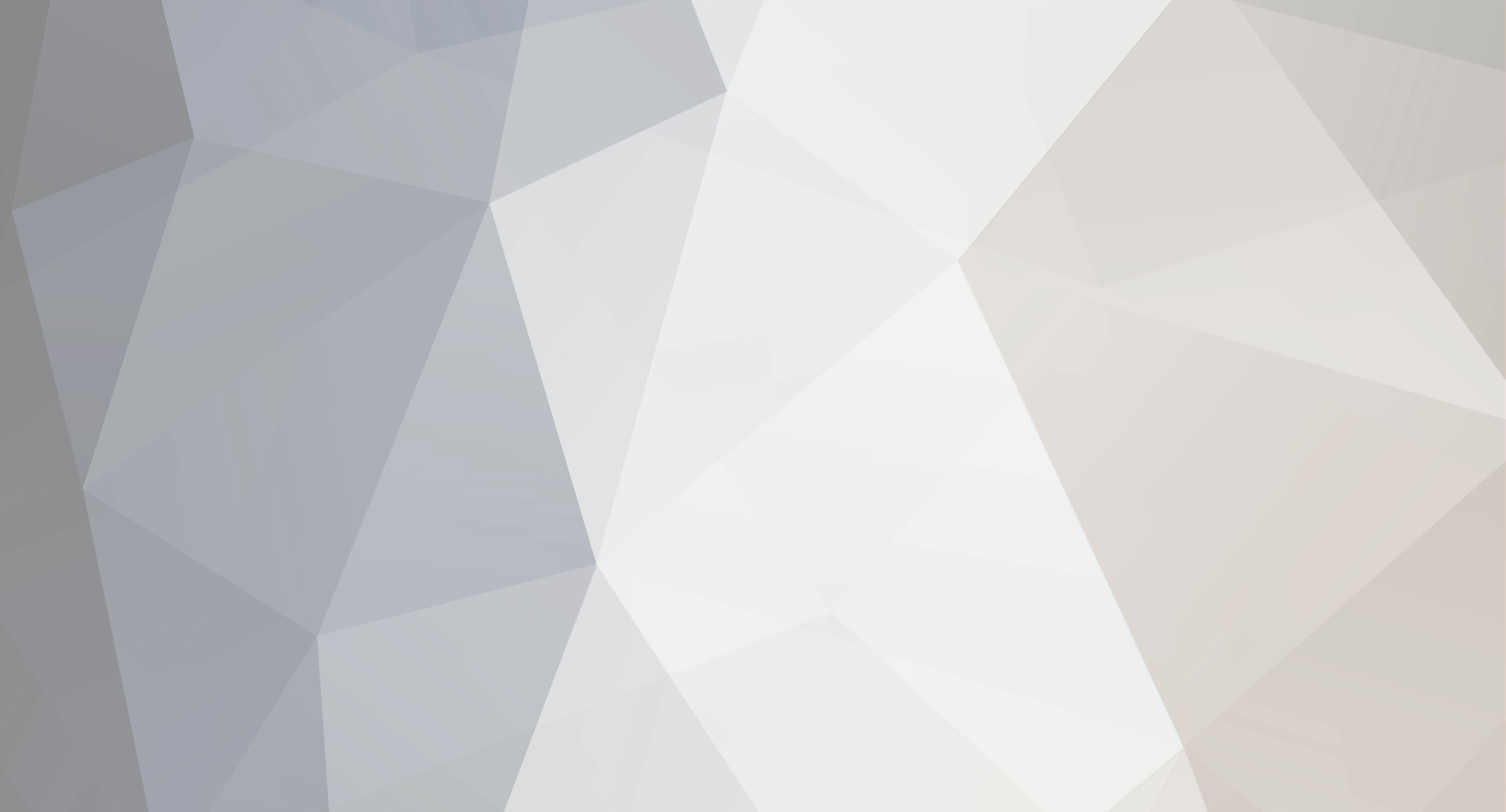

JCataclisma
Member-
Content Count
207 -
Joined
-
Last visited
-
Medals
Everything posted by JCataclisma
-
[READY] Create and Delete AI gunners for attack and support helicopters
JCataclisma posted a topic in ARMA 3 - MISSION EDITING & SCRIPTING
These are a couple of simple codes I am using, which are working both in editor and MP missions. I call them to add two permanent, different actions on the scroll menu, and they are shown only when player is on pilot seat, and to such pilot alone. Please note that "_currentVehicle" is a variable name, so you need to change it according to your situation - most likely replace it for "this" if you wanna use it on editor ("init" field on vehicle's properties), or for "cursorObject" (in case you wanna paste the whole code for immediately testing purposes - but remember to delete all comments after "//" in this case). For Kajman and Blackfoot: // This code is meant for use on Kajman (East) and Blackfoot (West) only; _currentVehicle addAction // replace "_currentVehicle" accordingly; [ "<t color='#f0cc0f'>Recruit AI Gunner</t>", { _aiGunner = (group player) createUnit ["B_Deck_Crew_F", [0,0,0], [], 0, "NONE"]; sleep 0.25; _aiGunner moveInGunner (vehicle player); _aiGunner setCombatBehaviour "COMBAT"; // check wiki for other options; _aiGunner setCombatMode "RED"; // check wiki for other options; }, nil, -8, false, true, "", "_this == _target turretUnit [-1]", //shown only for the pilot; 10, false ]; _currentVehicle addAction [ "<t color='#00000f'>Dismiss AI Gunner</t>", { _aiGunner = (vehicle player) turretUnit [0]; _aiGunner spawn { sleep 0.25; deleteVehicle _this; }; }, nil, -9, false, true, "", "_this == _target turretUnit [-1]", 10, false ]; And here is for Czapla (will probably work for Hellcat as well): // This code should work for helicopters Czapla and Hellcat; _currentVehicle addAction [ "<t color='#f0cc0f'>Recruit AI Gunner</t>", { _aiLeftGunner = (group player) createUnit ["B_Deck_Crew_F", [0,0,0], [], 0, "NONE"]; sleep 0.25; _aiLeftGunner moveInCargo [(vehicle player), 1]; removeAllWeapons _aiLeftGunner; _aiLeftGunner addWeapon "srifle_LRR_camo_LRPS_F"; _aiLeftGunner addMagazine "7Rnd_408_Mag"; _aiLeftGunner addMagazine "7Rnd_408_Mag"; _aiLeftGunner addMagazine "7Rnd_408_Mag"; _aiLeftGunner setCombatBehaviour "COMBAT"; _aiLeftGunner setCombatMode "RED"; sleep 0.25; _aiRightGunner = (group player) createUnit ["C_Man_formal_4_F", [0,0,0], [], 0, "NONE"]; sleep 0.25; _aiRightGunner moveInCargo [(vehicle player), 0]; _aiRightGunner addWeapon "srifle_LRR_camo_LRPS_F"; _aiRightGunner addMagazine "7Rnd_408_Mag"; _aiRightGunner addMagazine "7Rnd_408_Mag"; _aiRightGunner addMagazine "7Rnd_408_Mag"; _aiRightGunner setCombatBehaviour "COMBAT"; _aiRightGunner setCombatMode "RED"; sleep 0.25; }, nil, -8, false, true, "", "_this == _target turretUnit [-1]", 10, false ]; _currentVehicle addAction [ "<t color='#00000f'>Dismiss AI Gunner</t>", { // The vehicle the player is in _vehicle = vehicle player; // Get the units in cargo index 0 and cargo index 1 // cargo 0 is the 'shoot from vehicle' on the right side (pilot side, in this case), while cargo 1 is seated at the left side (co-pilot); _cargo0 = _vehicle call { _cargoUnits = crew _vehicle select {_x in (crew _vehicle select {assignedVehicleRole _x select 0 == "cargo"})}; if (count _cargoUnits > 0) then {_cargoUnits select 0} else {objNull}; }; _cargo1 = _vehicle call { _cargoUnits = crew _vehicle select {_x in (crew _vehicle select {assignedVehicleRole _x select 0 == "cargo"})}; if (count _cargoUnits > 1) then {_cargoUnits select 1} else {objNull}; }; _cargo0 spawn { sleep 0.25; deleteVehicle _this; }; _cargo1 spawn { sleep 0.25; deleteVehicle _this; }; }, nil, -9, false, true, "", "_this == _target turretUnit [-1]", 10, false ];- 1 reply
-
- 2
-
-
[READY] Create and Delete AI gunners for attack and support helicopters
JCataclisma replied to JCataclisma's topic in ARMA 3 - MISSION EDITING & SCRIPTING
The code below was changed to fit MH9 - Hummingbird, but it will probably NOT fit Pawnee. Note that there are three extra lines of code inside each addActions, which will REMOVE that action once executed. Those were added to prevent "infinite spawning" of gunners/shooters, which could be ejected by the player and then re-spawned. Such lines have the attached comment "// related to remove action once executed (line 1 of 3);" I did NOT add such "removeAction" on the previous code, but they can be easily pasted from here to there, at the same positions. _currentVehicle addAction [ "<t color='#f0cc0f'>Recruit AI Gunner</t>", { _recruitGunner = _this select 2; // related