot, tpos ); vgGetPosVec( tpos, &x, &y, &z, &h, &p, &r ); printf( "(x,y,z) = (%7.3f,%7.3f,%7.3f)\n", x,y,z ); printf( "(h,p,r) = (%7.3f,%7.3f,%7.3f)\n", h,p,r ); vgDelPos( tpos ); } break; case 's': { unsigned stats = vgGetProp( chan, VGCHAN_STATSSEL ); if ( ++stats > 4 ) stats = 0; vgProp( chan, VGCHAN_STATSSEL, stats ); break; } case 'T': toggleProp( gfx, VGGFX_TRANSPARENCY ); break; case 't': toggleProp( gfx, VGGFX_TEXTURE ); break; case 'u': toggleProp( gfx, VGGFX_BACKFACE ); break; case 'x': /* Toggle motion model state */ if ( mot ) toggleProp( mot, VGCOMMON_ENABLED ); break; case 'w': toggleProp( gfx, VGGFX_WIREFRAME ); break; case 'z': toggleProp( gfx, VGGFX_ZBUFFER ); break; case '?': printf( " D : increase time of day (brightness)\n" ); printf( " d : decrease time of day (brightness)\n" ); printf( " f : toggle fog (on/off)\n" ); printf( " j : toggle channel rendering (on/off)\n" ); printf( " l : toggle graphics state lighting (on/off)\n" ); printf( " m : cycle motion model type\n" ); printf( " P : print current eyepoint location\n" ); printf( " s : cycle statistics\n" ); printf( " T : toggle transparency (on/off)\n" ); printf( " t : toggle texture (on/off)\n" ); printf( " u : toggle backface display (on/off)\n" ); printf( " x : toggle motion model state (on/off)\n" ); printf( " w : toggle wireframe display (on/off)\n" ); printf( " z : toggle Z-Buffer (on/off)\n" ); break; default: break; } } /*************************************************************************** * * toggleProp() * * Toggle property of instance from on -> off -> on * ***************************************************************************/ static void toggleProp( void *handle, int what ) { if ( handle != NULL ) vgProp( handle, what, !((int) vgGetProp( handle, what )) ); } ---------- Post added at 09:59 PM ---------- Previous post was at 09:57 PM ---------- VCR API This is an example of how to create and use Vega VCRs. vcrAPI.c demonstrates a keyboard input only application. This sample also demonstrates VegaVCR's ability to record objects that are dynamically added to and removed from a scene. The blimp object is dynamically added to and removed from the scene. VCR usage is as follows: alt w = rewind alt s = stop alt r = record alt p = play alt f = fast forward alt x = pause Keys 1-9 on top of keyboard enable VCR numbers 1-9 to accept input from the keyboard D = increase time of day d = decrease time of day f = toggle fog on/off j = toggle channel rendering on/off l = toggle lighting on/off m = toggle motion model type P = print current eyepoint location s = show stats T = toggle transparency on/off t = toggle textures on/off u = toggle backfacing x = toggle motion model state on/off w = toggle wireframe on/off z = toggle Z-buffer on/off -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
What is now ? -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
Yes,you can research the software "VR Link of MAK" ,they can do this. This is the addr. http://www.mak.com/products/vrforces.php -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
What is the improvement ? -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
well, VBS2 may used the tech of "HLA,high level architecture",so they can record data of "PDU,Protocol Data Unit". So,zvukoper,you can study the distribute simulation system of Russia army! may be you can find the method. -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
What is improvement ? -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
I support you! Dont give up! Here must be methods! -
what kind of amtosphere model is in the Arma2
rcdxph replied to rcdxph's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Well,thank Carl Gustaffa ,and i am very happy to find the friends who study this kind of problem also. ---------- Post added at 11:43 PM ---------- Previous post was at 11:35 PM ---------- In the virtual battle technology, people can use the MM5 model to simulate the weather, such as Pressure,Temperatures , Moisture saturation level, Air parcel movements due pressures and local effects, Vertical air movement and so on. The kind of technology is called synthetical nature environment(SNE), and is used to the OneSAF,JMASS,STOW and so on. and ,the VBS2 is equal to OneSAF, so i think the Arma2 must be achieve this simulation. -
what kind of amtosphere model is in the Arma2
rcdxph replied to rcdxph's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
no one kown? -
what kind of amtosphere model is in the Arma2
rcdxph posted a topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
what kind of amtosphere model is in the Arma2? Is the mesoscale model MM5 ? -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
it is very mazing ! but,there is one bug that "how to finish the state of Spectating ,then reback the nomal state ? " -
AAR (After Action Review - replay of your battle in Arma2)
rcdxph replied to DZR_Mikhail's topic in ARMA 2 & OA : ADDONS - Configs & Scripting
it would be perfect if the AAR could compatible wiht the "TroopMon2 created by Charon"http://www.armaholic.com/page.php?id=7948, i hope zvukoper could make the compatibility for "the AAR and TroopMoo2" come true !