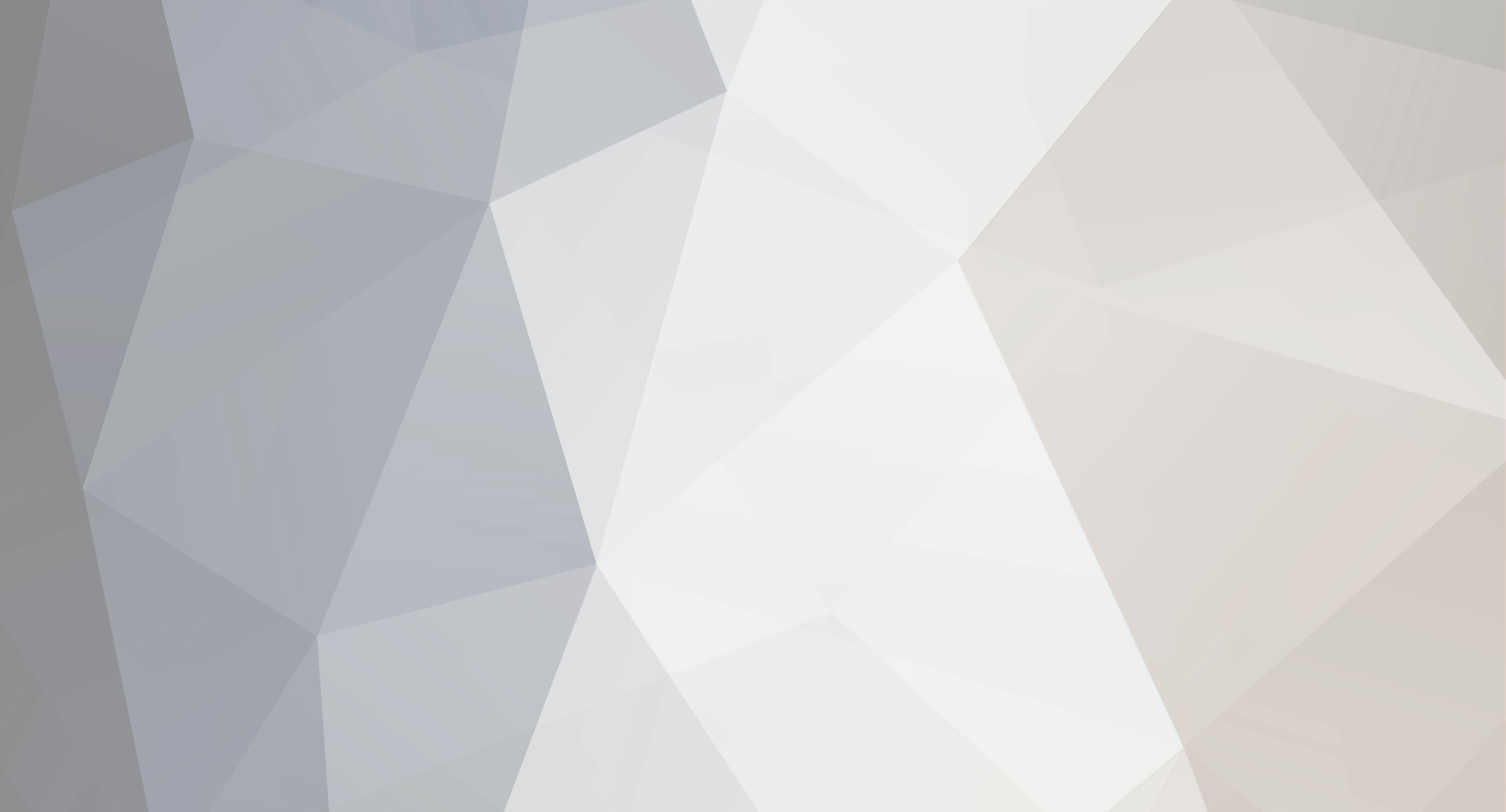

st_dux
Member-
Content Count
876 -
Joined
-
Last visited
-
Medals
Everything posted by st_dux
-
You could also use createDialog instead.