to remove action once executed (line 1 of 3); _currentHeli = (vehicle player); // related to remove action once executed (line 2 of 3); _aiLeftGunner = (group player) createUnit ["B_Deck_Crew_F", [0,0,0], [], 0, "NONE"]; sleep 0.25; _aiLeftGunner moveInCargo [(vehicle player), 3]; removeAllWeapons _aiLeftGunner; _aiLeftGunner addWeapon "srifle_DMR_02_camo_AMS_LP_F"; _aiLeftGunner addMagazine "10Rnd_338_Mag"; _aiLeftGunner addMagazine "10Rnd_338_Mag"; _aiLeftGunner addMagazine "10Rnd_338_Mag"; _aiLeftGunner setCombatBehaviour "COMBAT"; _aiLeftGunner setCombatMode "RED"; sleep 0.25; _aiRightGunner = (group player) createUnit ["B_Competitor_F", [0,0,0], [], 0, "NONE"]; sleep 0.25; _aiRightGunner moveInCargo [(vehicle player), 5]; _aiRightGunner addWeapon "srifle_LRR_camo_LRPS_F"; _aiRightGunner addMagazine "7Rnd_408_Mag"; _aiRightGunner addMagazine "7Rnd_408_Mag"; _aiRightGunner addMagazine "7Rnd_408_Mag"; _aiRightGunner setCombatBehaviour "COMBAT"; _aiRightGunner setCombatMode "RED"; sleep 0.25; _currentHeli removeAction _recruitGunner; // related to remove action once executed (line 3 of 3); }, nil, -8, false, true, "", "_this == _target turretUnit [-1]", 10, false ]; _currentVehicle addAction [ "<t color='#00000f'>Dismiss AI Gunner</t>", { _dismissGunner = _this select 2; // related to remove action once executed (line 1 of 3); _currentHeli = (vehicle player); // related to remove action once executed (line 2 of 3); // Get the vehicle the player is in _vehicle = vehicle player; // Get the units in cargo index 0 and cargo index 1 _cargo0 = _vehicle call { _cargoUnits = crew _vehicle select {_x in (crew _vehicle select {assignedVehicleRole _x select 0 == "cargo"})}; if (count _cargoUnits > 0) then {_cargoUnits select 0} else {objNull}; }; _cargo1 = _vehicle call { _cargoUnits = crew _vehicle select {_x in (crew _vehicle select {assignedVehicleRole _x select 0 == "cargo"})}; if (count _cargoUnits > 1) then {_cargoUnits select 1} else {objNull}; }; _cargo0 spawn { sleep 0.25; deleteVehicle _this; }; _cargo1 spawn { sleep 0.25; deleteVehicle _this; }; sleep 0.25; _currentHeli removeAction _dismissGunner; // related to remove action once executed (line 3 of 3); }, nil, -9, false, true, "", "_this == _target turretUnit [-1]", 10, false ]; -
A question about destructible and non-destructible prop items?
