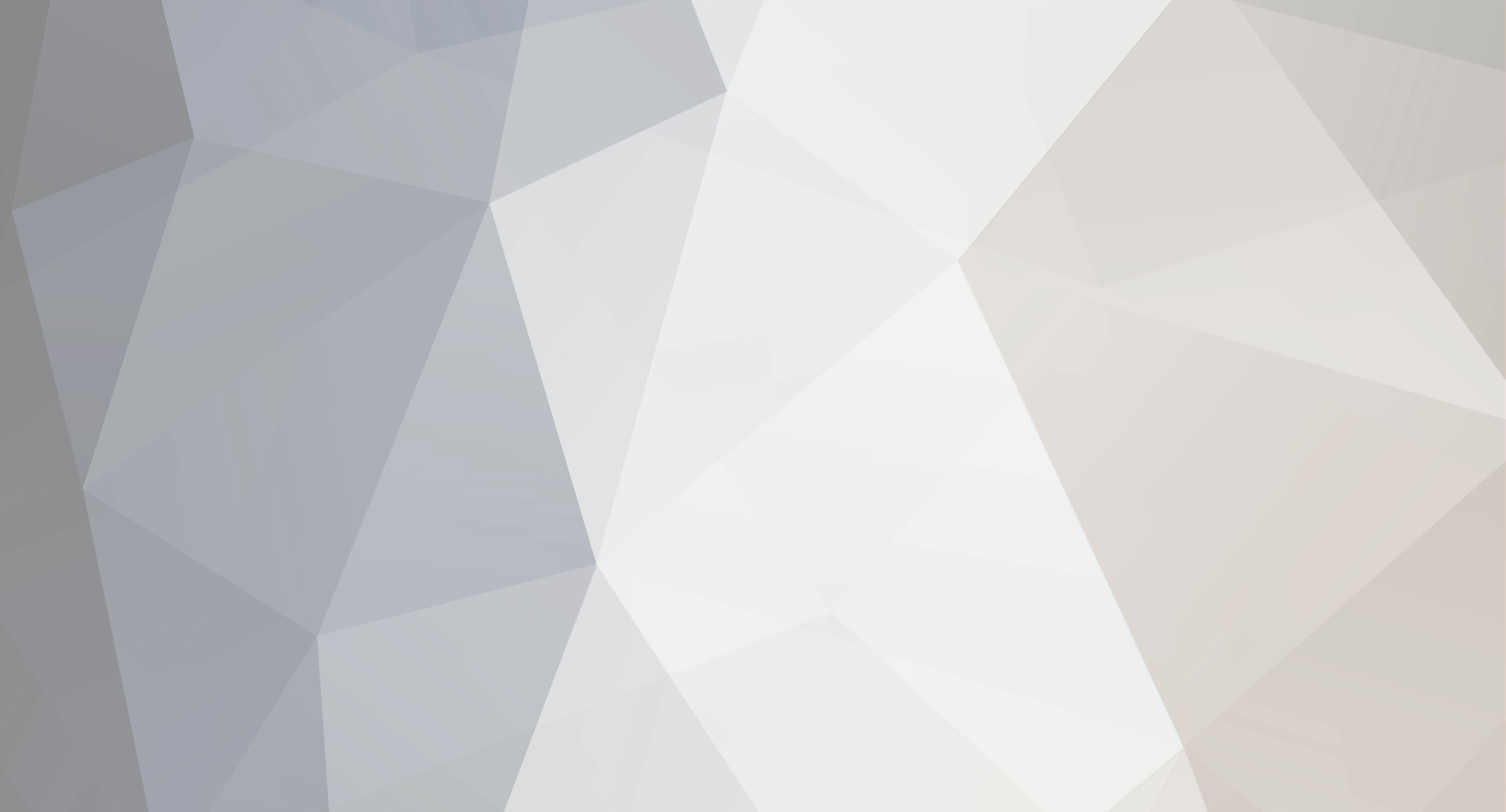

xxanimusxx
Member-
Content Count
453 -
Joined
-
Last visited
-
Medals
-
Medals
-
Everything posted by xxanimusxx
-
Position of newly killed man/animal?
