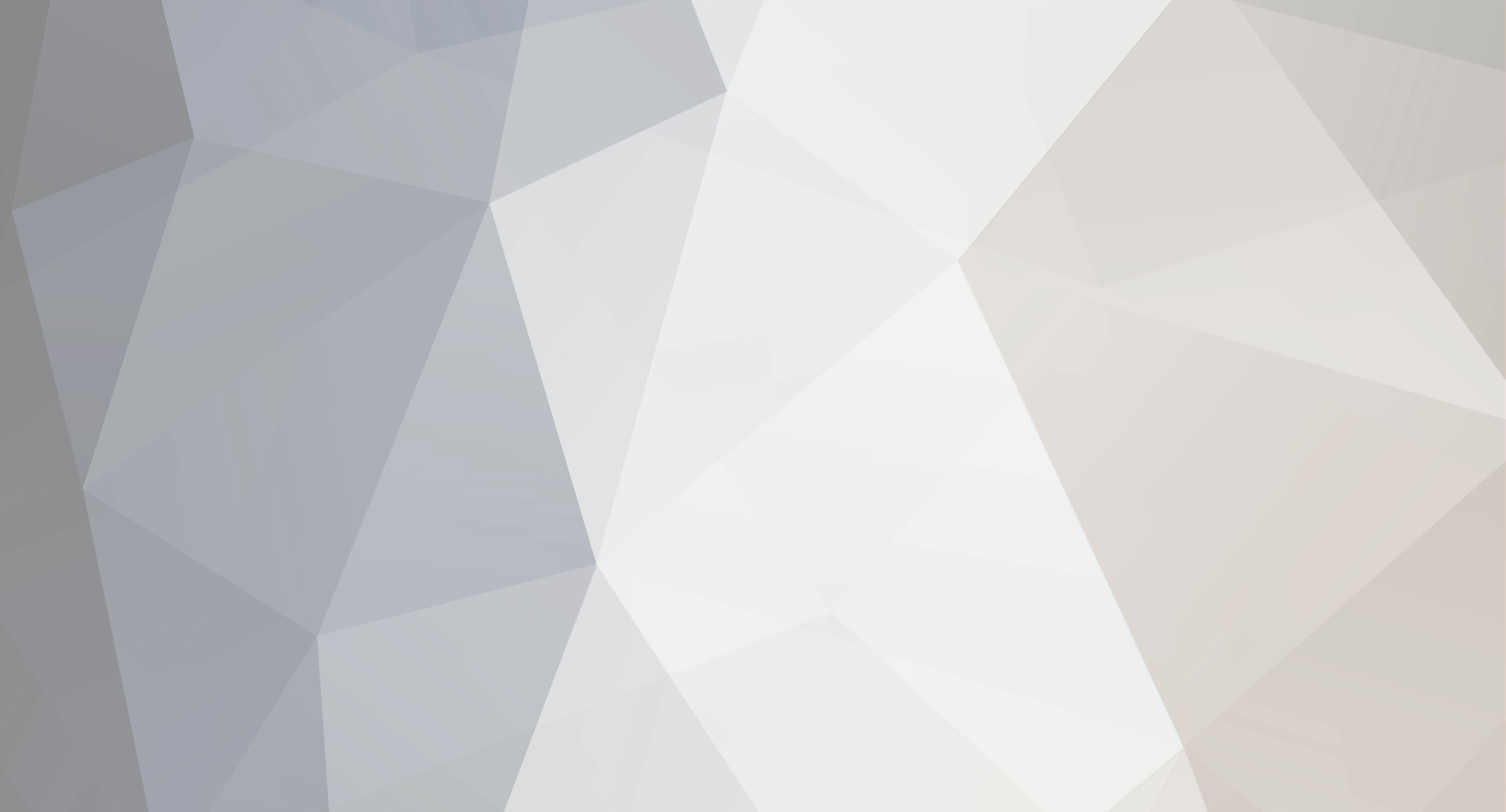

Mattar_Tharkari
-
Content Count
961 -
Joined
-
Last visited
-
Medals
Posts posted by Mattar_Tharkari
-
-
Most helicopters will turn on landing, a) to dump speed. b) to turn into the wind. Thats the main problem for me - the AI just yank back on the stick like a noob flyer and climb to lose speed - it's awful! Scripting a turn and setting the wind is easy - it's getting them to miss terrain features while doing it that's hard, probably why BIS have left it the way it is?
