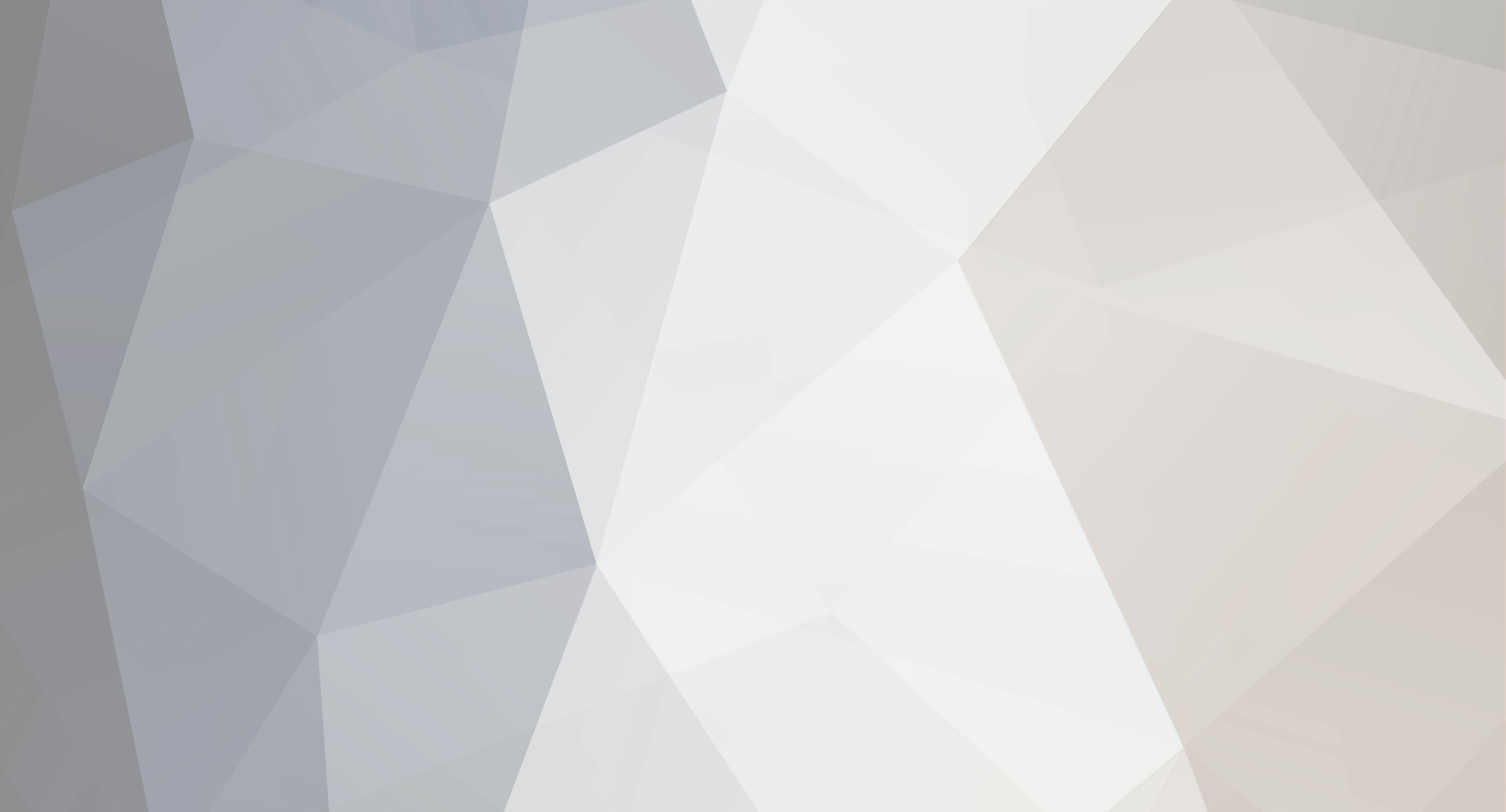

kovvalsky
-
Content Count
67 -
Joined
-
Last visited
-
Medals
Posts posted by kovvalsky
-
-
on cfgVehicles >> "C_Hatchback_01_F" there is a parameter named brakeIdleSpeed..
brakeIdleSpeed is speed in m/s under which the brakes are automatically applied to the vehicle. This speed should be reasonably low, higher value would mean strange breaking of slow cars, too low value would cause inability to stop the car.
(maybe we can override this parameter)
-
-
try to assign default value if westscore not exist:
_wscore = server_1 getVariable "westscore";
_wscore = server_1 getVariable ["westscore","westscore not ready"];
-
this also works..
MISSION_ROOT = call { private "_arr"; _arr = toArray str missionConfigFile; _arr resize (count _arr - 15); toString _arr }; playSound3D [MISSION_ROOT + "yourSoundsFolder\yourSound.ogg", _SoundSource, _isInside, _SoundPos, _SoundVolume, _SoundPitch, _SoundDistance];
-
I've done something similar recently:
this is the script used on AddAction (Detector: ON):
oDetector is an object attached to the player (player can take or drop the detector)
oHerramienta is the object to find.
Both placed on editor
_accObjeto = [_this, 0, objNull] call BIS_fnc_param; _accJugador = [_this, 1, objNull] call BIS_fnc_param; _accID = [_this, 2, -1] call BIS_fnc_param; _accArgument = [_this, 3, []] call BIS_fnc_param; oDetector setVariable ['Detector_ON',1,true]; _tiempoGuardado = time; while {((oDetector getVariable ['Detector_ON',0]) == 1)} Do { //if player drop the detector... if ((oDetector getVariable ['Detector_TAKE',1]) == 0) exitWith {oDetector setVariable ['Detector_ON',0,true];}; if (time > _tiempoGuardado) then { playSound3D [MISSION_ROOT + "Sounds\Detector_BEEP.ogg", oDetector, false, (getPosASL oDetector), 4, 1, 40]; //BEARING _pos1 = getpos oHerramienta; _pos2 = getpos _accJugador; _dir1 = ((_pos1 select 0) - (_pos2 select 0)) atan2 ((_pos1 select 1) - (_pos2 select 1)); _dir2 = getdir _accJugador; if (_dir1 < 0) then {_dir1 = 360 + _dir1}; _diff = (_dir2 max _dir1) - (_dir2 min _dir1); if (_diff > 180) then {_diff = abs(_diff - 360)}; _restoDir = ((0.5 * _diff) / 180); //DISTANCE _restoDis = ((oDetector distance oHerramienta)/100); _tiempoGuardado = (time + 0.05) + (_restoDis + _restoDir); }; };
this line can be modified (the script is wip)
_restoDis = ((oDetector distance oHerramienta)/100);
/100 is the max distance
the script emit a BEEP every 0.05 secs plus the distance between the object and player, plus bearing
may be you can take any idea..
-
Only other workaround I can think of would be to update the position of the light manually on each frame with modelToWorld. Though that would be a bit resource intensive.
Very expensive, plus I think they must be local, for use in multiplayer you should use BIS_fnc_MP to create them on each computer
-
-
ok, to use addaction on all clients i usually use a function (on init) like this:
myAddActionFunction = { private ["_ActObj","_ActTitle","_ActScript","_ActArgumn","_ActDistan"]; _ActObj = [_this,0] call BIS_fnc_param; _ActTitle = [_this,1] call BIS_fnc_param; _ActScript = [_this,2] call BIS_fnc_param; _ActArgumn = [_this,3,[],["",[],0,objNull]] call BIS_fnc_param; _ActDistan = [_this,4,2,[0]] call BIS_fnc_param; if(isNull _ActObj) exitWith {}; _ActObj addAction [_ActTitle, _ActScript, _ActArgumn, 1, True, True, "", "(_target distance _this) < " + str(_ActDistan)]; }
and then i use BIS_fnc_MP like this:
_Arguments = [_paramOne, _paramTwo, _paramThree]; _ActionTitle = "AddAction test"; [[_ObjectToAttachAction,_ActionTitle,_ScriptToCall,_Arguments,_ViewActionDistance],"myAddActionFunction",nil,true] call BIS_fnc_MP;
i use nil to broadcast over all clients
and true to make persistent
_Arguments and _ViewActionDistance are Optional
-
can you explain why u need to pass this using BIS_fn_MP? you need an Action visible on all clients? or what u need?
