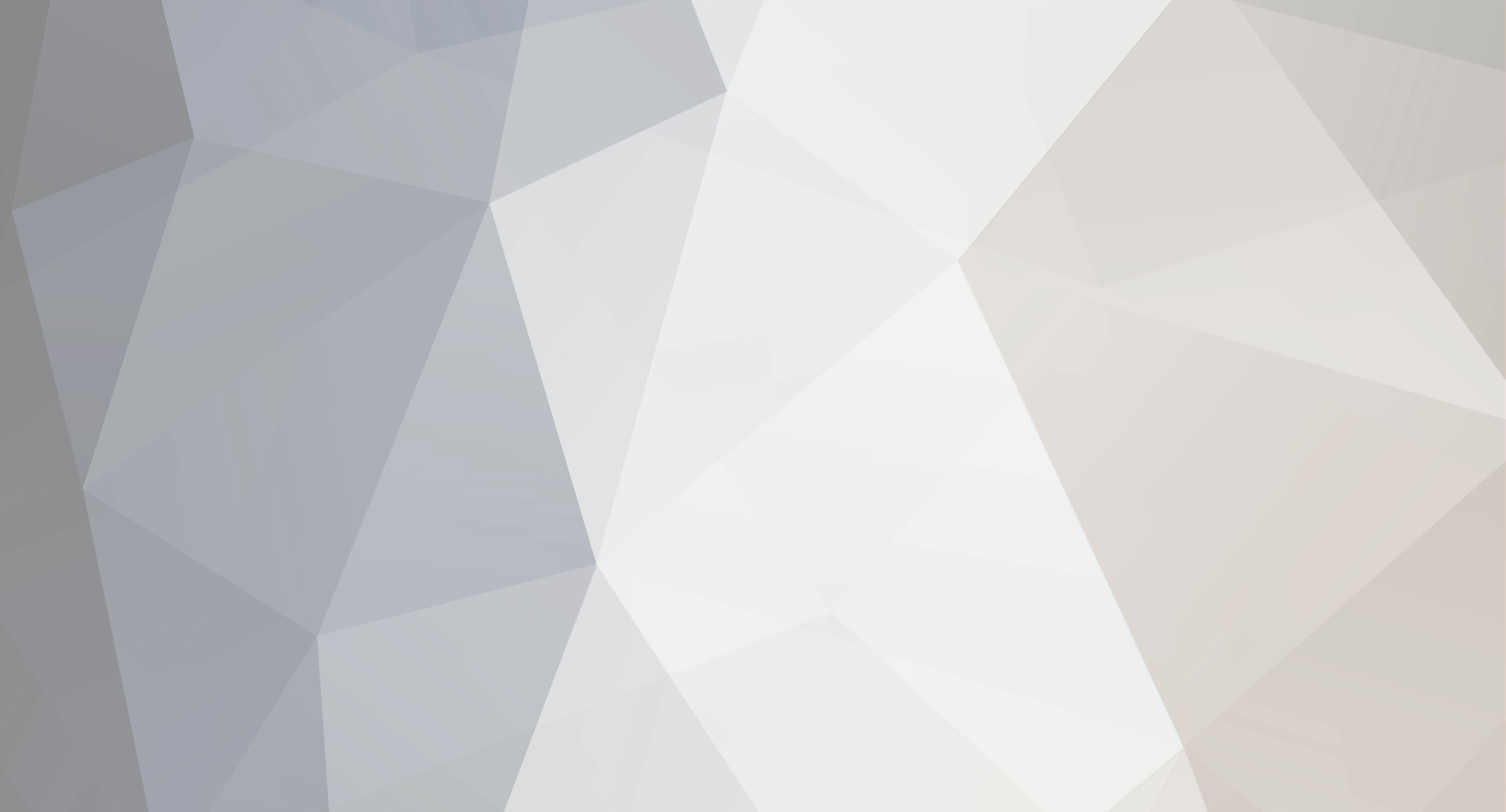

tracy_t
-
Content Count
330 -
Joined
-
Last visited
-
Medals
Posts posted by tracy_t
-
-
The init event handler performs one-time initialisation of a unit.
Insert it into your addon like so:
class Zombie1: Civilian1
{ Â Â Â
    class eventhandlers
   {
      init = [_this select 0] exec {\scottsaddon\initzombie.sqs};
   };
};
In the above example, a script called initzombie.sqs is called to initialise the zombie unit. Note the use of "_this" instead of "this" - the underscore is important.
Replace scottsaddon with the name of your pbo file (minus pbo extension of course)
-
-
HOW TO CREATE YOUR OWN ADDON
By Scott "Tracy" Tunstall
Before you read this, it helps if you have programmed in an object oriented language so you understand the concepts of inheritance and polymorphism.
The config scripting language appears to be a subset of C++, but does not AFAIK allow multiple inheritance of classes.
Anyway, the first thing to do when creating a new addon is to copy the #defines below into the top of your new addon's config.cpp. They make the code a lot more readable.
Then, Create your CfgPatches class. If the word "class" frightens you then I recommend you look up C++ classes (from msdn.microsoft.com, for example)
CfgPatches is used by OFP to determine what addons are to be added to the addon database, and what version of OFP is required in order to run them.
The contained class ScottT_Boat holds the important information:
The units[] array is a comma separated list that specifies the CLASS NAMES of the units we want to add to the OFP database. We only have one custom unit, and that is defined in class SuperFastBoat (nerd info: usually I would prefix the class with capital C, as per Microsoft C++ practices, but I haven't here. Do as I say, not as I do lol)
The requiredversion variable states that only operation flashpoint version 1.75 (ie: Resistance) and over can use this addon.