xxanimusxx replied to Tankbuster's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
I guess you are using createVehicle instead of createVehicle_array - use the latter one, its MUCH more quicker and you can disable collision checking, which is your problem it seems :D -
Help with hide body
xxanimusxx replied to malcon's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Well there are two ways to do this: Add an Killed-Eventhandler to every unit and once the handler is fired, add an action to the unit who died. Check if the player's cursor is pointing to a dead unit and add an action to the player The latter option is slightly more complex but has the advantage that dynamically spawned units won't have to be configured with an eventhandler. I'd suggest the first option though because it's easier to implement even for newbies :D The problem is not adding the action, but the "animation" to play when you want to hide a body. There's this features in DayZ and when you hide a body, the body sinks into the ground until it's away (?!). So you have to first decide what the body should do upon hiding - the rest is easy :D -
How to remove all weapons of player, when he steps into trigger?
xxanimusxx replied to frezinator's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
*cough* removeAllWeapons -
How to move respawn_west in trigger, when player steps in that trigger???
xxanimusxx replied to frezinator's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Well "respawn_west" is a marker, isn't it? If so, it's quite easy :D Name your trigger (for my example I'm going to use "myTrigger") and insert this into the activation field: "respawn_west" setMarkerPos (getPosATL myTrigger); -
How to add more GBU and Sidewinders missiles to F-35B jet plane???
xxanimusxx replied to frezinator's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Well you run it for example in the init-field of your F35B, you just have to replace "yourVehicle" with "this" and voila :D -
How to count AI soldiers kills?
xxanimusxx replied to lato1982's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
the callback used in the killed eventhandler will give you an array with the killer and the killed unit as a parameter. friendlyAIUnits = units (group player); killedEH = { _killed = _this select 0; _killer = _this select 1; _numKills = (_killer getVariable ["killsNum", 0]) + 1; _killer setVariable ["killsNum", _numKills, true]; if (_killer in friendlyAIUnits) then { // the killer is one of your AI members, do whatever you feel fancy here // preferably calling another function to add skill points or whatever. }; }; { if (!(isPlayer _x) && !(_x in friendlyAIUnits) ) then { _x addEventHandler ["killed", killedEH]; }; } forEach allUnits; using the above code will ensure that no matter who kills AI players, they'll get their kill counter increased. So using something like _numKilly = theUnit getVariable ["killsNum", 0]; on any AI or human unit in your game will get the corresponding kill counter. -
Editing Questions
xxanimusxx replied to anthropoid's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
The file formats of the missions saved when in 3D and 2D mode are different so you can't open them in both, you have to stick to one of them. There are, however, tools to convert 2D/3D missions somewhere if you really need them. Holding the ALT-Key on your keyboard while moving your mouse will change the Z-Value of the object you've selected. If that doesn't work, you can set the Z-Value in the init-field of the object using: "relPos = getPosATL this; rePos set [2, HEIGHT]; this setPosATL relPos;" There are several topics concerning placement in buildings in this forum which got tons of replies so I guess you've to search a little or wait for another user to remember the links :> -
unlock door/computer
xxanimusxx replied to RecondoLRRP's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Put all your guard's references into an array and randomly pick out one of them to hold the key. myGuards = [myGuard1, myGuard2, myGuard3 // ....]; // ... _guardHoldingKey = myGuards select ( floor( random(count myGuards) ) ); _guardHoldingKey setVariable ["holdingKey", true]; Now add actions to the guards (for example when they're dead) like "Search for key" and when the action is picked, look if the above variable is set. // In the SQF-file which is called upon picking the action attached to the guards _target = _this select 0; _caller = _this select 1; _target removeAction (_this select 2); _keyFound = _target getVariable ["holdingKey", false]; _target setVariable ["holdingKey", nil]; player setVariable ["keyFound", _keyFound]; if (_keyFound) then { hint "Got the key!"; } else { hint "He didn't have the key"; }; Now you'd need to slightly alter the addAction in the laptop's init-field: this addAction ["Hack Laptop", "hacking.sqf", nil, 6, false, true, "", "(_target distance _this < 2) && (_this getVariable ['keyFound', false])"]; Here's a list with script examples which should contain one or more script fitting your scenario. If you want to have an object act as a key (or a real key, I think there was one as an evidence type of object) you'd put it into the gear of the guard which is randomly picked and check for it in the condition-area of the addAction. The commands you'd use for that are: addMagazine, magazines -
How to add more GBU and Sidewinders missiles to F-35B jet plane???