-
yes, but BIS_fnc are a functions too.. you can go inside the function to see how it works via "Functions" on editor:
BIS_fnc_param
/* Author: Karel Moricky Description: Define script parameter Parameter(s): _this select 0: ARRAY - list of params _this select 1: NUMBER - selected index _this select 2 (Optional): ANY - default param (used when param is missing or of wrong type) - you can overload default value by setting 'BIS_fnc_<functionName>_<index>' _this select 3 (Optional): ARRAY - list of allowed type examples (e.g. ["",[],0,objnull]) _this select 4 (Optional): NUMBER - If value is ARRAY, checks if it has required number of elements Returns: ANY - either value from list of params, or default value */ /////////////////////////////////////////////////////////////////////////////////////////////////////////// #define FNC_DISABLEERRORS \ private ["_disableErrors"]; \ _disableErrors = false; // _disableErrors = if (count _this > 5) then {_this select 4} else {false}; #define FNC_TEXTTYPES \ private ["_textTypes"];\ _textTypes = "";\ {\ _textTypes = _textTypes + typename _x;\ if (_forEachIndex < count _types - 1) then {_textTypes = _textTypes + ", "};\ } foreach _types; /////////////////////////////////////////////////////////////////////////////////////////////////////////// private ["_params","_id","_value","_thisCount"]; //disableserialization; //--- Do not put this here or none of the scripts where BIS_fnc_param is used will be serialized! _thisCount = count _this; //if (typename _this != typename []) then {_this = [_this]}; _params = if (_thisCount > 0) then {_this select 0} else {[]}; _id = if (_thisCount > 1) then {_this select 1} else {0}; if (typename _params != typename []) then {_params = [_params]}; //if (typename _id != typename 00) then {_id = 0}; _value = if (count _params > _id) then {_params select _id} else {nil}; //--- Assign default value if (_thisCount > 2) then { private ["_default","_defaultOverload","_types","_typeDefault","_type"]; _default = _this select 2; //--- Overload default value #ifndef DISABLE_REWRITE if !(isnil "_fnc_scriptName") then { _defaultOverload = missionnamespace getvariable (_fnc_scriptName + "_" + str _id); if !(isnil "_defaultOverload") then { _default = _defaultOverload; }; }; #endif //--- Default set if (isnil "_value") then { _value = _default; }; //--- Check type if (_thisCount > 3) then { _types = _this select 3; //if (typename _types != typename []) then {_types = [_types]}; _type = typename _value; _typeDefault = typename _default; if !({_type == typename _x} count _types > 0) then { if ({_typeDefault == typename _x} count _types > 0) then { FNC_DISABLEERRORS if (!_disableErrors) then { FNC_TEXTTYPES ["#%1: %2 is type %3, must be %4. %5 used instead.",_id,str _value, _type, _textTypes, str _default] call bis_fnc_error; }; _value = _default; } else { FNC_DISABLEERRORS if (!_disableErrors) then { FNC_TEXTTYPES ["#%1: Default %2 is type %3, must be %4",_id, str _default, _typeDefault, _textTypes] call bis_fnc_error; }; }; }; }; //--- Check number of elements (ARRAY type only) if (_thisCount > 4) then { if (typename _value == typename []) then { private ["_valueCountRequired","_valueCount"]; _valueCountRequired = [_this,4,0,[0,[]]] call bis_fnc_param; if (typename _valueCountRequired != typename []) then {_valueCountRequired = [_valueCountRequired]}; _valueCount = count _value; if !(_valueCount in _valueCountRequired) then { _value = _default; ["#%1: %2 elements provided, %3 expected: %4",_id,_valueCount,_valueCountRequired,_value] call bis_fnc_error; }; }; }; _value } else { if (isnil "_value") then {nil} else {_value} };
BIS_fnc_inTrigger
// /* File: inTrigger.sqf Author: Karel Moricky Description: Detects whether is position within trigger area. Parameter(s): _this select 0: OBJECT or ARRAY - Trigger or trigger area _this select 1: ARRAY or OBJECT - Position _this select 2 (Optional): BOOL - true for scalar result (distance from border) Returns: Boolean (true when position is in area, false if not). */ private ["_trig","_position","_scalarresult","_tPos","_tPosX","_tPosY","_tArea","_tX","_tY","_tDir","_tShape","_in"]; _trig = [_this,0,objnull,[objnull,[],""]] call bis_fnc_param; _position = [_this,1,[0,0,0]] call bis_fnc_param; _scalarresult = [_this,2,false,[false]] call bis_fnc_param; if (typename _trig == typename objnull) then { if (_trig iskindof "emptydetector") then {_trig = [position _trig,triggerarea _trig];} else {_scalarresult = 0;}; }; if (typename _scalarresult == typename 00) exitwith {["%1 is not a trigger",_trig] call bis_fnc_error; false}; if (typename _trig == typename "") then {_trig = [markerpos _trig,markersize _trig + [markerdir _trig,markershape _trig == "rectangle"]]}; //--- Trigger position _position = _position call bis_fnc_position; _position resize 2; _tPos = [_trig,0,[0,0,0],[[],objnull]] call bis_fnc_paramin; if (typename _tPos == typename objnull) then {_tPos = position _tPos}; _tPos resize 2; _tPosX = [_tPos,0,0,[0]] call bis_fnc_paramin; _tPosY = [_tPos,1,0,[0]] call bis_fnc_paramin; if (_position distance [_tPosX,_tPosY] == 0) then {["Compared positions '%1' and '%2' cannot be the same.",_position,_trig] call bis_fnc_error; _tPosY = _tPosY + 0.01;}; //--- Trigger area _tArea = [_trig,1,0,[[],0]] call bis_fnc_paramin; if (typename _tArea == typename 0) then {_tArea = [_tArea,_tArea,0,false]}; _tX = [_tarea,0,0,[0]] call bis_fnc_paramin; _tY = [_tarea,1,0,[0]] call bis_fnc_paramin; _tDir = [_tarea,2,0,[0]] call bis_fnc_paramin; _tShape = [_tarea,3,false,[false]] call bis_fnc_paramin; _in = false; if (_tshape) then { private ["_difX","_difY","_dir","_relativeDir","_adis","_bdis","_borderdis","_positiondis"]; //--- RECTANGLE _difx = (_position select 0) - _tPosx; _dify = (_position select 1) - _tPosy; _dir = atan (_difx / _dify); if (_dify < 0) then {_dir = _dir + 180}; _relativedir = _tdir - _dir; _adis = abs (_tx / cos (90 - _relativedir)); _bdis = abs (_ty / cos _relativedir); _borderdis = _adis min _bdis; _positiondis = _position distance _tPos; _in = if (_scalarresult) then { _positiondis - _borderdis; } else { if (_positiondis < _borderdis) then {true} else {false}; }; } else { private ["_e","_posF1","_posF2","_total","_dis1","_dis2"]; //--- ELLIPSE _e = sqrt(_tx^2 - _ty^2); _posF1 = [_tPosx + (sin (_tdir + 90) * _e),_tPosy + (cos (_tdir + 90) * _e)]; _posF2 = [_tPosx - (sin (_tdir + 90) * _e),_tPosy - (cos (_tdir + 90) * _e)]; _posF1 resize 2; _posF2 resize 2; _total = 2 * _tx; _dis1 = _position distance _posF1; _dis2 = _position distance _posF2; _in = if (_scalarresult) then { ((_dis1 + _dis2) - _total) * 0.5; } else { if (_dis1 + _dis2 < _total) then {true} else {false}; }; }; _in