-
True it can be frustrating - problem is you really need to read the wiki as some waypoints don't act the way you expect. Helicopters are also affected by the wind - hence the odd landing backwards behaviour. The AI are programmed to land into the wind but have no knowledge of turning into the wind to land, so I find I have to set the headwind for them in order to guarantee a realistic landing. So yes it's frustrating as the setWind command is a headache to understand.
-
Syntax is a little off - try this, addwaypoint returns an array (format waypoint) with [group,index] so you don't need to add it again:
_fitwp1 = fitter addwaypoint [position fitterwp1,0]; _fitwp1 setWaypointType "MOVE"; _fitwp2 = fitter addwaypoint [position fitterwp2,1]; _fitwp2 setWaypointType "CYCLE";
Your basic problem is not enough waypoints - they will not fly to the CYCLE WP - it simply instructs them to return to the closest / initial WP.
In your case they will fly from WP-0 (created when bis_fnc_spawnvehicle spawns vehicles) to WP-1 (_fitwp1) - then CYCLE back to WP-0 (or WP-1 if it's closer).
Just tried it in the editor, Takistan - put a group of 2 jets on the map, flying, near southern airfield, with AI as leader, player in rear jet - put a WP on the south airfield type MOVE, put another WP on the north airfield type CYCLE. The AI will circle the 2 waypints at the bottom of the map and never fly near the CYCLE waypoint. You need to add another MOVE type up north to get them to do the correct thing.
What are fitterwp1, fitterwp2, fitterspawn? Objects?
-
Better version of the above that targets nearest enemy (dependant on if they know about the enemy), I suppose you could add to this and change the velocity of the grenade depending on the distance to the enemy. However getting them to throw it as far away as possible is probably a good standard option - grenades are dangerous things in Arma2 lol:
for init.sqf
{if (side _x == EAST && !(isPlayer _x)) then { _x addEventHandler ["firedNear", " _nrstNME = (_this select 0) findNearestEnemy (_this select 0); _frnd = side (_this select 0); _val = floor(random 11); if (!(isNull _nrstNME) && _nrstNME distance (_this select 0) >= 40 && _nrstNME distance (_this select 0) <= 60 && _val == 1 && !(_frnd == side (_this select 1))) then { _dir = ((getPosATL _nrstNME select 0) - (getPosATL (_this select 0) select 0)) atan2 ((getPosATL _nrstNME select 1) - (getPosATL (_this select 0) select 1)); _dir = _dir % 360; (group (_this select 0)) setFormDir _dir; (_this select 0) setDir _dir; (_this select 0) fire ['HandGrenadeMuzzle', 'HandGrenadeMuzzle', 'HandGrenade_West']; hint format['Shot detected 40m-60m from %1',name (_this select 0)];}; "]; }; } forEach allUnits;
example mission:
https://dl.dropbox.com/u/37698503/scriptdThrwFNE.Shapur_BAF.zip
-
inv = [bYBox] call BIS_fnc_invstring;
BYBox need to be a player, AI or driveable vehicle - that function doesn't work on objects or ammo boxes;
To add the magazines to a HMWWV put this in it's init (eg three Laserbatteries, change the mag name to whatever):
this addMagazineCargoGlobal ["Laserbatteries",3];
-
Made something light and quick but not perfect, the grenades are usually thrown in the right direction but may bounce back off obstacles:
//==================================================================================================================== // Example mission by // Mattar Tharkari // Make AI throw grenades //==================================================================================================================== // The next event handler adds the grenade throwing action // at the correct range 40-60m // in the correct direction // triggers if the AI is fired on // _val is the chance of a throw occuring, 1 in 11 in this case; // change (side _x == ****) to EAST or WEST // remove the hint - it's only to show detection frequency // will only work if AI have grenades - you need to equip them if not. //==================================================================================================================== {if (side _x == EAST && !(isPlayer _x)) then { _x addEventHandler ["firedNear", " _dir = ((getPosATL (_this select 1) select 0) - (getPosATL (_this select 0) select 0)) atan2 ((getPosATL (_this select 1) select 1) - (getPosATL (_this select 0) select 1)); _dir = _dir % 360; _val = floor(random 11); _frnd = side (_this select 0); if (_this select 2 >= 40 && _this select 2 <= 60 && _val == 1 && !(_frnd == side (_this select 1))) then { (group (_this select 0)) setFormDir _dir; (_this select 0) setDir _dir; (_this select 0) fire ['HandGrenadeMuzzle', 'HandGrenadeMuzzle', 'HandGrenade_West']; hint format['Shot detected 40m-60m from %1',name (_this select 0)];}; "]; }; } forEach allUnits;
Example Mission:
https://dl.dropbox.com/u/37698503/scriptedGnadeThrow.Shapur_BAF.zip
Bullets are disabled in the mission, although there is lots of shooting, it's only the grenades doing the killing
-
Info is all there in the wiki Avi - the guard waypoint needs a guard trigger type to work, it's possible you haven't set it up properly:
http://community.bistudio.com/wiki/Waypoint:Guard
-
It's already answered:
_pos = [(getPosATL player select 0) + (1000 * sin(360)), (getPosATL player select 1) + (1000 * cos(360)), 0]; //position 1000m north of player wp1 = Tank1 addwaypoint [_pos, 0];//place WP at the above position wp1 setwaypointtype "SAD"; Wp1 setWaypointCompletionRadius 100;
-
It's working perfectly - there are no "LaserBatteries" in _mags, however if you check for "Laserbatteries" you will find you have them!