-
First off, you do not need to create a new file just to store an array. That array could be declared anywhere from your init.sqf file to the initialization field of any unit in the editor to the activation field of any trigger with condition "true" (activates automatically at mission start). Since you're already using an init.sqf file, that would probably be the easiest place to store it. All you need to do is add the following line to your init.sqf file: sq1 = [tom,dick,harry]; That's it. Now, after your mission loads, the variable sq1 will return the array [tom,dick,harry]. The question then becomes, "what do you want do with this array?" And I am a little confused about that based on your description, so you'll have to clarify.
-
mission Elapsed time, including 'skipTime' using 'date'
st_dux replied to stilton's topic in ARMA 3 - MISSION EDITING & SCRIPTING
_elapsedDays = (dateToNumber(_curDate) - dateToNumber(_startDate)) * 365; _elapsedHours = (_elapsedDays * 24) mod 24; _elapsedMins = (_elapsedHours * 60) mod 60; _elapsed = format ["%1, %2, %3",floor(_elapsedDays),floor(_elapsedHours),floor(_elapsedMins)]; The "mod" operator (short for modulo) provides a division remainder, which allows you to get remaining hours after elapsed days and remaining minutes after elapsed hours. I assume this is what you really want. -
I sure wish Arma 3 had terrain deformation, mud and raging torrents like SpinTires
st_dux replied to cadmium77's topic in ARMA 3 - GENERAL
Cool video, although I'm not sure all that is really needed in ArmA. The ability to dig holes in the terrain would be a pretty amazing addition, though. I've always felt that digging in is one of the core aspects of real-world infantry combat that ArmA has always more or less ignored. -
There already is a way to get the AI to ignore threats: "CARELESS" behavior. The problem with this is that apart from ignoring threats, "CARELESS" basically emulates "SAFE" behavior, which means that it is always slow and rarely useful. I would love it if "CARELESS" were changed to emulate "AWARE" behavior instead.
- 5179 replies
-
- branch
- development
-
(and 1 more)
Tagged with:
-
getting ai really close to a chair? (ai object avoidance)
st_dux replied to stilton's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Yes, I am. -
getting ai really close to a chair? (ai object avoidance)
st_dux replied to stilton's topic in ARMA 3 - MISSION EDITING & SCRIPTING
You can pass disableAI any string without getting an error, but only certain strings will actually have an effect. PATHPLAN/THREAT_PLAN are VBS-specific parameters that are not recognized in ArmA. If they were recognized, you'd have had no problem getting your AI to walk up to -- or even right through -- your chair. -
mission Elapsed time, including 'skipTime' using 'date'
st_dux replied to stilton's topic in ARMA 3 - MISSION EDITING & SCRIPTING
I would suggest using dateToNumber to get the time difference as a simple scalar value (dateToNumber the current date minus dateToNumber the starting date); then, multiply that value by 365 to get days elapsed. You can figure out hours/minutes/whatever from there pretty easily. -
Because I got tired of the AI moving at a snail's pace simply because they noticed an enemy vehicle that's now a mile a way and no threat at all, I decided to write a function to erase a group's memory of enemy contacts (allowing "COMBAT" behavior to be disabled immediately). This is really a workaround as what it actually does is move all units of a group into a newly created (and enemy contact-free) group, but all of the information (including all waypoints) from the previous group is carried over, so everything set up for the original group in the editor should be in tact. Copy the code below into a file in your mission folder, save it to a variable on mission startup, and follow the instructions in the comments to use. This function has not been tested extensively, but it seems to work just fine in all of the trials I've run it through so far. Let me know if you find any bugs. forget.sqf: /* ---------- dux_fnc_forget by Dux ---------- This function can be used to clear an AI group's memory of enemy contacts, allowing you to immediately reset