BIS_fnc_countdown
/* Author: Karel Moricky Description: Trigger countdown Parameter(s): 0: NUMBER - countdown in seconds 1: BOOL - true to set the value globally Returns: NUMBER */ private ["_countdown","_isGlobal"]; _countdown = [_this,0,0,[0,true]] call bis_fnc_param; _isGlobal = [_this,1,true,[true]] call bis_fnc_param; switch (typename _countdown) do { case (typename 0): { private ["_servertime"]; _servertime = if (ismultiplayer) then {servertime} else {time}; switch true do { case (_countdown < 0): { missionnamespace setvariable ["bis_fnc_countdown_time",nil]; if (_isGlobal) then {publicvariable "bis_fnc_countdown_time";}; -1 }; case (_countdown == 0): { private ["_time"]; _time = missionnamespace getvariable "bis_fnc_countdown_time"; if (isnil "_time") then { -1 } else { (_time - _servertime) max 0 }; }; case (_countdown > 0): { private ["_time"]; _time = _servertime + _countdown; missionnamespace setvariable ["bis_fnc_countdown_time",_time]; if (_isGlobal) then {publicvariable "bis_fnc_countdown_time";}; _time }; default { -1 }; }; }; case (typename true): { ([] call bis_fnc_countdown) > 0 }; default { -1 }; };
-
/* ------------------------------------------------------------------------------ FUNCION: fnc_RestriccionZonaCombate ------------------------------------------------------------------------------ DESCRIPCION: Restriccion del jugador a la zona de combate. Se debe ejecutar cada vez que se hace respawn en cada cliente ------------------------------------------------------------------------------ PARAMETROS: 0: [sTRING] marker name 1: [iNTEGER] Countdown 2: [OBJECT] Player ------------------------------------------------------------------------------ EJEMPLO: - Crear un marcador con un area de 50m llamado "mkZonaCombate" 0 = ["mkZonaCombate",15,player] spawn fnc_RestriccionZonaCombate; ------------------------------------------------------------------------------ */ private ["_marcadorArea", "_soldado", "_segundos"]; _marcadorArea = [_this,0] call BIS_fnc_param; _soldado = [_this,1,player,[objNull]] call BIS_fnc_param; _segundos = [_this,2,15,[0]] call BIS_fnc_param; _CuentaAtras = 0; _bEnZonaCombate = false; _aviso = ""; if ((_marcadorArea != "") && ((markerShape _marcadorArea) != "ICON" )) then { waitUntil {alive _soldado}; while {(alive _soldado)} Do { _bEnZonaCombate = [_marcadorArea, (getPosATL _soldado)] call BIS_fnc_inTrigger; if (!_bEnZonaCombate) then { _CuentaAtras = [_segundos,false] call BIS_fnc_countdown; while {(!_bEnZonaCombate) && _CuentaAtras > 0} Do { _CuentaAtras = [0,false] call BIS_fnc_countdown; _aviso = parseText Format ["<t size='2' color='#FF0000' align='center' underline='true'>ATENCION</t><br/><t size='1' color='#FFFFFF'>FUERA DEL LIMITE</t><br/><t size='2.5' align='center'>%1</t><br/><t size='1'>PARA MORIR</t>", round (_CuentaAtras)]; hintSilent _aviso; sleep 0.2; _bEnZonaCombate = [_marcadorArea, (getPosATL _soldado)] call BIS_fnc_inTrigger; }; if (!_bEnZonaCombate) then { while {(alive _soldado)} Do { // [[_soldado,1,true],"BIS_fnc_moduleLightning",nil,false,true] call BIS_fnc_MP; // sleep 1; // code to kill a soldier // (i used a moduleLightning putit on editor with false on triger act, and change to true to kill) // [[_soldado],"CAOE_fnc_Relampago",false,false] call BIS_fnc_MP; }; } else { _aviso = parseText "<t size='2' color='#008000' align='center' >SIN PELIGRO</t>"; hintSilent _aviso; }; }; }; };
-
maybe you need to use BIS_fnc_MP with persistent = true on AddAction..
on init.sqf make a function to all:
fnc_MyAddActionFunction = { private ["_ActObj","_ActName","_ActScript","_ActArgumn","_ActCondition"]; _ActObj = [_this,0] call BIS_fnc_param; _ActName= [_this,1] call BIS_fnc_param; _ActScript = [_this,2] call BIS_fnc_param; _ActArgumn = [_this,3,[],["",[],0,objNull]] call BIS_fnc_param; _ActCondition = [_this,4, "(_target distance _this) < 3",[""]] call BIS_fnc_param; if(isNull _ActObj) exitWith {}; _ActObj addAction [_ActName, _ActScript, _ActArgumn, 1, True, True, "",_ActCondition]; };
and then replace:
_MHQ addAction["Deploy MHQ","Deploy.sqf",[_MHQ], 0, false, true, "", "_this in (crew _target) && {speed _target < 1}"];
with this
[[_MHQ,"<t color='#FFFF00'>Deploy MHQ</t>","Deploy.sqf",[],"_this in (crew _target) && {speed _target < 1}"],"fnc_MyAddActionFunction",nil,true] call BIS_fnc_MP;
-
thank you very much! I will be very grateful for anything you can contribute to the code. There may be many things wrong because I do not have much experience
-
Hello everyone!
I'm doing a mission and need to fix a lot of things I did not understand. In this community I have found very helpful info and I am very grateful.
First of all thanks for the time you dedicate.
I have to comment that the programming is done in Spanish (text, comments, variables, etc ...) I'm sorry for that, I will use stringtables later, when everything works well.
Without further ado, here goes:
MISSION FEATURES:
-COMBAT AREAwhich is delimited by a area marker(on editor). if you go out.. you die-MONEY / SHOP SYSTEM: You can buy everything, even improvements (as fatigue or additional backpack) and logistics (mercenaries, combat dogs, airstrike)-ATM SYSTEM: you can deposit at the ATM money you have.-INDIVIDUAL RESPAWN SYSTEM: you can choose which you appear via a sleeping bag. (player action)-SPY SATELLITE: simply, every x seconds the position of all players shown on the map.-MONEY DROPs: A helicopter flies over the combat zone and is destroyed. On landing the money appear within a radius 5 meters.-SUPPLY DROPs:A helicopter flies over the combat zone and drops a supply box with things.MISSION WORKFLOW:
- The player starts in a container, no clothes, no weapons. only a mapItem.- Have an initial $ ??? cash (specified on the lobby)- When you bought your gear, you'll jump to the combat zone through a map is inside the container.- When you touch the ground, planting the respawn action appears. if you do not plant, when you die, a random position is calculated to respawn.- When you die, you loose the money you have, but preserves all the equipment.- Every x seconds (specified on the lobby), money or supply drop appears over combat zone.- Every x seconds (specified on the lobby), spy satellite is activated.FILES (ver 1.0)
PROBLEMS
[PROB1] When you die on a vehicle (like parachute or quad) primaryWeapon is not stored:
i use a "killed" eventHandler to store all gear on a local public variable (EQUIPO):
script called when "killed" EH:
_Soldado = [_this,0] call BIS_fnc_param; _Asesino = [_this,1] call BIS_fnc_param; if ((_Asesino != _Soldado) && !(isNull _Asesino)) then { _MuertesAsesino = _Asesino getVariable ["MUERTES",-1]; if (_MuertesAsesino != -1) then { _MuertesAsesino = _MuertesAsesino + 1; _Asesino setVariable ["MUERTES", _MuertesAsesino, true]; } else { hint Format ["NO EXISTE LA VARIABLE EN %1",_Asesino]; }; }; _Ropa = uniform player; //UNIFORME _ContenidoRopa = uniformItems player; //CONTENIDO DEL UNIFORME _Chaleco = vest player; //CHALECO _ContenidoChaleco = vestItems player; //CONTENIDO DEL CHALECO _Mochila = backpack player; //MOCHILA _ContenidoMochila = backpackItems player; //CONTENIDO DE LA MOCHILA _ArmaPrincipal = primaryWeapon player; //ARMA PRINCIPAL _ArmaPriAcc = primaryWeaponItems player; //ACCESORIOS DEL ARMA PRINCIPAL _ArmaPriAmmo = []; //MUNICION ARMA PRINCIPAL [NombreClase,Cantidad] if (_ArmaPrincipal != "") then { if ((player ammo _ArmaPrincipal) > 0) then { _classMag = (primaryWeaponMagazine player) select 0; _countMag = player ammo _ArmaPrincipal; _ArmaPriAmmo = [_classMag,_countMag] ; }; }; _ArmaSecundaria = secondaryWeapon player; //ARMA SECUNDARIA _ArmaSecAcc = secondaryWeaponItems player; //ACCESORIOS DEL ARMA SECUNDARIA _ArmaSecAmmo = ""; if (_ArmaSecundaria != "") then { if ((player ammo _ArmaSecundaria) > 0) then { _ArmaSecAmmo = (secondaryWeaponMagazine player) select 0; }; }; _ArmaPistola = handgunWeapon player; //PISTOLA _ArmaPisAcc = handgunItems player; //ACCESORIOS DE LA PISTOLA _ArmaPisAmmo = []; if (_ArmaPistola != "") then { if ((player ammo _ArmaPistola) > 0) then { _classMag = (handgunMagazine player) select 0; _countMag = player ammo _ArmaPistola; _ArmaPisAmmo = [_classMag,_countMag] ; }; }; _Gadjets = assignedItems player; //GADJETS _Casco = headgear player; //ACCESORIOS DE LA CABEZA _Gafas = goggles player; //GAFAS EQUIPO = [_Ropa, _ContenidoRopa, _Chaleco, _ContenidoChaleco, _Mochila, _ContenidoMochila, _ArmaPrincipal, _ArmaPriAmmo, _ArmaPriAcc, _ArmaSecundaria, _ArmaSecAmmo, _ArmaSecAcc, _ArmaPistola, _ArmaPisAmmo, _ArmaPisAcc, _Gadjets, _Casco, _Gafas ]; if (DINERO > 0) then { //Suelto la pasta... _PosDinero = _Soldado modelToWorld [((random -0.2)+(random 0.2)),1,((random -0.2)+(random 0.2))]; [[_PosDinero,DINERO],"CAOE_fnc_createDineroMuerte",false,true] call BIS_fnc_MP; }; //Reseteo la pasta... DINERO = 100;
and script called when "respawn" EH:
_Soldado = [_this,0] call BIS_fnc_param; _Cuerpo = [_this,1] call BIS_fnc_param; 12452 cutText ["","BLACK OUT",0.01]; _Soldado playActionNow "PlayerProne"; //Vuelvo a ejecutar la restriccion de zona _Null = ["mkZonaCombate",_Soldado,15] spawn CAOE_fnc_RestriccionZonaCombate; //pasa a ser Renegado (para el Friendly fire) _Soldado addRating -10000; _Ropa = EQUIPO select 0; //UNIFORME _ContenidoRopa = EQUIPO select 1; //CONTENIDO DEL UNIFORME _Chaleco = EQUIPO select 2; //CHALECO _ContenidoChaleco = EQUIPO select 3; //CONTENIDO DEL CHALECO _Mochila = EQUIPO select 4; //MOCHILA _ContenidoMochila = EQUIPO select 5; //CONTENIDO DE LA MOCHILA _ArmaPrincipal = EQUIPO select 6; //ARMA PRINCIPAL _ArmaPriAmmo = EQUIPO select 7; //ARMA PRINCIPAL AMMO _ArmaPriAcc = EQUIPO select 8; //ACCESORIOS DEL ARMA PRINCIPAL _ArmaSecundaria = EQUIPO select 9; //ARMA SECUNDARIA _ArmaSecAmmo = EQUIPO select 10; // _ArmaSecAcc = EQUIPO select 11; //ACCESORIOS DEL ARMA SECUNDARIA _ArmaPistola = EQUIPO select 12; //PISTOLA _ArmaPisAmmo = EQUIPO select 13; _ArmaPisAcc = EQUIPO select 14; //ACCESORIOS DE LA PISTOLA _Gadjets = EQUIPO select 15; //GADJETS _Casco = EQUIPO select 16; //ACCESORIOS DE LA CABEZA _Gafas = EQUIPO select 17; //GAFAS _Soldado enableFatigue (!("me_FFv4" in MEJORAS)); if (_ArmaSecundaria != "") then { if (_ArmaSecAmmo != "") then { _Soldado addBackpack "B_HuntingBackpack"; _Soldado addMagazine _ArmaSecAmmo; _Soldado addWeapon _ArmaSecundaria; removeBackpack _Soldado; } else { _Soldado addWeapon _ArmaSecundaria; }; {if (_x != "") then {_Soldado addSecondaryWeaponItem _x;};}forEach _ArmaSecAcc; }; if (_Ropa != "") then { if(!(_Soldado isUniformAllowed _Ropa)) then { _Soldado forceAddUniform _Ropa; } else { _Soldado addUniform _Ropa; }; {_Soldado addItemToUniform _x;}forEach _ContenidoRopa; } else { removeUniform _Soldado; }; if (_Chaleco != "") then { _Soldado addVest _Chaleco; {_Soldado addItemToVest _x;}forEach _ContenidoChaleco; } else { removeVest _Soldado; }; if (_Mochila != "") then { _Soldado addBackpack _Mochila; {_Soldado addItemToBackpack _x;}forEach _ContenidoMochila; } else { removeBackpack _Soldado; }; if (_ArmaPrincipal != "") then { _Soldado addWeapon _ArmaPrincipal; {if (_x != "") then {_Soldado addPrimaryWeaponItem _x;};}forEach _ArmaPriAcc; if ((count _ArmaPriAmmo) > 0) then { _Soldado addMagazine (_ArmaPriAmmo select 0); _Soldado setAmmo [_ArmaPrincipal,_ArmaPriAmmo select 1]; }; }; if (_ArmaPistola != "") then { _Soldado addWeapon _ArmaPistola; {if (_x != "") then {_Soldado addHandgunItem _x;};}forEach _ArmaPisAcc; if ((count _ArmaPisAmmo) > 0) then { _Soldado addMagazine (_ArmaPisAmmo select 0); _Soldado setAmmo [_ArmaPistola,_ArmaPisAmmo select 1]; }; }; if ((count _Gadjets) > 0) then { { _Soldado linkItem _x; }forEach _Gadjets; }; if (_Casco != "") then { _Soldado addHeadgear _Casco; } else { removeHeadgear _Soldado; }; if (_Gafas != "") then { _Soldado addGoggles _Gafas; } else { removeGoggles _Soldado; }; sleep 1; 12452 cutText ["","BLACK IN",3]; if ((count RESPAWNPOS) > 1) then { _Soldado setDir (RESPAWNDIR + 180); _Soldado setPosATL RESPAWNPOS; }else{ _posAleatorio = [["mkZonaDrop"]] call BIS_fnc_randomPos; _posicionLibre = _posAleatorio findEmptyPosition [1,30,"B_soldier_PG_F"]; _Soldado setPosATL _posicionLibre; _Soldado addAction ["PLANTAR TIENDA RESPAWN","Scripts\TRespawn_Plantar.sqf","",1]; };
_____________________________________________________________________________________________________________________
[PROB2] Sometimes the gun dealer do not appear:
init.sqf (at the end of SERVER SETUP section)