_mags = magazines player; hint format ["%1",_mags]; if ("Laserbatteries" in _mags) exitWith { player sidechat "You have a battery"; }; if !("Laserbatteries" in _mags) exitWith { player sidechat "You do not have a battery"; };
If you have problems with checking an array - hint it - it will show you exactly whats in it. Strings are case sensitive.
-
Locality issue - the player is on the client - all the other stuff is running on the server
setPos is global - untested but this should work in there?
{_x setPos (getMarkerPos "Base")} forEach playableUnits;
(getMarkerPos is local but may work with the CBA function? if not setMarkerPos the marker at it's current location 1st?)
some other stuff that may help here:
-
It's the 200 in that script line - that's the distance nearObjects checks for street lights.
You don't actually need to use a game logic there, eg:
{_x switchLight "OFF"} forEach ((getPosATL player) nearObjects ["StreetLamp",200]);
That in a script will turn off light's within 200m based on the position of the player - substitute player for any object, vehicle, unit, marker or position:
{_x switchLight "OFF"} forEach ((getMarkerPos "lightsOff") nearObjects ["StreetLamp",200]);
-
ROFL @ video soundtrack :rolleyes: nicework but maybe the Geiger Counter would be better with a beep instead of a buzz to avoid those unintended comedy puns? (If you don't know what I mean, I'm not going to explain it to you!)
Not sure but can't see where you created the effect?
http://community.bistudio.com/wiki/ppEffectCreate
Also PP stands for post processing - if you have it switched off in the game video options, bluring and filmGrain will never work. To make sure everyone gets an effect regardless of video settings - maybe a high frequency camShake would be better? If you search for "camShake" in the scripting wiki you will find all the commands you need.
-
It's terribly mixed up - you are not passing it the correct info when spawning the new script;
[m] spawn town_object;
here you are passing a marker reference string and then using it as a poistion?:
_positions = _this select 0; you should replace m with _pos for example?
_town setMarkerColor west_near;
This will not work because _town is an object, m is the marker?
You can't check the distance between something and a marker directly as the distance command doesn't work with markers, possibly why you are using the H - BUT you can use the markers position:
if (_x distance (getMarkerPos "m") < 200) then {};
-
Well it's just an example, you can build an array of positions, objects, vehicles, markers etc and set the waypoints however you like. If you set the waypoint to "SAD" they will in fact search and destroy - why not just have a "move" waypoint synchronised to a trigger switch - activation: opfor, not present? The commands are in the wiki to script all that to make it dynamic. Creating 8 waypoints all on the player's position is a little strange?
-
No delays or sleeps in your init.sqf is there? Usually this happens when there is something that delays the start of the script - could be script errors somewhere too? got -showscripterrors on?