JCataclisma replied to avibird 1's topic in ARMA 3 - MISSION EDITING & SCRIPTING
You are definitely getting your degree as "Explosives Specialist"! 😁 I know that, since a year ago, Quiksilver has dealt with a lot of such stuff while upgrading and adding that kind of effects into his "Invade & Annex" framework, especially because he figured it out and created a system in which destroyed vehicles become wrecks, and such wrecks receive some special fire and/or smoke, and thus they can be towed back to base to be restored. I don't have the slightest idea about how he made it, but in case you wanna try and ask directly to him, he's still active on his Discord - not THAT much active, but he usually answers within a few days. He also has a specific topic for the framework here in forums, but I don't think he visits this place anymore. https://discord.gg/KQb8qtYw -
Need help to add a fuel pump explosion to a prop item
JCataclisma replied to avibird 1's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Also, take a look at the second part of this recent Gunter's video. It might give you even crazier ideas. 😎 -
Ok, found it. But, so far, it doesn't have any additional addAction as you wished - only the original action from the switch. I have several objects "Land_PortableLight_02_single_folded_black_F" attached to a vehicle, as well as a switch box "Land_TransferSwitch_01_F". There is also a game logic. All of these objects ares synched (right-click => synch): lamps, switch box and logic. And this is what's on LOGIC's attributes -> Init: [this, vangnamStyle] call BIS_fnc_attachToRelative; // I needed the switch box attached to the van - unnecessary in case of static (but functional) switch; _objs = synchronizedObjects this; _switch = _objs select{ _x isKindOf "Land_TransferSwitch_01_F" } select 0; _lights = _objs select { (_x isKindOf "Land_PortableLight_02_single_folded_olive_F") || (_x isKindOf "Land_PortableLight_02_single_folded_sand_F") || (_x isKindOf "Land_PortableLight_02_single_folded_black_F") }; TAG_fnc_lightsState = { params[ "_lights", "_lightState" ]; { _x switchLight _lightState; }forEach _lights; }; _switch setVariable[ "LightActionID", _switch addAction[ "SuperLights Off", { params [ "_target", "_caller", "_id", "_args" ]; _args params[ "_lights" ]; _info = if ( _target animationSourcePhase "SwitchPosition" == 0 ) then { [ "OFF", "SuperLights On", 1, 0 ] }else{ [ "ON", "SuperLightsLights Off", 0, 1 ] }; _info params[ "_lightState", "_actionText", "_switchPosition", "_switchLight" ]; _target animateSource[ "SwitchPosition", _switchPosition ]; _target animateSource[ "SwitchLight", _switchLight ]; [ [ _target, _actionText ], { params[ "_target", "_actionText" ]; _target setUserActionText[ _target getVariable "LightActionID", _actionText ] } ] remoteExec[ "BIS_fnc_call", 0, format[ "%1_actionState", _target call BIS_fnc_objectVar ] ]; [ _lights, _lightState ] remoteExec[ "TAG_fnc_lightsState", 0, format[ "%1_lightState", _target call BIS_fnc_objectVar ] ]; }, [ _lights ], 1, true, false ] ];
-
Man, I have done a very similar thing on those "folded camp lamps" I attached to a helicopter, but it was more than a year ago. I will run a better search, but I am sure I got it from a topis here in forums, most likely from Larrow, and it also got the "synch" in editor involved (right-click -> synch). Here is one of topics which have also helped me then, but they're using triggers, instead.
-
[SOLVED] Script to center helicopter gunner's aiming at pilot's view/screen?
