Sign in to follow this
Followers
0
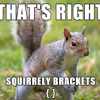
How to "find" subarray by element of that subarray?
By
Heeeere's johnny!, in ARMA 3 - MISSION EDITING & SCRIPTING
By
Heeeere's johnny!, in ARMA 3 - MISSION EDITING & SCRIPTING