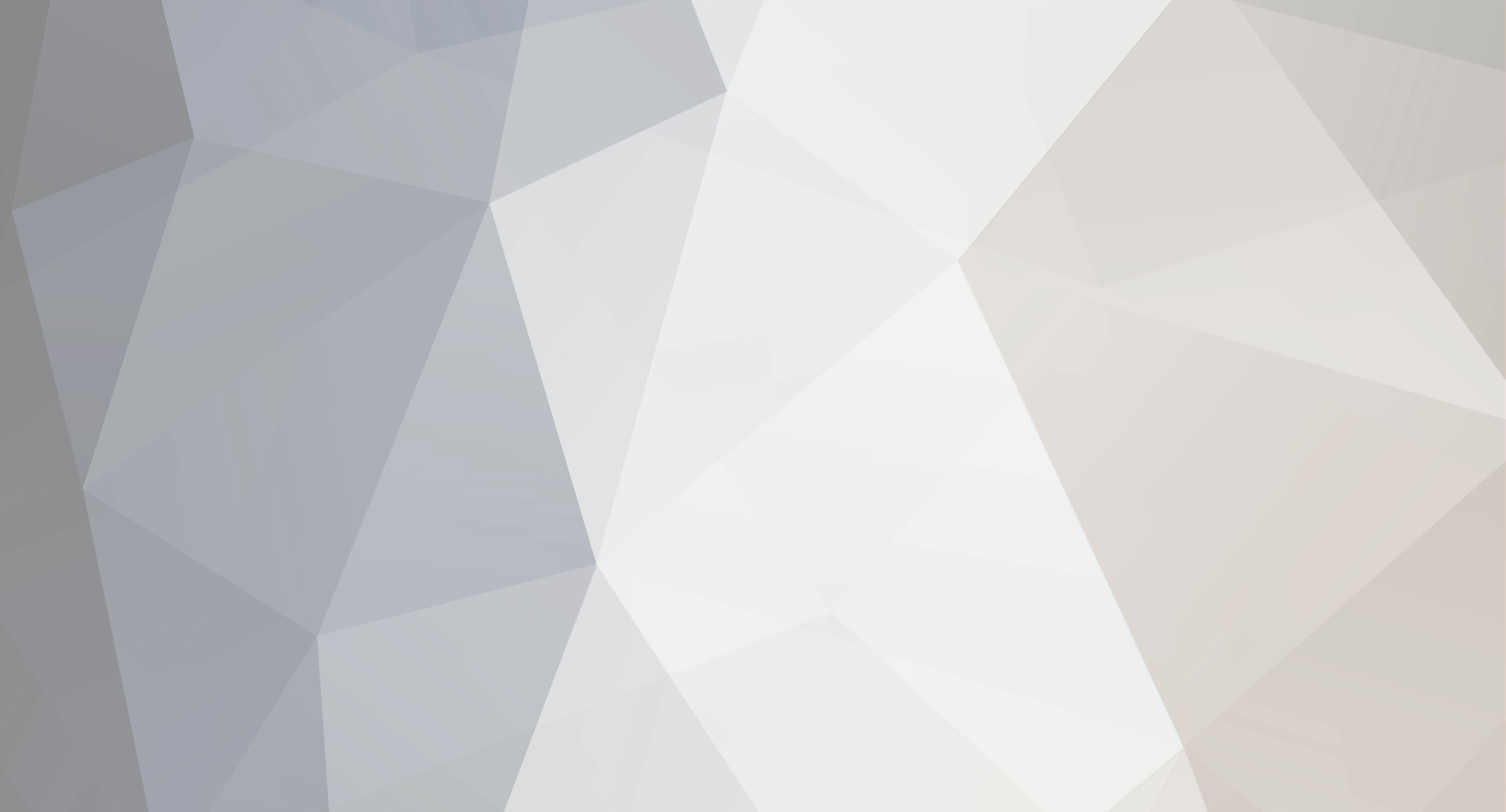

Rommel
-
Content Count
1225 -
Joined
-
Last visited
-
Medals
Posts posted by Rommel
-
-
Multiplayer 29 NOV 09 - There were more, but alas casualties of war (and cameraman), front guy is AI VIP (rescued)
Addons: Aardvarks SASR (1.2) || AAW Steyrs || RAF Chinook
-
Have you made the following markers: respawn_vehicle_west or respawn_vehicle_east
-
Is the functions module placed?
-
The ingame gravity is 9.8m/s (Tested over and over). However there is ENORMOUS air resistance on a lot of things; whilst there is no terminal velocities.
-
Couldn't see the harm in the delay, as long as it doesn't prioritise over other things.
-
And how do you spot them?When a state can't exit because all conditions out won't become true.
I found a few deadlocks, but am interested to see if they coincide with Xeno's.
-
Xeno, what was the deadlock condition in that script? Apart from the deadlock when the unit dies.
-
That is called the overview picture. Open up a BIS mission to figure it out. This is a volunteer help forum mate, don't expect anything, especially with that attitude.
-
That should work.
-
Excellent. FSM's cause negative FPS drop... :D
FYI: Fincuan, your script is exactly why I am asking this question.