JCataclisma posted a topic in ARMA 3 - MISSION EDITING & SCRIPTING
INFORMATION NOTE: THIS FIRST MESSAGE CONTAINS THE STARTING DOUBTS AND THE WRONG CODE. Please scroll down a bit to see another message containing the correct applied code. ============================================ Hello, guys! I have watched some gameplays in which players entered helicopters like Kajman and Blackfoot, without neither other player or AI on gunner seat, and every time they switched to Manual Fire, the aiming of the gun instantly and automatically "centers" to match the pilot's view. Unfortunately, I don't have access to any script, PBO or mission files, to try and search what those servers' admins might have used. I have found a "Talk" piece on Arma 3 Wiki, in which KilzoneKid and AgentRev posted some comments to such specific situation, but I wasn't able to make it work by myself. https://community.bistudio.com/wiki/Talk:animateSource The code below might be the closed I have come so far. I get no errors, and both "hints" are shown on screen, so it runs completely. But I got no aiming movement at all. I have even tried a suggestion to ad some permanent aiming coordinates on screen, for debug purpouses, and it worked great, but still no movement. Have also performed some attempts with "eyePos", still no luck at all. https://community.bistudio.com/wiki/eyePos Any suggestions where could I go from here to make that gun automatically centered? Cheers! 😎 NOTE: In the videos I have seen, the stuff is done automatically when activating Manual Fire. Here, I am initially trying it by using a "Zeroing" action that checks whether manual fire is on, prior to try and perform stuff.- 3 replies
-
- helicopter aiming
- gunship weapons
- (and 3 more)
-
[SOLVED] Script to center helicopter gunner's aiming at pilot's view/screen?
JCataclisma replied to JCataclisma's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Well, it happened that I was in the very right track, following that "talk" link regarding AnimateSource. But I forgot that some lines at the top of my code were blocking the access to the gunship's gunner seats, so the fake agent or unit created was not allowed to perform the moveInGunner, thus the gun would never move.Already got my Facepalm Award for the week. 🤦♂️ Here is the code I am using, almost completely like the wiki: _fakeGunner = createAgent ["B_UAV_AI", [0,0,0], [], 0, "NONE"]; sleep 0.25; _fakeGunner moveInGunner (vehicle player); _fakeGunner lockCameraTo [(vehicle player),[0],false]; _fakeGunner spawn { sleep 2; deleteVehicle _this; };- 3 replies
-
- 2
-
-
- helicopter aiming
- gunship weapons
- (and 3 more)
-
[SOLVED] Script to center helicopter gunner's aiming at pilot's view/screen?
JCataclisma replied to JCataclisma's topic in ARMA 3 - MISSION EDITING & SCRIPTING
That's a really simple and efficient idea. I will put it for another use I got in mind, thank you!- 3 replies
-
- helicopter aiming
- gunship weapons
- (and 3 more)
-
Action heal in car
JCataclisma replied to Davis Welder's topic in ARMA 3 - MISSION EDITING & SCRIPTING
It seems like your best bet is to go for ACE mod: https://steamcommunity.com/sharedfiles/filedetails/?id=463939057&searchtext=ace -
Adding an addAction to a specific door
JCataclisma replied to The Rook's topic in ARMA 3 - MISSION EDITING & SCRIPTING
As far as I remember, player having a key or any other object on their inventory won't help anything to perform changes on what happens around them. I think the closest I have seen to what you desire was that once the player finds a key, such key would have an addAction on it that would hence unlock some specific door for that player. I cannot find the "correct" post here in forums I used to keep as a guide for such. Or maybe in a more "easy" way: you add that key where it should be found, and give it a "variable name" on the editor. Create a trigger that's activated by the player, and such trigger will teleport the key closer to the door you wish to unlock. At such door, there could be another trigger that is activated/synchronized when the key is present, so to switch the building's door status to unlocked. -
Need help to add a fuel pump explosion to a prop item
JCataclisma replied to avibird 1's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Also, take a look at this: -
Need help to add a fuel pump explosion to a prop item
JCataclisma replied to avibird 1's topic in ARMA 3 - MISSION EDITING & SCRIPTING
I cannot access the game today to verify, but have you tried looking into the option "View in Config Files", after right-clicking the object while using the mission editor? There is a sub-menu called "Animation Sources", which usually contains the hit marks for the object and could give you some names to try. ================ EDIT: I have never tried what you are doing, but this is a line I have from that station in a text editor; maybe it could help: configfile >> "CfgVehicles" >> "Land_fs_feed_F" >> "DestructionEffects" >> "FuelDestr" -
I probably miss something but what?