sleep 0.1; startLoadingScreen ["ZzZzZzZZzzzz..."]; //********************************************************* //**************** PARAMETROS ********************* //********************************************************* DMLS_ParamDuracion = paramsArray select 1; DMLS_ParamNumSuministros = paramsArray select 2; DMLS_ParamNumDinero = paramsArray select 3; DMLS_ParamSatelite = paramsArray select 4; DMLS_ParamAtaqueIA = paramsArray select 5; DMLS_ParamDineroInicial = paramsArray select 6; DMLS_ParamPrecio = paramsArray select 7; DMLS_ParamBancoPersistente = paramsArray select 8; DMLS_ParamHora = paramsArray select 10; DMLS_ParamClima = paramsArray select 11; DMLS_ParamDistancia = paramsArray select 12; DMLS_ParamDistanciaObj = paramsArray select 13; DMLS_ParamCalidad = paramsArray select 14; DMLS_Param3PVista = paramsArray select 15; //********************************************************* //************* VARIABLES PUBLICAS ****************** //********************************************************* //ESPECIFICAS PARA CADA JUGADOR if (!isDedicated) then { waitUntil {!(isNull player)}; DINERO = DMLS_ParamDineroInicial; DINERO_BANCO = [] call CAOE_fnc_DineroEnBanco; EQUIPO = []; LOGISTICA = []; MEJORAS = []; RESPAWNPOS = []; RESPAWNDIR = 0; player setVariable ["VEHICULOS",[],true]; player setVariable ["MUERTES", 0, true]; //CONTROL DEL FINAL "DMLS_GANADORES" addPublicVariableEventHandler { _ArrayGanadores = [_this,1] call BIS_fnc_param; cutText ["","BLACK OUT",2]; player EnableSimulation false; player AllowDamage false; PlayMusic "Track05_Underwater2"; 0 = [_ArrayGanadores] Spawn { SetTerrainGrid 6.25; _Ganadores = [_this,0] call BIS_fnc_param; _textGanadores = "GANADOR"; _soyGanador = false; if ((count _Ganadores)>1 ) then { _textGanadores = _textGanadores + "ES";}; { if (_x == player) then {_soyGanador = true;}; _Null =["<t>" + _textGanadores + "</t><br/><t size='0.6'>CON </t><t color='#FF0000' size='0.6'>"+ Format ["%1",(_x getVariable "MUERTES")] + "</t><t size='0.6'> MUERTES</t><br/><br/><t color='#DDFF00'>" + Format ["%1",Name _x] + "</t>",0,-0.3,12,2] spawn BIS_fnc_dynamicText; sleep 2; cutText ["","BLACK IN",1]; _Cam = "Camera" CamCreate (_x modelToWorld [0,5,1]); _Cam CameraEffect ["External", "Back"]; _Cam camSetTarget _x; _Cam camSetRelPos [0,1,1.5]; _Cam camsetFOV 0.5; _Cam camCommit 8; Sleep 10; }forEach _Ganadores; ["end1",(_soyGanador)] call BIS_fnc_endMission; }; }; }; //LIMITES DE TIEMPO TIEMPO_Limite = DMLS_ParamDuracion; TIEMPO_Transcurrido = 0; TIEMPO_ConFormato = ""; //********************************************************* //********* FUNCIONES PARA EL MP ******************* //********************************************************* fnc_DevolverDineroBanco = {DINERO_BANCO;}; //********************************************************* //*************** PARTIDA SETUP ********************** //********************************************************* enableSaving [false, false]; //RADIO OFF enableSentences false; 0 fadeRadio 0; //HORA setDate [2014,5,7,DMLS_ParamHora,0]; //DISTANCIAS DE VISION setViewDistance DMLS_ParamDistancia; setObjectViewDistance DMLS_ParamDistanciaObj; //CLIMA skipTime -24; 86400 setOvercast (DMLS_ParamClima / 100); skipTime 24; if(DMLS_ParamClima >= 60) then { 60 setRain (DMLS_ParamClima / 100); 60 setWindForce (DMLS_ParamClima /200); //Establezco la fuerza del viento 60 setWindStr (DMLS_ParamClima /200); }; 0 = [] spawn { sleep 0.1; simulWeatherSync;}; //CALIDAD DEL TERRENO setTerrainGrid DMLS_ParamCalidad; //********************************************************* //*************** SERVER SETUP ********************** //********************************************************* if (isDedicated) then { pVarRelampago = false; //WEST setFriend [WEST, 0]; // ---------------------------------------------- // --------- Cronometro del server ---------- // ---------------------------------------------- [] spawn { TIEMPO_Inicial = diag_tickTime; while {TIEMPO_Transcurrido < TIEMPO_Limite} do { TIEMPO_Transcurrido = diag_tickTime - TIEMPO_Inicial; sleep 1; publicVariable "TIEMPO_Transcurrido"; }; // Uso un publicVariableEH para controlar el final, al acabarse el tiempo busco el jugador/es // con mas muertes y se lo paso a la variable que activa el EH en todas las maquinas. _MuertesMax = 0; _ArrayGanadores = []; _Jugadores = [] call BIS_fnc_listPlayers; { _Muertes = _x getVariable "MUERTES"; switch (true) Do { case (_Muertes == _MuertesMax): { _ArrayGanadores = _ArrayGanadores + [_x]; }; case (_Muertes > _MuertesMax): { _MuertesMax = _Muertes; _ArrayGanadores = [_x]; }; }; }forEach _Jugadores; missionNamespace setVariable ["DMLS_GANADORES", _ArrayGanadores]; publicVariable "DMLS_GANADORES"; }; [] spawn { private ["_tiempo","_tiempoFinal_Minutos","_tiempoFinal_Segundos"]; while{TIEMPO_Transcurrido < TIEMPO_Limite} do { _tiempo = TIEMPO_Limite - TIEMPO_Transcurrido; _tiempoFinal_Minutos = floor(_tiempo / 60); _tiempoFinal_Segundos = floor(_tiempo) - (60 * _tiempoFinal_Minutos); if(_tiempoFinal_Segundos < 10) then { _tiempoFinal_Segundos = format ["0%1", _tiempoFinal_Segundos];}; if(_tiempoFinal_Minutos < 10) then { _tiempoFinal_Minutos = format ["0%1", _tiempoFinal_Minutos];}; TIEMPO_ConFormato = format ["%1:%2", _tiempoFinal_Minutos, _tiempoFinal_Segundos]; publicVariable "TIEMPO_ConFormato"; sleep 1; }; }; // ----------------------------------------------- // ------------ Satelite espia ------------ // ----------------------------------------------- if (DMLS_ParamSatelite != 1000) then { [] spawn { private ["_tiempo","_tiempoFinal_Minutos","_tiempoFinal_Segundos"]; _tiempoEspia = DMLS_ParamSatelite; while{TIEMPO_Transcurrido < TIEMPO_Limite} do { if (TIEMPO_Transcurrido >= _tiempoEspia) then { _Null = [] call CAOE_fnc_SateliteEspia; _tiempoEspia = _tiempoEspia + DMLS_ParamSatelite; }; }; }; }; // ------------------------------------------------ // --------- Lanzador de suministros --------- // ------------------------------------------------ [] spawn { private ["_tiempoProvIntervalo","_tiempoProvisiones","_bLanzamiento","_bLanzamientoAlt"]; _tiempoProvIntervalo = TIEMPO_Limite / (DMLS_ParamNumSuministros + 1); for "_i" from 1 to DMLS_ParamNumSuministros do { _tiempoProvisiones = _tiempoProvIntervalo * _i; waitUntil {(TIEMPO_Transcurrido >= _tiempoProvisiones)}; _bLanzamiento = ["mkZonaDrop"] call CAOE_fnc_LanzarSuministros; if (!_bLanzamiento) then { _bLanzamientoAlt = ["mkZonaDrop"] call CAOE_fnc_LanzarSuministros; }; }; }; // ---------------------------------------------- // --------- Lanzador de Dinero ---------- // ---------------------------------------------- [] spawn { private ["_tiempoProvIntervalo","_tiempoProvisiones","_bLanzamiento","_bLanzamientoAlt"]; _tiempoProvIntervalo = TIEMPO_Limite / (DMLS_ParamNumDinero + 1); for "_i" from 1 to DMLS_ParamNumDinero do { _tiempoProvisiones = _tiempoProvIntervalo * _i; waitUntil {(TIEMPO_Transcurrido >= _tiempoProvisiones)}; _bLanzamiento = ["mkZonaDrop"] call CAOE_fnc_LanzarDinero; if (!