xxanimusxx replied to frezinator's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
yourVehicle addEventHandler ["killed", { _vehicle = (_this select 0); // Spawn a new one on the exact same spot _newVehicle = (typeOf _vehicle) createVehicle (getPosATL _vehicle); // Delete the old one deleteVehicle _vehicle; _newVehicle addMagazine "2Rnd_GBU12"; // ..... }]; This is one way you could achieve this, but it's not that practical because the vehicle spawns instantly after the other one got "killed", so you have to think of an appropriate way of respawning your vehicle. -
How to add more GBU and Sidewinders missiles to F-35B jet plane???
xxanimusxx replied to frezinator's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Well if your jet is respawned, you have to re-supply it using the code lines I posted above - if you don't do it, your jet will have the default amount of ammo. -
[Help] Issues with values.
xxanimusxx replied to Col. Ben Sherman's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Scalar is the result of mathematical calculations - mostly returned when devided by zero or when other strange calculations are made. Never got that result in valid calculations though xD -
[Help] Issues with values.
xxanimusxx replied to Col. Ben Sherman's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Well if "Value" is used in more than one sqf-file, maybe it get's altered before you show it on your dialog. You could test for this occurance naming your variable "Value" to something unique, like "iWantToTestThis = _myValue"; and try to output that in your dialog. If this works, you know the cause of your problem and have to search every of your files in which "Value" is used. -
How to add more GBU and Sidewinders missiles to F-35B jet plane???
xxanimusxx replied to frezinator's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
You can use addMagazine to, yeah, add magazines to units or vehicles, even if they don't have enough slots left for it. To add 2 more GBU's to the F35B: yourVehicle addMagazine "2Rnd_GBU12"; And to add 2 more sidewinders: yourVehicle addMagazine "2Rnd_Sidewinder_F35"; If you want much more of em, do it in a loop :D -
[Help] Issues with values.
xxanimusxx replied to Col. Ben Sherman's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
So how is "kontostand" associated with "Value"? Or is this just an example? Does it work if you forward-space your variable ("kontostand", "Value" or whatever) with a "str" ? Something like lbAdd [1, format ["Value: %2", localize "STRS_statdialog_value", str kontostand]]; (it's weird to use "str" in a format but as we are grasping at straws here we have to try everything out) -
[Help] Issues with values.