JCataclisma replied to Belial0harvester's topic in ARMA 3 - MISSION EDITING & SCRIPTING
The thing that I have noticed is that --on the MEDIC trait, you have an additional "getUnitTrait", which is not present at the others. Try and remove that "getUnitTrait" line to see if the error stops. Or even better: according to Wiki, you should try the other syntax: player setUnitTrait ["Medic", true]; _med = "uns_men_USMC_65_MED" createUnit [getMarkerPos "ret4", _group]; _med setUnitRank "CORPORAL"; // _med getUnitTrait "Medic"; // try changing this one as bellow or removing this one, for starters; _med setUnitTrait ["Medic", true]; You see, the error you received from the game is prior to "_gl", so it's most likely related to that medic line right before it. -
How do you make a gbu-12 bomb spawn with a trigger?
JCataclisma replied to HistoryFan_13654's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Do you want it to explode as well, or that it's just spawned? You could add full damage to into to force explosion: setDamage [1,TRUE]; _boom="Bo_GBU12_LGB" createVehicle getPos whatever; _boom setDamage [1,true]; And in case you want to follow that traditional suggestion of initially creating the object on the "0,0,0" mark, and then move it to desired position immediately after: _boom = createVehicle ["Bo_GBU12_LGB",[0,0,0]]; _boom setPos (getPos whatever); _boom setDamage [1,true]; -
Flare from Supply Drop
JCataclisma replied to DMRocks's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Finally, there are also Pierre-MGI's modules, which handle that subjects as well: https://steamcommunity.com/sharedfiles/filedetails/?id=1682280809 ============ Edit/Note This is what I use to create a smoke on vehicles at the point where I disembark them from boats (by using "attachTo" in scripts) _charge_9 = createVehicle ["SmokeShellYellow",[0,0,0]]; _charge_9 setPosATL (_object modelToWorldVisual [0,0,0]); _charge_9 setVectorDirAndUp [(_object modelToWorld [0,0,0]) vectorFromTo (_object modelToWorld [0,0,0]),[0,0,2]]; _charge_9 setDamage [1,TRUE]; -
Flare from Supply Drop
JCataclisma replied to DMRocks's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Try ant take a look at this recent video from Gunter. I think it will give you exactly what is missing. 😎 I would also change your createVehicle line into something like this: flrObj = "F_20mm_Red" createvehicle ((flrpos) ModelToWorld [0,0,50]); There also this topic from a few hours ago, where I put the code I use to spawn/create flares relative to the player's position when they use an action, so you can use the "createvehicle ((player) ModelToWorld" from there: -
Take a look on the page regarding "magic variables". It will probably help you understand a little more about that "this/_this" difference but, in a very general way, "this" concerns to the physical object which you are dealing with, while "_this" will tell the script that you are messing witch an element and/or variable name inside the script, regarding it's an object or not. For multiplayer, you will probably never use "this". Usually, "this" will work mostly of time in editor because we commonly write the script at the init box of the object itself, so anything there should not exactly affect the rest of the stuff. https://community.bistudio.com/wiki/Magic_Variables
-
I think you might NOT achieve the result you're looking for by using this method, because as far as I remember, the original smoke screen on tanks is actually a weapon, not a "feature". There are ways to create "fake" weapons on vehicles to make them shoot pretty much any types of weapons we want, but I have never tried it for this. 🤔 I will mess with it a little bit and inform here in case of any good news!