them to an "AWARE" or "SAFE" state. The function takes advantage of the fact that known enemies are tied to groups and not to units by moving all group members into a newly created group that starts with no known enemies. All of the group's waypoints (including all waypoint statements and synchronization states made in the editor) are carried over along with the group's variable name and group ID; the player should not be able to notice that anything has changed other than the group's behaviour. Note that immediate proximity to enemies will result in the AI quickly re-establishing known enemies, so the function is best used outside of real active combat. Usage: Save this function to a variable via: dux_fnc_forget = compile preprocessFileLineNumbers "forget.sqf"; This function should be spawned. The only argument required is the variable name of the group to forget enemies, sent as a string (NOT the group variable itself). Example call: "myGroup" spawn dux_fnc_forget; Change log: v. 1.0, released 5/5/2015 - initial release v 1.1, released 5/8/2015 - added code to capture group combat mode, formation and speed mode information in case it's not re-established at each waypoint ("no change" selection in editor) This code may be used, shared and modified freely. Please retain use of "dux" variable tag and include original author name when sharing. */ #define SETWP(ARG) (_wp_##ARG select _i) if (typeName _this != "STRING") exitWith {systemChat "dux_fnc_forget requires the group's variable NAME sent as a string (e.g., ""myGroup""), NOT the actual group variable!"}; _varName = _this; _group = call compile _this; _groupID = groupID _group; _leader = leader _group; _side = side _leader; _units = units _group; _waypoints = waypoints _group; // store waypoint info _wp_positions = []; {_wp_positions pushBack (waypointPosition _x)} forEach _waypoints; _wp_types = []; {_wp_types pushBack (waypointType _x)} forEach _waypoints; _wp_behaviours = []; {_wp_behaviours pushBack (waypointBehaviour _x)} forEach _waypoints; _wp_combatModes = []; {_wp_combatModes pushBack (waypointCombatMode _x)} forEach _waypoints; _wp_formations = []; {_wp_formations pushBack (waypointFormation _x)} forEach _waypoints; _wp_speeds = []; {_wp_speeds pushBack (waypointSpeed _x)} forEach _waypoints; _wp_statements = []; {_wp_statements pushBack (waypointStatements _x)} forEach _waypoints; _wp_timeouts = []; {_wp_timeouts pushBack (waypointTimeout _x)} forEach _waypoints; _wp_syncs = []; {_wp_syncs pushBack (synchronizedWaypoints _x)} forEach _waypoints; _wp_triggers = []; {_wp_triggers pushBack (synchronizedTriggers _x)} forEach _waypoints; _wp_current = (currentWaypoint _group) + 1; // +1 here is to account for the extra waypoint that createGroup adds at [0,0,0] // store combat mode, formation, speed mode (behaviour not lost as it is tied to individual units) _combatMode = combatMode _group; _formation = formation _group; _speedMode = speedMode _group; // everyone leaves and joins new group _units joinSilent (createGroup _side); // reset group var name call compile format ["%1 = group %2",_varName,_leader]; _group = call compile _varName; // reset leader, group ID _group selectLeader _leader; _group setGroupID [_groupID]; // reset combat mode, formation, speed mode _group setCombatMode _combatMode; _group setFormation _formation; _group setSpeedMode _speedMode; // reset waypoints for "_i" from 0 to ((count _waypoints) - 1) do { _wp = _group addWaypoint [sETWP(positions),0]; _wp setWaypointType SETWP(types); _wp setWaypointBehaviour SETWP(behaviours); _wp setWaypointCombatMode SETWP(combatModes); _wp setWaypointFormation SETWP(formations); _wp setWaypointSpeed SETWP(speeds); _wp setWaypointStatements SETWP(statements); _wp setWaypointTimeout SETWP(timeouts); _wp synchronizeWaypoint SETWP(syncs); {_x synchronizeTrigger (synchronizedWaypoints _x + [_wp])} forEach SETWP(triggers); }; _group setCurrentWaypoint [_group,_wp_current];
-
[Release] [Function] dux_fnc_forget, a function to make the AI forget its contacts
st_dux replied to st_dux's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Small update: -
Incorrect information posted previously. I'm not sure why your first example isn't working. It looks OK to me. :confused:
-
Distance command without Z
st_dux replied to citazenman's topic in ARMA 3 - MISSION EDITING & SCRIPTING
This would be a problem how exactly? The OP's goal would be achieved just the same. I actually didn't know about that alternate syntax for the select command. Thanks for that, Das Attorney! -
Distance command without Z
st_dux replied to citazenman's topic in ARMA 3 - MISSION EDITING & SCRIPTING
This is misleading. Yes, it's technically slower, but the difference is completely negligible; we're talking about fractions of a millisecond here. Unless performance is really an issue, I always recommend simplicity. -
Disembarked units getting stuck
st_dux replied to L3TUC3's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Get rid of the "GET OUT" waypoint for the squad, and it should work. -
Distance command without Z
st_dux replied to citazenman's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Alternatively, you could use the function that BIS made for this already: waitUntil {([_c130pilot,player] call BIS_fnc_distance2D) < 350}; -
https://community.bistudio.com/wiki/setAmmo
-
@NeMeSiS: I tested it, and it seems that disableAI "FSM" has no effect on the automatic switch to "COMBAT" behavior as I had feared :(. I'm still not really sure what it's supposed to do. On the bright side, seba1976's trick can be utilized in a workaround that effectively reset's a group's memory instantly, causing "area clear"-type behavior until the group runs across another enemy: fnc_forgetEnemies = { _varName = _this; _group = call compile _this; _leader = leader _group; _units = units _group; _side = side _leader; _units joinSilent (createGroup _side); call compile format ["%1 = group %2;%1 selectLeader %2",_varName,_leader]; }; The above can be called for any group by passing the group's variable name as a string (e.g., "groupBravo" for a group assigned to variable groupBravo), and the result will be that that the group forgets all enemies immediately while retaining the same leader and group variable. However, you will lose all waypoints, which is pretty terrible. You could use waypoint scripting commands to recreate them all, but the hacky-ness level begins to approach 9,000 at this point. Still, this is probably our best bet for now, unfortunately. By "mentioned scripting command," I assume you're referring to disableAI "FSM"; however, my testing has shown me that this doesn't actually stop the automatic switch to "COMBAT" behavior that occurs when a group knows about any enemies. This implies one of two things: Either disableAI "FSM" doesn't actually disable all of the active FSMs, or it does but there is something else going on in the background that causes the automatic behavior mode switch when enemies are detected. Do you know which of these is the case? This is very exciting! Thank you for the update. I agree that the AI should value its life by default, but I don't believe this precludes the inclusion of a "force-move" option for mission designers. There are many instances where such a function, when applied carefully, would result in realistic and immersive AI combat maneuvers that simply are not possible to create while the "COMBAT" behavior AI is active. Also, the ability to override the AI's life-preserving default behavior has been available to mission editors in the form of "CARELESS" mode since OFP, but for whatever reason this mode also forces very slow, "SAFE"-style movement that 9 times out of 10 will render the mode useless. If "CARELESS" emulated "AWARE" behavior without concern for enemy presence instead of "SAFE" behavior without concern for enemy presence as it does now, it would be perfect.
- 5179 replies
-
- branch
- development
-
(and 1 more)
Tagged with:
-
That's very interesting information. I can see making a workaround using this, but it might be messy. This would only work to bring about a "clear" state faster, though; I can't see it really doing anything for movement under fire. Is this really true? I will have to test it more. I remember trying disableAI "FSM" and finding that it did nothing to prevent automatic "COMBAT" behavior, but perhaps I wasn't using it right. Now I have something to test. Thanks guys!