_bLanzamiento) then { _bLanzamientoAlt = ["mkZonaDrop"] call CAOE_fnc_LanzarDinero; }; }; }; //CARGO OBJETOS PARA EL INICIO DEL SERVER _Null = execVM "Scripts\iniciarServidor.sqf"; }; endLoadingScreen; //********************************************************* //*************** PLAYER SETUP ********************** //********************************************************* if (isMultiplayer && hasInterface) then { 12452 cutText [".: PARTIDA CARGADA :.\n\n· USA EL [ORDENADOR] PARA ESCOJER TU EQUIPACION.\n· USA EL [MAPA] PARA SALTAR A LA ZONA COMBATE.\n\n\n " + Format ["CAERAN %1 SUMINISTROS DURANTE PARTIDA",DMLS_ParamNumSuministros]+ "\n\n" + Format ["%1 TRANSPORTES DE DINERO PASARAN CERCA",DMLS_ParamNumDinero], "BLACK FADED", 50000]; player addRating -10000; player allowDamage false; sleep 9; 12452 cutText ["", "PLAIN"]; player addEventHandler ["Killed", {_this execVM "Scripts\EH_Killed.sqf"}]; player addEventHandler ["Respawn", {_this execVM "Scripts\EH_Respawn.sqf"}]; _null = []execVM "HUD\mostrarHUD_Jugador.sqf"; };
iniciarServidor.sqf
private ["_Iniciado", "_idx", "_jugador", "_Contenedor", "_Mesa", "_Ordenador", "_Mapa", "_Luz","_Silla","_posicion","_Vendedor","_iniTienda","_MostradorA","_MostradorB"]; //Uso una variable publica para indicar cuando el proceso de carga ha terminado //Oculto los marcadores.. "mkZonaDrop" setMarkerAlpha 0; "inicioIA_1" setMarkerAlpha 0; "inicioIA_2" setMarkerAlpha 0; "inicioIA_3" setMarkerAlpha 0; "inicioIA_4" setMarkerAlpha 0; "inicioIA_5" setMarkerAlpha 0; "inicioIA_6" setMarkerAlpha 0; "inicioIA_7" setMarkerAlpha 0; // Creo los objetos necesarios para el inicio For "_idx" from 1 to 10 do { _Contenedor = objNull; _Mesa = objNull; _Ordenador = objNull; _Mapa = objNull; _Luz = objNull; _Silla = objNull; //Creo un contenedor en la posicion del marcador "mkInicial_x" y bloqueo las puertas _Contenedor = createVehicle ["Land_Cargo20_light_green_F",(getMarkerPos (Format ["mkInicial_%1",_idx])),[],0,"CAN_COLLIDE"]; _Contenedor enableSimulationGlobal false; _Contenedor setVariable ['bis_disabled_Door_1',1,true]; _Contenedor setVariable ['bis_disabled_Door_2',1,true]; _Mesa = createVehicle ["Land_CampingTable_F",(_Contenedor modelToWorld [0,0,0]),[],0,"CAN_COLLIDE"]; _Ordenador = createVehicle ["Land_Laptop_device_F",(_Contenedor modelToWorld [0,0,0]),[],0,"CAN_COLLIDE"]; _Luz = createVehicle ["Land_Camping_Light_F",(_Contenedor modelToWorld [0,0,0]),[],0,"CAN_COLLIDE"]; _Silla = createVehicle ["Land_CampingChair_V2_F",(_Contenedor modelToWorld [0,0,0]),[],0,"CAN_COLLIDE"]; _Mapa = createVehicle ["Land_Map_altis_F",(_Contenedor modelToWorld [2.95,0,0.4]),[],0,"CAN_COLLIDE"]; _Mapa setVectorDir [0,-1,0]; _Mapa setVectorUp [-1,0,0]; _Mapa enableSimulationGlobal false; _Mesa enableSimulationGlobal false; _Ordenador enableSimulationGlobal false; _Luz enableSimulationGlobal false; _Silla enableSimulationGlobal false; [_Contenedor,_Mesa,[-2.35,0,0.175],90] call BIS_fnc_relPosObject; [_Contenedor,_Ordenador,[-2.35,-0.55,0.975],80] call BIS_fnc_relPosObject; [_Contenedor,_Luz,[-2.4,0.6,0.975],0] call BIS_fnc_relPosObject; [_Contenedor,_Silla,[-1.5,0.55,0.2],90] call BIS_fnc_relPosObject; //Añado las acciones pertinentes en MP [[_Ordenador,"<t color='#FFFF00'>COMPRAR EQUIPO</t>","Scripts\act_Tienda.sqf",0],"CAOE_fnc_AddAction",nil,true] call BIS_fnc_MP; [[_Mapa,"<t color='#F0A804'>SALTAR A LA ZONA DE COMBATE</t>","Scripts\act_ParacaSaltar.sqf","mkZonaDrop"],"CAOE_fnc_AddAction",nil,true] call BIS_fnc_MP; }; //Creo las tiendas y los cajeros For "_idx" from 1 to 2 do { _posicion = getMarkerPos (Format ["mkTienda_%1",_idx]); _Vendedor = createVehicle ["C_man_hunter_1_F",_posicion,[],0,"CAN_COLLIDE"]; _Vendedor setPosATL _posicion; _Vendedor setDir (markerDir (Format ["mkTienda_%1",_idx])); [[_Vendedor,"BRIEFING"], "BIS_fnc_ambientAnim", nil, true] call BIS_fnc_MP; [[_Vendedor,"<t color='#FFFF00'>COMPRAR</t>","Scripts\act_Tienda.sqf",1,3],"CAOE_fnc_AddAction",nil,true] call BIS_fnc_MP; _Vendedor allowDamage false; //CAJEROS _posicion = getMarkerPos (Format ["mkATM_%1",_idx]); _Cajero = createVehicle ["Land_Atm_01_F",_posicion,[],0,"CAN_COLLIDE"]; _Cajero enableSimulationGlobal false; _Cajero setDir (markerDir (Format ["mkATM_%1",_idx])); [[_Cajero,"<t color='#FFFF00'>INTRODUCIR TARJETA..</t>","Scripts\act_Cajero.sqf"],"CAOE_fnc_AddAction",nil,true] call BIS_fnc_MP; };
_____________________________________________________________________________________________________________________
[PROB3] When you kill inside a vehicle killCount is not stored:
i use a setVariable command with public parameter on true to set the kills count on "killed" EH
"killed" EH script involving the problem:
_Soldado = [_this,0] call BIS_fnc_param; _Asesino = [_this,1] call BIS_fnc_param; if ((_Asesino != _Soldado) && !(isNull _Asesino)) then { _MuertesAsesino = _Asesino getVariable ["MUERTES",-1]; }; if (_MuertesAsesino != -1) then { _MuertesAsesino = _MuertesAsesino + 1; _Asesino setVariable ["MUERTES", _MuertesAsesino, true]; } else { hint Format ["NO EXISTE LA VARIABLE EN %1",_Asesino]; }; };
Fixed?
_Soldado = [_this,0] call BIS_fnc_param; _Asesino = [_this,1] call BIS_fnc_param; if ((_Asesino != _Soldado) && !(isNull _Asesino)) then { if (!((vehicle _Asesino) isKindOf "man")) then { _Asesino = (crew _Asesino) select 0; }; _MuertesAsesino = _Asesino getVariable ["MUERTES",-1]; if (_MuertesAsesino != -1) then { _MuertesAsesino = _MuertesAsesino + 1; _Asesino setVariable ["MUERTES", _MuertesAsesino, true]; } else { hint Format ["NO EXISTE LA VARIABLE EN %1",_Asesino]; }; };
-
yes, the only solution is
_Soldier disableAI "AUTOTARGET";
and you specify the target with the radio
---------- Post added at 07:16 ---------- Previous post was at 07:11 ----------
but in your case you can use Civilians:
Civilian setFriend [WEST,0]; WEST setFriend [Civilian ,0]; Civilian setFriend [EAST,0]; EAST setFriend [Civilian ,0]; ...
use addWeapon to the Civs, or addUniform, etc...
-
yes, i need to know too..
my mission is a DM, with recruit option..
in my init.sqf i put this line to avoid Friendly fire:
WEST setFriend [WEST,0];
and on player Init and player Respawn to make renegades (ENEMY):
player addRating -10000;
in my tests can't do it..
_Soldier = (group player) createUnit ["B_Soldier_F", player modelToWorld [0,4,0],[],0,"FORM"]; _Soldier addRating (rating player); _Soldier joinAsSilent [(group player),2] _Soldier disableAI "AUTOTARGET";
i think the path to do that is with FSM..