-
The reason it doesn't work is because #define is a pre-processor command; ie before the game itself truly compiles the code.
For example:
#define MYVAR "test" if ("test" == MYVAR) exitwith {};
The game engine during compilation see's this as:
if ("test" == "test") exitwith {};
Therefore, with your code:
#define MYDEFINE "it works" call compile preprocessFileLineNumbers "fnc.sqf"; sleep 3; call fnc1;
Becomes:
call compile preprocessFileLineNumbers "fnc.sqf"; sleep 3; call fnc1;
The code is then executed by the game engine, and becomes the following:
call {fnc1 = {hint str(MYDEFINE)}}; sleep 3; call {hint str(MYDEFINE)};
The compiler has already made its pre-processor run through, long before this, so therefore MYDEFINE will not work.
Try the following instead:
init.sqf
#define MYDEFINE "it works" MYDEFINE call compile preprocessFileLineNumbers "fnc.sqf"; sleep 3; call fnc1;
fnc.sqf
fnc1 = compile format ["hint %1", _this];
Therefore:
call fnc1 == call {hint "it works"};
-
Can we have performance differences from BIS? If there is indeed an advantage, I'd like to know. :confused:
-
_i = _a find 1; while {_i != -1} do { _a set [_i, "null"]; _a = _a - ["null"]; _i = _a find 1; };
Really...
@Dissaifer
Minimal coding, if you use a something that has been processed twice, store it into memory.
So instead of:
_man = (units group _this) select 0; _man1 = (units group _this) select 1;
Do:
_units = units (group _this); _man1 = _units select 0; _man2 = _units select 1;
Any constants should be defined and not stored into memory.
So instead of:
_distance = 30; _b = _distance * _this; _c = _distance / _this;
Do:
#define DISTANCE 30 _b = DISTANCE * _this; _c = DISTANCE / _this;
If you have a function, or a piece of code you execute multiple times, compile it and save it to a variable; saves constant compilation by the engine.
So instead of:
{ if (alive _x) then { _pos = getpos _x; _pos set [2, 50]; _pos set [1, 20]; _pos set [0, (_pos select 0) - 2]; _x setpos _pos; }; } foreach (units _group);
Do:
_fPos = { _pos = getpos _this; _pos set [2, 50]; _pos set [1, 20]; _pos set [0, (_pos select 0) - 2]; _this setpos _pos; }; { if (alive _x) then {_x call _fPos}; } foreach (units _group);
Obviously in that situation, it seems a bit stupid, but it can be very handy later and save on repeating lines of code over and over. Saves compilation too.
-
Perhaps check the FAQ, or read the previous posts. Its not hard.. and you could have saved us all some time.
-
Sorry. I expected people to use common sense from there on.
_a = [1,2,3]; _i = _a find 1; _a set [_i, "REMOVETHISCRAP"]; _a = _a - ["REMOVETHISCRAP"];
_a == [2,3]
To resize:
_a = [2,3]; _a resize 1; _a = [2];
-
Check the FAQ.
http://forums.bistudio.com/showthread.php?t=73424
If you have any questions, you should ask them there rather than make a new thread.
-
As said above.
Also, good practice:
(units group this)
-
Please use the FAQ. It has this answer 10 fold over.
-
...I just needed to know there is no other way than looping through the array...I just posted it above you. Use the find command.
-
It takes me 15s to change over the version and have it loading again. It doesn't take that long?
-
_a = [1,2,3]; _i = _a find 1; _a set [_i, 0]; hint format ["%1 and %2", _a, [0,2,3]];
Self explanatory that _a has the first element changed to a 0 and is then displayed.
-
What is RMM_recordPos
A time saver. It records the players positions to an array, which can then (via trigger) be copied to your clipboard for easy output.
Why would you use RMM_recordPos
You can spend hours trying to get the perfect position for things in the editor, or even the 3D editor, but a hands on approach is still the best way when placing units in the 2D editor.
How to use RMM_recordPos
Use the action in the players menu 'record position', to add a position to the array, this is done via RMM_record.sqf.
To copy the array to the clipboard, use 0-0-1
To copy the current player position to the clipboard, use 0-0-2
To reset the array, use 0-0-3.
Hopefully this helps save time, I know it has for me.
http://www.4shared.com/file/147105212/8a6023b3/RMM_RecordPosChernarus.html
(fyi: attachments don't work; error received was 'cannot access uploads path')
-
in HALO.sqs there is :BIS_HALO_unit_dir = 0
BIS_HALO_unit_speed = 50
BIS_HALO_turnR = 0
How can i do to have the unit dir of the player when he was in the plane and not North direction ( 0 ) ???
Maybe vectorDir? Otherwise I don't think it is possible.
-
I wouldn't recommend this if you using lots of groups, whilst it is slightly less laggy than having the groups themselves, the script checks are very heavy on CPU.
As for it not working, I'll be releasing my own pack soon, just wait a few days.
Convoy Manager
in ARMA 2 & OA - ADDONS & MODS: COMPLETE
Posted · Edited by Rommel
For your convoy manager, may I suggest you use an equation instead of all the switches? In Pseudo code (PM me for my own script)