Sign in to follow this
Followers
0
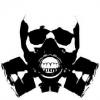
MP compatability
By
iceman77, in ARMA 2 & OA : MISSIONS - Editing & Scripting
By
iceman77, in ARMA 2 & OA : MISSIONS - Editing & Scripting