xxanimusxx replied to Col. Ben Sherman's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
So how do you actually hint the value? Do you use "hint Value" or "hint str Value" ? -
unlock door/computer
xxanimusxx replied to RecondoLRRP's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
One scenario could be achieved with the following steps: Add an action to the object you want to interact with (door/pc or whatever) When the action is picked, the associated script is run Now start a timer in the script - you should do this using a spawn so the timer will decrease independently Start a while-loop in which you check the cursorTarget and compare it to the object the player is looking If the cursorTarget is not for example the door, stop the timer and display an appropriate message and re-add the action to the object When the timer is expired and the player looked at the object the whole time, stop the while-loop and do whatever you want to do at this point The only problem using cursorTarget is: it can be a bitch sometimes :D Looking at small objects like a laptop will be slightly okay if your character doesn't move and looks straight to the object, but looking at objects which are stacked together with just a small gap between them will cause the cursorTarget-command to return another object even if you just as move your character for an inch. Another way to do this would be using "eyePos" and add a tolerance area but yeah, this is overkill imho. // edit: here's an example script, didn't test it so could be buggy. create a script named "hacking.sqf" and paste this code in there: _refObj = _this select 0; _player = _this select 1; _actID = _this select 2; // Remove the action so you can't hack twice! _refObj removeAction _actID; playerIsHacking = true; hackTime = 10; // in seconds maxBars = 20; char = "#"; _timer = { _bars = ""; _quotient = 0; for "_time" from hackTime to 1 step -1 do { _quotient = ceil((_time/hackTime) * maxBars); _bars = ""; for "_i" from 1 to _quotient do { _bars = _bars + char; }; for "_i" from _quotient+1 to maxBars do { _bars = _bars + " "; }; hintsilent format["Hacking in progress:\n[%1]", _bars]; sleep 1; }; playerIsHacking = false; }; _timerHandle = [] spawn _timer; waitUntil {!playerIsHacking || cursorTarget != _refObj}; hintsilent ""; // So why did we get here? if (playerIsHacking) then { // The player did look away! You're a bad, bad boy! terminate _timerHandle; hint "Hacking aborted!"; // Re-Enable action (optional); _refObj addAction ["Hack Laptop", "hacking.sqf", nil, 6, false, true, "", "_target distance _this < 2"]; } else { // WE DID IIIIIT! hint "Hacking successful - hacked into FBI database"; // Now do what you want here, preferably execVM another script. }; playerIsHacking = false; Place an object in the editor (I took a laptop) and put this into the init-field: this addAction ["Hack Laptop", "hacking.sqf", nil, 6, false, true, "", "_target distance _this < 2"]; -
[HELP]Remote shoot unmanned Static turret [MP]
xxanimusxx replied to OMGJannik's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
The command "fire" needs a unit to work imho. The description of the command is: "Forces a unit to fire the given weapon." I was trying to do this as well some time ago and the only other option I saw fit was spawning an invisible AI into the turret and use AI-commands to let him fire the turret. Well, I didn't have much success so don't ask me how I did it xDD -
cursorTarget for multiplayer?
xxanimusxx replied to KilEKarl's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
How do you define "works"? cursorTarget always returns the object you're pointing at, regardless of single or multiplayer. You have to tell us more what you want to achieve with this command, maybe there's some other multiplayer localness problem in your code :D -
[Help] Issues with values.
xxanimusxx replied to Col. Ben Sherman's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
I'd look what _dbquery returns using a hint: hint str _dbquery; This way you can make sure that no malformed data is returned, thus leading to "Any" upon compiling the returned string. You should consider looking into _dbvalue as well using the above hint to check if the array your working with is being created correctly. -
crB_UnitCaching
xxanimusxx replied to Blitzer134's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
//diag_log format["MSO-%1 CEP Caching # %2", time, _str]; //hint format["MSO-%1 CEP Caching # %2", time, _str]; This will do the trick. -
crB_UnitCaching
xxanimusxx replied to Blitzer134's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Hm, don't be offended mate but I don't think anyone would be motivated to help you out with you just providing one sentence to your problem. How about telling us more about the scripts/mods you refer to in more detail before making a request? Doing so will help everyone (including me) who don't know anything about your topic understanding more and will eventually lead into solving your problem. Without knowing anything about "crB_UnitCaching" I'd say just look for the hint/hintsilent-command in the script files which contains the specific text which is showing on your screen. Once you found out the scriptline, just delete or comment it for it to disappear. Every "advanced" text editing program like Notepad++ has a function to search recursively in folders so this would be an easy task I'd say :> -
[Release] AniRadar - customizable radar for your needs
xxanimusxx replied to xxanimusxx's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
That's really a good idea Hand of MoscoW, normally you'd even get depth information using a real sonar so why not? It's really simple to get the altitude and the speed of the objects, but as I'm using native GUI-elements to show the images on the radar screen, it will create more overhead in the description.ext. This would be pretty easy to achieve once I switch to drawing an interactive map into my radar instead of using images but as I wrote in my first post, I'm just beginning to understand the concepts of GUI scripting so it will take some time I guess :D I'm gonna memorize your suggestion and turn it into the next great update :D -
How do u change the spawn degree of a building spawned from a script
xxanimusxx replied to iwantfun's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Questions: What is "Castlegate" and why do you get the position of it? If this is a gamelogic, why not replacing it wholly with this command or a marker? O_O What do you mean with "degree"? Is this some sort of translation quirk or did you really mean the direction the building should face? If you meant the direction, use BoltboxerPro's suggestion, but put an underscore at the very beginning, otherwise it won't work. -
CursorTarget : Need to stop it returning all objects.