-
this execVM "clearInventory.sqf";
u need a handle
null = this execVM "clearInventory.sqf";
---------------------
and need parentesis
if (!local _unit || _unit getVariable ["loadoutDone", FALSE]) exitWith {};
if (!(local _unidad) || (_unidad getVariable ["loadoutDone", FALSE])) exitWith {};
and maybe replace _unit with player (in my tests, when player disconnect and reconnect, soldier still have loadoutDone = true because (i think) when you assign _unit = _this; takes the name of the unit not the player..)
sorry by my bad english
-
-
hi beaksby.
first, markers dont have altitude, are 2D. createMarker
you can explain what you need to do?
i've writed a supply drop script.. maybe can help you
/* ------------------------------------------------------------------------------ FUNCION: fnc_LanzarSuministros ------------------------------------------------------------------------------ DESC: Make a heli and drop a supplyBox with parachute on a random position inside an area. call on server only ------------------------------------------------------------------------------ PARAMETROS: 0: [sTRING] Area Marker name (marker need to have circular) 1: [NUMERO] (Opcional) How far heli has created in meters 2: [NUMERO] (Opcional) Altitude ------------------------------------------------------------------------------ EJEMPLO: - Place a marker with 50m area and name it "mkZonaCombate" ["mkZonaCombate", 2000, 100] call fnc_LanzarSuministros; ------------------------------------------------------------------------------ */ private ["_mkZonaLanzamiento","_Distancia","_Altura","_posIniAleatoria","_posicionInicial","_posicionDrop","_posicionSimetrica","_grp","_HeliDrop","_wpSupply","_wpExit","_Suministros","_paraca","_nombreMarcador","_Marcador","_Completado"]; _mkZonaLanzamiento = [_this,0,"",[""]] call BIS_fnc_param; _Distancia = [_this,1,1200,[0]] call BIS_fnc_param; _Altura = [_this,2,120,[0]] call BIS_fnc_param; _Completado = false; _posIniAleatoria = [(getMarkerPos _mkZonaLanzamiento), _Distancia, (floor (random 360))] call BIS_fnc_relPos; _posicionInicial = [(_posIniAleatoria select 0),(_posIniAleatoria select 1),(_Altura + 30)]; _posicionDrop = [[_mkZonaLanzamiento]] call BIS_fnc_randomPos; _posicionSimetrica = [((_posicionDrop select 0) * 2) - (_posicionInicial select 0),((_posicionDrop select 1) * 2) - (_posicionInicial select 1), (_Altura + 30)]; _grp = createGroup WEST; _HeliDrop = [_posicionInicial, ([_posicionInicial, _posicionDrop] call BIS_fnc_dirTo), "I_Heli_Transport_02_F", _grp] call BIS_fnc_spawnVehicle; _wpSupply = _grp AddWaypoint [_posicionDrop,0]; _wpExit = _grp AddWaypoint [_posicionSimetrica,0]; _wpSupply setWaypointType "MOVE"; _wpExit setWaypointType "MOVE"; (_HeliDrop select 0) flyInHeight _Altura; waitUntil {((currentWaypoint _grp) == 2) || (!canMove(_HeliDrop select 0))}; if (canMove(_HeliDrop select 0)) then { _HeliPos = getPosATL (_HeliDrop select 0); _paraca = createVehicle ["B_Parachute_02_F", [_HeliPos select 0,_HeliPos select 1,(_HeliPos select 2)-8], [], 0, "CAN_COLLIDE"]; _Suministros = createVehicle ["B_supplyCrate_F", [_HeliPos select 0,_HeliPos select 1,(_HeliPos select 2)-10], [], 0, "CAN_COLLIDE"]; _Suministros attachTo [_paraca,[0,0,0]]; _Suministros allowDamage false; clearMagazineCargoGlobal _Suministros; clearWeaponCargoGlobal _Suministros; clearItemCargoGlobal _Suministros; clearBackpackCargoGlobal _Suministros; _Suministros addMagazineCargoGlobal ["30Rnd_65x39_caseless_green",50]; _Suministros addMagazineCargoGlobal ["30Rnd_65x39_caseless_mag",50]; _Suministros addMagazineCargoGlobal ["30Rnd_556x45_Stanag",20]; _Suministros addMagazineCargoGlobal ["100Rnd_65x39_caseless_mag",10]; _Suministros addMagazineCargoGlobal ["150Rnd_762x51_Box",5]; _Suministros addMagazineCargoGlobal ["20Rnd_762x51_Mag",20]; _Suministros addMagazineCargoGlobal ["10Rnd_762x51_Mag",20]; _Suministros addMagazineCargoGlobal ["7Rnd_408_Mag",15]; _Suministros addMagazineCargoGlobal ["5Rnd_127x108_Mag",15]; _Suministros addMagazineCargoGlobal ["5Rnd_127x108_APDS_Mag",15]; _Suministros addMagazineCargoGlobal ["200Rnd_65x39_cased_Box",15]; _Suministros addMagazineCargoGlobal ["RPG32_F",5]; _Suministros addMagazineCargoGlobal ["RPG32_HE_F",5]; _Suministros addMagazineCargoGlobal ["NLAW_F",5]; _Suministros addMagazineCargoGlobal ["Titan_AA",5]; _Suministros addMagazineCargoGlobal ["Titan_AP",5]; _Suministros addMagazineCargoGlobal ["3Rnd_UGL_FlareWhite_F",5]; _Suministros addMagazineCargoGlobal ["UGL_FlareWhite_F",5]; _Suministros addMagazineCargoGlobal ["HandGrenade",15]; _Suministros addMagazineCargoGlobal ["MiniGrenade",15]; _Suministros addMagazineCargoGlobal ["SmokeShell",15]; _Suministros addMagazineCargoGlobal ["APERSMine_Range_Mag",10]; _Suministros addMagazineCargoGlobal ["APERSBoundingMine_Range_Mag",10]; _Suministros addMagazineCargoGlobal ["APERSTripMine_Wire_Mag",10]; _Suministros addMagazineCargoGlobal ["SLAMDirectionalMine_Wire_Mag",5]; _Suministros addItemCargoGlobal ["optic_Arco",5]; _Suministros addItemCargoGlobal ["optic_Hamr",5]; _Suministros addItemCargoGlobal ["optic_MRCO",5]; _Suministros addItemCargoGlobal ["optic_DMS",5]; _Suministros addItemCargoGlobal ["optic_LRPS",5]; _Suministros addItemCargoGlobal ["FirstAidKit", 2]; _Suministros addItemCargoGlobal ["Rangefinder", 1]; _Suministros addItemCargoGlobal ["muzzle_snds_H", 1]; _Suministros addItemCargoGlobal ["muzzle_snds_H_MG", 1]; _Suministros addItemCargoGlobal ["muzzle_snds_M", 1]; _Suministros addItemCargoGlobal ["muzzle_snds_B", 1]; if !(isNull _paraca) then { waitUntil {isNull _paraca}; deleteVehicle _paraca; // _Suministros allowDamage true; }; _nombreMarcador = Format ["mk%1Supply%2", str(floor(random 500)),str(floor(random 500))]; _Marcador = createMarker [_nombreMarcador,(getPosATL (_Suministros))]; _Marcador setMarkerShape "ICON"; _Marcador setMarkerType "b_unknown"; _Marcador setMarkerText "Suministros"; _Marcador setMarkerAlpha 1; waitUntil {((currentWaypoint _grp) == 3) || (!canMove(_HeliDrop select 0))}; {deleteVehicle _x} forEach crew (_HeliDrop select 0); waitUntil {(count crew (_HeliDrop select 0)) == 0}; deleteVehicle (_HeliDrop select 0); _Completado = true; } else { _aviso = parseText "<t size='2' color='#FF0000' align='center' underline='true'>ATENCION</t><br/><br/><t size='1' color='#FFFFFF'>SUMINISTROS INTERCEPTADOS</t><br/><t color='C0C0C0'>SE INTENTARA MANDAR OTRO</t>"; [_aviso,"fnc_Hint",true,false] call BIS_fnc_MP; sleep 10; {deleteVehicle _x} forEach crew (_HeliDrop select 0); waitUntil {(count crew (_HeliDrop select 0)) == 0}; deleteVehicle (_HeliDrop select 0); }; _Completado;
-
hi Iceman77, thankyou very much! i try to explain it.
I have a UI Dialog (shop) with two lists. When you buy a item, i need to ask to the player something using a Yes/No dialog, but in some cases i need more options to ask (Yes/No/Add to...) so i try to make a custom msgBox dialog with no success..
Anyway i will send my mission as soon as possible
-
Aargh .. I have not found the solution yet
I will use "BIS_fnc_guiMessage" instead..
-
thanks DreadedEntity. i take look to that.. but when i call "FirstDialog" i use this:
pasedTo_AddActionScript.sqf
createDialog "FirstDialog"; waitUntil {dialog}; 0 = [] spawn { private ["_comboCtrl","_listCtrl","_treeCtrl"]; disableSerialization; _comboCtrl = (findDisplay 4000 displayCtrl 4001); _listCtrl = (findDisplay 4000 displayCtrl 4002); _treeCtrl = (findDisplay 4000 displayCtrl 4021); ... ... };
inside _listCtrl i have a onLBDblClick = "[_this] call CAOE_fnc_ConfigureThings;" ctrlEventHandler.