-
There is a parameter which allow you to define a custom key for any "addAction" you put in your scripts. Take a look at this last part of the code I have previously posted, but now I have added a "user16" . That will tell the script that whenever the player uses the key configured as the "custom16" (see settings -> controls -> custom), it will execute this action. You can have several "addActions" with the same key set, so they will trigger simultaneously once the custom control is pressed, but it can also be the same key already set for a mod, for instance, so there might be and easy-to-solve conflict. But you just need to check those control settings prior to set a "user" key, and everything is fine. }, nil, -3, false, true, "user16", // here you chose which custom key will trigger your action; "_this == _target turretUnit [-1] && (getPosATL _target) select 2 < 16", 10, false ]; Oh, and I also forgot to mention that you don't exactly need the whole line bellow, as everything after the "&&" is there just because I wanted to avoid the action showing unless vehicle was flying bellow 17 meters height. So you could NOT use this: "_this == _target turretUnit [-1] && (getPosATL _target) select 2 < 16" ...and just replace it for this instead: "_this == _target turretUnit [-1]"
-
My apologies! Don't know where my mind was, and I have completely messed up: my idea was to suggest the ACTUAL smoke, not the flares! Dang it! Regardless, here is a SMOKE spawning, instead of flares. Cheers! _thids addAction [ "<t color='#24c048'>SMOKE</t>", { chemLitW2 = "SmokeShell" createvehicle ((player) ModelToWorld [-7,5,1]); chemLitN = "SmokeShellGreen" createvehicle ((player) ModelToWorld [0,7,1]); chemLitE = "SmokeShell" createvehicle ((player) ModelToWorld [7,0,1]); chemLitE2 = "SmokeShell" createvehicle ((player) ModelToWorld [7,5,1]); chemLitS = "SmokeShellGreen" createvehicle ((player) ModelToWorld [0,-16,1]); chemLitSW = "Chemlight_yellow" createvehicle ((player) ModelToWorld [-5,-13,1]); chemLitSE = "Chemlight_yellow" createvehicle ((player) ModelToWorld [5,-13,1]); chemLitW = "SmokeShell" createvehicle ((player) ModelToWorld [-7,0,1]); chemLitW2 = "SmokeShell" createvehicle ((player) ModelToWorld [-19,17,1]); chemLitN2 = "SmokeShellGreen" createvehicle ((player) ModelToWorld [0,19,1]); chemLitE2 = "SmokeShell" createvehicle ((player) ModelToWorld [19,0,1]); chemLitE22 = "SmokeShell" createvehicle ((player) ModelToWorld [19,17,1]); chemLitS2 = "SmokeShellGreen" createvehicle ((player) ModelToWorld [0,-13,1]); chemLitSW2 = "Chemlight_yellow" createvehicle ((player) ModelToWorld [-17,-19,1]); chemLitSE2 = "Chemlight_yellow" createvehicle ((player) ModelToWorld [17,-19,1]); chemLitW2 = "SmokeShell" createvehicle ((player) ModelToWorld [-19,0,1]); }, nil, -3, false, true, "", "_this == _target turretUnit [-1] && (getPosATL _target) select 2 < 16", 10, false ];
-
Or, if you want to try and go a little bit crazier, add this to the init checkbox of the vehicle (in editor) or execute in the pause menu debug/script console. It will add an action to spawn a nice collection of flares on the pilot/driver's menu. If it doesn't work at first attempt, replace "this" with "_this", or point the cursor to the cabin of the vehicle you want to add this action and replace "this" with "cursorObject". this addAction // (you might need to replaced this to _this instead; [ "<t color='#cb8f0f'>Night FLARES</t>", { if (!isNil "flaresLastSpawned" && {flaresLastSpawned > time - 42}) exitWith { // (a little cooldown to avoid spawning them insanely; hint "Cooldown 42 secs"; }; flaresLastSpawned = time; flareN = "F_20mm_Green" createvehicle ((player) ModelToWorld [0,50,150]); flareN setVelocity [0,25,-5]; flareNE = "F_20mm_Yellow" createvehicle ((player) ModelToWorld [15,25,150]); flareNE setVelocity [5,15,-15]; flareE = "F_20mm_Red" createvehicle ((player) ModelToWorld [15,0,150]); flareE setVelocity [10,25,-5]; flareSE = "F_20mm_Yellow" createvehicle ((player) ModelToWorld [15,-25,150]); flareSE setVelocity [-5,-15,-15]; flareS = "F_20mm_Green" createvehicle ((player) ModelToWorld [0,-50,150]); flareS setVelocity [0,-25,-5]; flareSW = "F_20mm_Yellow" createvehicle ((player) ModelToWorld [-15,-25,150]); flareSW setVelocity [-5,-15,-15]; flareW = "F_20mm_Red" createvehicle ((player) ModelToWorld [-15,0,150]); flareW setVelocity [-10,25,-5]; flareNW = "F_20mm_Yellow" createvehicle ((player) ModelToWorld [-15,25,150]); flareNW setVelocity [-5,15,-15]; }, nil, -3, false, true, "User16", "_this == _target turretUnit [-1] && (getPosATL _target) select 2 < 150", // the [-1] means it will show only for pilot/driver; 10, false ];
-
[SOLVED] How to board attached static weapons using addAction on a vehicle?