xxanimusxx replied to marker's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Okay the following code is working: while {true} do { if(player != vehicle player) then { _name = ""; _vehicle = assignedVehicle player; _vehname= getText (configFile >> "CfgVehicles" >> (typeOf vehicle player) >> "DisplayName"); _weapname = getarray (configFile >> "CfgVehicles" >> typeOf (vehicle player) >> "Turrets" >> "MainTurret" >> "weapons"); _weap = _weapname select 0; _name = format ["<t size='1.25' color='#556b2f'>%1</t><br/>", _vehname]; { _vehicleID = ""; _picture = ""; if((driver _vehicle == _x) || (gunner _vehicle == _x)) then { if(driver _vehicle == _x) then { _name = format ["<t size='0.85' color='#f0e68c'>%1 %2</t> <img size='0.7' color='#6b8e23' image=''/><br/>", _name, (name _x)]; } else { _target = cursorTarget; if (_target isKindOf "Car" || _target isKindOf "Motorcycle" || _target isKindOf "Tank" || _target isKindOf "Air" || _target isKindOf "Ship") then { _vehicleID = getText (configFile >> "cfgVehicles" >> typeOf _target >> "displayname"); _picture = getText (configFile >> "cfgVehicles" >> typeOf _target >> "picture"); }; _wepdir = (vehicle player) weaponDirection _weap; _Azimuth = round (((_wepdir select 0) ) atan2 ((_wepdir select 1) ) + 360) % 360; _name = format ["<t size='0.85' color='#f0e68c'>%1 %2</t> <img size='0.7' color='#6b8e23' image=''/><br/> <t size='0.85' color='#f0e68c'>Heading :<t/> <t size='0.85' color='#ff0000'>%3</t><br/><t size='0.85' color='#f0e68c'> Target :<t/> <t size='0.85' color='#ff0000'>%4</t><br/><t size='0.85' color='#f0e68c'> Display : </t><t size='0.85' color='#f0e68c'><img size='1' image='%5'/></t><br/>", _name, (name _x), _Azimuth,_vehicleID, _picture]; }; } else { _name = format ["<t size='0.85' color='#f0e68c'>%1 %2</t> <img size='0.7' color='#6b8e23' image=''/><br/>", _name, (name _x)]; }; } forEach crew _vehicle; hintsilent (parseText _name); }; sleep 1; }; You should set your variables to a default value if you want them to be assigned within a loop - I was getting "any" because of that! I was forced to delete the images' source because I did test this in ArmA2 and it gave me some errors... Use this to rebuild your HUD. -
CursorTarget : Need to stop it returning all objects.
xxanimusxx replied to marker's topic in ARMA 2 & OA : MISSIONS - Editing & Scripting
Just by the look of the above scripts it has to work :S But could I suggest something? Get the following variables above the while-loop: 1000 cutRsc ["HudNames","PLAIN"]; _ui = uiNameSpace getVariable "HudNames"; _HudNames = _ui displayCtrl 99999; You just need to define them once and also use cutRsc once to show your HUD, you don't need to constantly show it. The following command should normally output an error: _Azimuth = round (((_wepdir select 0) ) atan2 ((_wepdir select 1) ) + 360) % 360; because there doesn't seem to be any operator between atan2 and the previous bracket. Do you get any script errors? If so, please post all of them in this thread for us :> Also to be able to discern the source of your problems, use debug prints instead of GUI-commands, like "silenthint" or "sideChat" to output the _target and see if the target is a valid one. And the last thing: I'm not really sure but isn't your mission an ArmA3 one? Like I don't know of any map named "Stratis" for ArmA2 or is there an adaption? :S