And inside fn_ConfigureThings.sqf i call the msgBox with another call
structure:
[font=Courier New] pasedTo_AddActionScript.sqf [color="#008000"]* First call using Spawn[/color] 0 = Spawn [ [color="#008000"] * 2ond call using CtrlEventHandler (function called is defined in description.ext)[/color] call CAOE_fnc_ConfigureThings [ [color="#008000"] * 3th call using a function defined in desc.ext too[/color] call CAOE_fnc_MsgBox [ [color="#FF0000"]waitUntil Error[/color] ] } ] [/font]
is a scheduled env?
-
Hi, im trying to do a messageBox Dialog control.
I have one dialog called "FirstDialog" and i need to ask something to the player inside "FirstDialog"..
so i make another dialog called "MsgBox_Dialog". (maybe this is the problem.. dont know)
Both are defined in description.ext like
class "FirstDialog" { class controls { blah, blah }; }; class MsgBox_Dialog { idd = 5000; duration = 7200; onLoad = ""; controls[]= { DMLS_MsgBox_Frame, DMLS_MsgBox_Fondo, DMLS_MsgBox_Pregunta, DMLS_MsgBox_Btn0, DMLS_MsgBox_Btn1, DMLS_MsgBox_Btn2 }; class DMLS_MsgBox_Frame: IGUIBack { idc = 5010; x = 0.293752 * safezoneW + safezoneX; y = 0.357047 * safezoneH + safezoneY; w = 0.412495 * safezoneW; h = 0.263914 * safezoneH; }; class DMLS_MsgBox_Fondo: RscFrame { idc = 5011; x = 0.293752 * safezoneW + safezoneX; y = 0.357047 * safezoneH + safezoneY; w = 0.412495 * safezoneW; h = 0.263914 * safezoneH; }; class DMLS_MsgBox_Pregunta: RscStructuredText { idc = 5001; x = 0.304065 * safezoneW + safezoneX; y = 0.37904 * safezoneH + safezoneY; w = 0.39187 * safezoneW; h = 0.175943 * safezoneH; }; class DMLS_MsgBox_Btn0: RscButton { idc = 5020; x = 0.613436 * safezoneW + safezoneX; y = 0.565978 * safezoneH + safezoneY; w = 0.082499 * safezoneW; h = 0.0439856 * safezoneH; }; class DMLS_MsgBox_Btn1: RscButton { idc = 5021; x = 0.510312 * safezoneW + safezoneX; y = 0.565978 * safezoneH + safezoneY; w = 0.082499 * safezoneW; h = 0.0439856 * safezoneH; }; class DMLS_MsgBox_Btn2: RscButton { idc = 5022; x = 0.304065 * safezoneW + safezoneX; y = 0.565978 * safezoneH + safezoneY; w = 0.185623 * safezoneW; h = 0.0439856 * safezoneH; }; };
i make a function to call it:
class CfgFunctions { class CAOE { class logic { class MsgBox {}; }; }; };
CAOE_fnc_MsgBox.sqf
/* ------------------------------------------------------------------------------ FUNCION: CAOE_fnc_MsgBox ------------------------------------------------------------------------------ DESCRIPCION: Crea un msgBox ------------------------------------------------------------------------------ PARAMETROS: 0: [sTRING] structured text - question 1: [sTRING] Button 1 2: [sTRING] (Optional) Button 2 3: [sTRING] (Optional) Button 3 ------------------------------------------------------------------------------ RETURN: [sTRING] Button selected ------------------------------------------------------------------------------- EXAMPLE: _Msg = "<t size='2' color='#FF0000' align='center' underline='true'>ATENTION</t><br/><t size='1.25' color='#FFFFFF'>You are a code master?</t>"; _Return = [_Msg,"Yes","No"] call CAOE_fnc_MsgBox; ------------------------------------------------------------------------------ */ private["_Contenido","_Btn0","_Btn1","_Btn2","_Retorna"]; _Contenido =[_this,0,"",[""]] call BIS_fnc_param; _Btn0 = [_this,1,"",[""]] call BIS_fnc_param; _Btn1 = [_this,2,"",[""]] call BIS_fnc_param; _Btn2 = [_this,3,"",[""]] call BIS_fnc_param; _msgBoxDialog = createDialog "MsgBox_Dialog"; waitUntil {_msgBoxDialog}; _TextCtrl = (findDisplay 5000 displayCtrl 5001); _Btn0Ctrl = (findDisplay 5000 displayCtrl 5020); _Btn1Ctrl = (findDisplay 5000 displayCtrl 5021); _Btn2Ctrl = (findDisplay 5000 displayCtrl 5022); _TextCtrl ctrlSetStructuredText parseText _Contenido; uiNamespace setVariable ["msgBox","-1"]; switch (true) Do { case (_Btn0 != "" && _Btn1 == "" && _Btn2 == ""): { _Btn0Ctrl ctrlSetText _Btn0; _Btn0Ctrl ctrlAddEventHandler ["onMouseButtonClick",{uiNamespace setVariable ["msgBox",_Btn0]}]; //TODO: I need to close dialog when choose an option _Btn1Ctrl ctrlShow false; _Btn2Ctrl ctrlShow false; }; case (_Btn0 != "" && _Btn1 != "" && _Btn2 == ""): { _Btn0Ctrl ctrlSetText _Btn0; _Btn0Ctrl ctrlAddEventHandler ["onMouseButtonClick",{uiNamespace setVariable ["msgBox",_Btn0]}]; //TODO: I need to close the dialog when choose an option _Btn1Ctrl ctrlSetText _Btn1; _Btn1Ctrl ctrlAddEventHandler ["onMouseButtonClick",{uiNamespace setVariable ["msgBox",_Btn1]}]; //TODO: I need to close the dialog when choose an option _Btn2Ctrl ctrlShow false; }; case (_Btn0 != "" && _Btn1 != "" && _Btn2 != ""): { _Btn0Ctrl ctrlSetText _Btn0; _Btn0Ctrl ctrlAddEventHandler ["onMouseButtonClick",{uiNamespace setVariable ["msgBox",_Btn0]}]; //TODO: I need to close the dialog when choose an option _Btn1Ctrl ctrlSetText _Btn1; _Btn1Ctrl ctrlAddEventHandler ["onMouseButtonClick",{uiNamespace setVariable ["msgBox",_Btn1]}]; //TODO: I need to close the dialog when choose an option _Btn2Ctrl ctrlSetText _Btn2; _Btn2Ctrl ctrlAddEventHandler ["onMouseButtonClick",{uiNamespace setVariable ["msgBox",_Btn2]}]; //TODO: I need to close the dialog when choose an option }; }; waitUntil {!dialog}; //ERROR ON THIS LINE _Retorna = uiNamespace getVariable ("msgBox"); _Retorna;
and finally i call the function:
_Msg = "<t size='2' color='#FF0000' align='center' underline='true'>ATENTION</t><br/><t size='1.25' color='#FFFFFF'>You are a code master?</t>"; _return = [_Msg,"Yes","No"] call CAOE_fnc_MsgBox; if (_return == "Yes") then { hint str("I'm a codemaster"); } else { hint str("I need help"); };
The problem is the waitUntil command at the end of CAOE_fnc_MsgBox.sqf.. doesn't work and i don't know why.
Is there any way to do this?
or any template to use?
or something?
thanks!!
-
hurts my feelings :pjejej sorry!! not my intention, i love old school
I really like your function, I appreciate the contribution
Any way to get player's new body when Respawn without EH?
in ARMA 3 - MISSION EDITING & SCRIPTING
Posted · Edited by KoVValsky
if you have a _player variable (i.e. _player = player) and player is killed, _player becomes a nullObject when respawn..
Is there any way to get player's new body without Event Handlers or Event Scripts?
thankyou!
----------------------------------------------------
SOLUTION FOUND:
----------------------------------------------------
if you assign a name to the player, you can ask to that name with this:
if you want's a player object variable:
----------------------------------------------------
i.e. if you have a player named "Soldier1" in the editor
call (compileFinal "Soldier1") is equal to Soldier1 so:
it's the same as:
this prevents objNull on respawn when you have assigned a unit to a variable