-
You can condense much of that, tested and working:
waitUntil {!isNil "bis_fnc_init"};//you need a functions module on the map _GroupTank = CreateGroup West; //you need marker "tankspawn" on map with a 100m clear radius for the tanks to spawn: {[[(getMarkerPos "tankspawn" select 0) + (random 50 * sin(random 360)), (getMarkerPos "tankspawn" select 1) + (random 50 * cos(random 360)), 0], 360, "M1A2_US_TUSK_MG_EP1", _GroupTank] call bis_fnc_spawnvehicle; sleep 0.5;} forEach [1,2,3,4,5,6,7]; {_x setDamage 0} forEach units _GroupTank; _mkrlst = ["wp1mkr","wp2mkr","wp3mkr","wp4mkr","wp5mkr","wp6mkr","wp7mkr","wp8mkr"]; //place markers with these names on the map where you want the waypoints to be {_wpt = _GroupTank addwaypoint [getMarkerPos _x, 0]; _wpt setwaypointtype "MOVE"; _wpt setWaypointSpeed "FULL"; _wpt setWaypointCompletionRadius 10; } forEach _mkrlst; //this moves and changes the type for waypoint 2, 1000m north of the player [_GroupTank, 2] setWaypointPosition [[(getPosATL player select 0) + (1000 * sin(360)), (getPosATL player select 1) + (1000 * cos(360)), 0], 0]; [_GroupTank, 2] setWaypointType "SAD"; //They will spend a long time searching - if you need them to move on change the waypoint back to "move" when the task is completed. //debug _wPos = waypointPosition [_GroupTank, 2]; _mkr0 = createMarker["mkrtest",_wPos]; _mkr0 setMarkerShape "ICON"; _mkr0 setMarkerType "dot"; _mkr0 setMarkerColor "colorGreen"; _mkr0 setMarkerText "New WP2 location"; //To delete all the tanks and crews at WP8: [_GroupTank, 8] setWaypointStatements ["true", "{deleteVehicle (vehicle _x)} foreach units (group this); {deletevehicle _x} foreach units (group this);"];
-
Okay by principle it works very well. However, it seems that the shell explodes directly after the round was shot, killing the gunner. :(Really? Works for me? Where did you put the event handler?
-
http://community.bistudio.com/wiki/setSimpleTaskDestination
tskobj_1 setSimpleTaskDestination (getMarkerPos "mkrName");
-
No it's random - if you want a position 1000m north you have to generate it first:
_dir = 360; _dist = 1000; _ref = getPosATL player; _pos = [(_ref select 0) + (_dist * sin(_dir)), (_ref select 1) + (_dist * cos(_dir)), 0]; _wp2 = _group addWaypoint [_pos, 0, 2]; [_group, 2] setWaypointType "MOVE";
_ref can be any marker,object or position eg, getMarkerPos "mkr"; getPosATL _vehicle; [0,0,0];
waypoint index is 2 in the above - adjust to whatever.
-
You need the command: str , it converts any variable to a string:
eg to check the array on modelToWorld:
_worldPos = [6,6,6]; _x = 6;_y = 6;_z = 6; if (format ["%1",_worldPos] == str[_x,_y,_z]) then {hint "location Ozzy Osbourne found";};
str[_x,_y,_z] == "[6,6,6]"
format ["%1",_worldPos] == "[6,6,6]"
Do you want the whole array as 1 string (as above) or each element converted to a string? as in:
[1,2,3] to ["1","2","3"]
nul = [] execVM "stringArrayCnv.sqf";
_array1 = [1,2,3]; _array2 = []; for "_i" from 0 to (count _array1 - 1) do { _array2 set [_i, str(_array1 select _i)]; }; player sidechat format ["%1",_array2];
-
^ Just been working on an insurgent rocket attack script (rocket fired low trajectory from launch to target with guidance) using the vector function by Venori - @ZK you want me to send it to u to finish off lol? I wan't to put in a random firing composition based on this:
Og7KRzBY4hs
It will probably end up as a small mission to track down the firing team.
-
modelToWorld does work? If you want the marker always to the right of the vehicle, no matter what vehicle direction :)
"markerName" SetMarkerPos (vehicleName ModelToWorld [5,0,0]);
Try that in a radio trigger, BIS_fnc_relPos is the other good option (was going to suggest it last night until I found MTW worked! which was news to me), you sure you have a visible marker on the map?
-
_string = ""; if (isNil _string) then {player sidechat "nil string"}; if (_string=="") then {player sidechat "emptyQuotes string"};
-
To delete the projectile:
_mortar addEventHandler ["fired", {deleteVehicle (_this select 6);}];
If you want the AI to fire:
_mortar action ["useWeapon", _mortar, (gunner _mortar),0];
nul = [_mortarName, 3] execVM "mortar.sqf";
_mortar = _this select 0; _rounds = _this select 1; for "_i" from 0 to (_rounds-1) do { if (count (magazines _mortar) <= 2) then {{_mortar addMagazine "8Rnd_81mmHE_M252"} forEach [1,2,3]; hint "mortar reloaded";}; _mortar action ["useWeapon", _mortar, (gunner _mortar),0]; sleep 6; };
Dialog Tutorial For Noobs [By A Noob]
in ARMA 2 & OA : MISSIONS - Editing & Scripting
Posted
Nice work! Thank you!