JCataclisma replied to JCataclisma's topic in ARMA 3 - MISSION EDITING & SCRIPTING
OH, indeed! My apologies: now, after your latest message, I've read once more your previous issue and realized that it was I who has misunderstood what was actually the difficulty. Cheers! -
[SOLVED] How to board attached static weapons using addAction on a vehicle?
JCataclisma posted a topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hello, guys! I have once played in a mission in which player would "automatically" assume the gunner position in one of the specific sentries that were attached to a vehicle, once they clicked "Get in as passenger" for some seats. In my current case, I'd like the player manning the right static mounted AT when clicking to getin the rear right passenger seat, and manning as a gunner for the HMG by clicking to getIn as the left rear passenger. I know that, for the NATO Prowler, these are the Cargo4/cargoTurret_04 and Cargo5/cargoTurret_05 seats. I use the script bellow to successfully attach the desired weapons to a nearby vehicle of the specific class, once I execute an addAction that calls such script. But I am stuck regarding the eventHandler situation, because when using 'GetIn' I got a "generic error in expression", and by using GetInMan instead, nothing happens - I believe is because the latter one should work only for units and not vehicles. So it seems the code bellow is the closer I've got so far. Any suggestions regarding how could I change it so that "Get in as passenger right seat 3" would NOT seat, but man the "_objMidTowerD" turret, and so that "get is passenger left seat 3" would make the player board as gunner for the "_objLeftGun" object? Cheers! ============ P.s.: currently, I also use the lines _currentVehicle lockCargo [4, true]; _currentVehicle lockCargo [5, true]; , so that nobody can actually seat on those places while the weapons are attached, but I DON'T use these when attempting to use eventHandler. private _nearbyVehicles = nearestObjects[player, ["Car"], 15]; { private _currentVehicle = _x; if (typeName _currentVehicle == "OBJECT" && (typeOf _currentVehicle in ["B_T_LSV_01_unarmed_F", "B_LSV_01_unarmed_F"])) then { _objLeftGun = "B_HMG_01_high_F" createVehicle [0, 0, 0]; _objLeftGun attachTo [_currentVehicle, [-0.7, -1.4, 0.7]]; _objLeftGun setDir 210; _objLeftGun enableWeaponDisassembly FALSE; _objMidTowerD = "B_T_Static_AT_F" createVehicle [0, 0, 0]; _objMidTowerD attachTo [_currentVehicle, [0.4, -1.7, 0.2]]; _objMidTowerD setDir 30; _objMidTowerD enableWeaponDisassembly FALSE; _currentVehicle addEventHandler ["GetIn", { params ["_vehicle", "_unit", "_role", "_turret"]; if (_unit == player && _role == 'cargo') then { player moveInAny _objMidTowerD; }; }]; _currentVehicle addEventHandler ["GetIn", { params ["_vehicle", "_unit", "_role", "_turret"]; if (_unit == player && _role == 'cargo' && _turret == 5) then { player moveInTurret [_objLeftGun, [0]]; }; }]; }; } forEach _nearbyVehicles; -
Simple Single Player Cheat Menu
JCataclisma replied to benargee's topic in ARMA 3 - ADDONS & MODS: COMPLETE
Thank you, Janez. But for the record, I gotta mention that, at least for me, it worked just perfectly from the very first attempt on my recently restart on "Old Man". 😎 Cheers!