- 5179 replies
-
- branch
- development
-
(and 1 more)
Tagged with:
-
I really don't understand why this request is continuously ignored. Honestly, how difficult could it be to make the AI ignore automatic danger behavior? There is no need to add anything at all beyond a simple switch to do what the engine does automatically anyway. There are several ways this could be done: As mentioned earlier, introduce a new behavior mode that would be identical to "AWARE" except that it would override the automatic switch to "COMBAT" like "CARELESS" and "STEALTH" modes already do. Like "CARELESS", this mode would probably make the most sense as a script-only mode. Introduce a new scripting command that forces the "Area Clear" AI mentality. This would essentially suppress all reaction to knowledge of enemy presence until disabled. Modify the effect of disableAI "FSM" so that it essentially produces the same "knowledge suppression" effect. (Side note: disableAI "FSM" currently seems totally pointless). I'm sure there are other possible methods, too. This can't be that difficult. Can it?
- 5179 replies
-
- branch
- development
-
(and 1 more)
Tagged with:
-
Problem with while do loop
st_dux replied to spitfire007's topic in ARMA 3 - MISSION EDITING & SCRIPTING
_selectedBuilding is showing up as "any" because you haven't declared it outside of the while loop's scope; thus, the variable is meaningless outside of the loop. Put "_selectedBuilding = objNull;" right below your first line (before the while loop) to solve this problem. See also: http://community.bistudio.com/wiki/Variables#Scope -
@oukej: Is there a plan to give mission editors/scripters the ability to override automatic combat behavior in the AI? I realize that the AI switching to combat behavior automatically when "feeling threatened" goes back to OFP, but combat behavior has changed a lot since then, and right now the situation is such that combat behavior is essentially non-responsive behavior. It makes it practically impossible to script something as simple as getting an infantry unit to go directly from point A to point B while in enemy territory. As a mission designer, this is extremely frustrating. "Stealth" and "Careless" modes already override the automatic switch to combat behavior. Unfortunately, "Careless" results in forced "Safe" behavior, which is rarely useful, and "Stealth" is pretty much just "Combat" mode with whisper sounds instead of yelling ones. Why can't we have access to an "Aware" (or "Aware"-like) mode that also overrides the automatic switch to combat behavior? This one little thing would allow for so much more control over the AI.
- 5179 replies
-
- branch
- development
-
(and 1 more)
Tagged with:
-
In the init field of each FIA group leader: this call fnc_makeOPFOR fnc_makeOPFOR: { _units = units (group _this); _newGroup = createGroup east; _units join _newGroup; }; Note that for this to work the OPFOR side will need to have been initialized (easily done by placing a single OPFOR unit anywhere in the editor).
-
This functionality has been requested since time immemorial but has never been granted. I have no idea why; it would make scripting effective AI assaults actually feasible.
- 22 replies
-
Retain leadership of AI in multiplayer co-op using team switch
st_dux replied to jassida's topic in ARMA 3 - MISSION EDITING & SCRIPTING
I didn't know about the onTeamSwitch command. I would definitely try that first! -
Retain leadership of AI in multiplayer co-op using team switch
st_dux replied to jassida's topic in ARMA 3 - MISSION EDITING & SCRIPTING
There's rarely ever a single best way to do something, but the script approach would be simple enough. When called, the following little script will make the unit at which you are pointing the group leader and then give you control of that unit, provided that you are pointing at a unit in your group. _target = cursorTarget player; _group = group player; _playerVarName = vehicleVarName player; if (isNil _target) exitWith {hint "No target in sight!"}; if (!_target in (units _group)) exitWith {hint "Target is not part of your group!"}; _group selectLeader _target; selectPlayer _target; _target setVehicleVarName _playerVarName; hint "You have occupied a new body!"; This script should only be called locally on your machine during a mission. Your original unit needs to be named in the editor for the vehicleVarName stuff to work.