Next, you need to create a CfgVehicles class. CfgVehicles is, again, a standard OFP class, and is responsible for containing the implementation details of your custom addons. You MUST NOT leave CfgVehicles as an empty class!
Now, this is where it gets fun. Look at class All{}. To the uninitiated, class All looks like an "empty" class containing no data. However, this is wrong. When you declare class All in this way, you are telling the OFP config parser to IMPORT the standard operation Flashpoint "class All" data from BIS's own config.cpp.
We can see that class AllVehicles inherits from class All. Without class All{} you cannot have a class AllVehicles{} - try to declare class AllVehicles{} without class All{} and you'll crash to desktop pretty fast. Again, we want AllVehicles to be imported from BIS's config.cpp, hence the {} "empty implementation".
Now, if you have not done so already, download OFP: RES 1.90 config.cpp from
http://web.quick.cz/fholesinsky/OF/config190.zip
You now need to identify what vehicle you want to "subclass" - subclassing means, "to take an existing class and derive a new class from it". In my case, I wanted to tweak the Mark II PBR ship.
In config190.cpp you can see the Mark II PBR ship is defined in class BoatW. (The DisplayName variable gives it away.)
To make a new version of BoatW, not only do you have to derive a class from BoatW, but you have to import all the "super classes" (I don't like using object oriented tech speak, but it's for the best). The super classes basically are all the classes that BoatW inherits from.
In Config190.cpp, we can see that BoatW inherits from class SmallShip. In turn, SmallShip inherits from Ship, and Ship inherits from AllVehicles.
So I import the existing BIS Ship, SmallShip and BoatW classes into my config.cpp like so:
<table border="0" align="center" width="95%" cellpadding="0" cellspacing="0"><tr><td>Code Sample </td></tr><tr><td id="CODE">
class Ship: AllVehicles {};
class SmallShip: Ship {};
class BoatW: SmallShip {};
(Note that the order of class declaration is very important. Â Try moving class Ship to the bottom of the list - again, OFP will quit back to desktop!)
OK, now I have imported the Mark 2 PBR into my project, I want to make my new super-ship.
I create a new ship like so:
<table border="0" align="center" width="95%" cellpadding="0" cellspacing="0"><tr><td>Code Sample </td></tr><tr><td id="CODE">
class SuperFastBoat: BoatW
and this time, because I want to make my boat act differently from BoatW, I add some implementation details to class Superfastboat.
<table border="0" align="center" width="95%" cellpadding="0" cellspacing="0"><tr><td>Code Sample </td></tr><tr><td id="CODE">
{
    scope = public;   // So this appears in OFP editor
    vehicleclass = "Superfast Armoured Ships";
    side = TWest;    // Make ship West
    // Maxspeed of 100 = 85kph, 300 = 110 kph, 500 = superfast!
    maxspeed = 500;   // Higher number = more speed
}
The scope variable determines how this new unit will appear to the OFP editor. (Note: the scope #defines are misleading. The Public, Protected and Private declarations have nothing to do with C++ inheritance specifiers!!!)
Public - seen in easy & advanced mission editor mode
Protected - seen only in advanced editor mode
Private - the class is only to be used as a "base" class, and cannot be used in the editor at all.
VehicleClass is the TYPE of the unit that will appear in the mission editor unit type Drop Down List. This name will not appear in game. You can call it "Scott's special boat" for all I care
DisplayName is the NAME of the unit that will appear on the mission editor map, and in game.
Side can be a number from 0-7.
East = 0
West = 1
Guerrila (resistance) = 2
Civilian = 3
Unknown = 4 (don't use this)
Enemy = 5 (don't use this)
Friendly = 6 (don't use this)
Logic = 7 (can't see a use for this, lol)
OK, the last thing I need to do for my super fast boat is to make it super fast!
Therefore, all I do is change the maxspeed variable from 60
(as it is set in BoatW class - see config190.cpp) to something mad like 500.
Now, I check that all the braces and semicolons are in the correct place, then I save my new config.cpp to a new folder, cunningly called SuperFastBoat. I use MakePBO utility to convert the contents of the directory into a .PBO file, and put it in Res\Addons.
Et voila! A new boat under West | SuperFast Armoured Ships.
<table border="0" align="center" width="95%" cellpadding="0" cellspacing="0"><tr><td>Code Sample </td></tr><tr><td id="CODE">
#define TEast 0
#define TWest 1
#define TGuerrila 2
#define TCivilian 3
#define TSideUnknown 4
#define TEnemy 5
#define TFriendly 6
#define TLogic 7
#define true 1
#define false 0
// type scope
#define private 0
#define protected 1
#define public 2
class CfgPatches
{
    class ScottT_Boat
    {
    units[]={SuperFastBoat};
    requiredversion=1.750000;  // version of OFP you need
    };
};
class CfgVehicles
{
class All {};
class AllVehicles: All {};
  class Ship: AllVehicles {};
  class SmallShip: Ship {};
  class BoatW: SmallShip {};
  class SuperFastBoat : BoatW
  {
    scope = public;   // So this appears in OFP editor
    vehicleclass = "Superfast Armoured Ships";
    displayName = "Fast Armoured Mark2 PBR";
    side = TWest;    // Make ship West
    // Maxspeed of 100 = 85kph, 300 = 110 kph, 500 = superfast!
    maxspeed = 500;   // Higher number = more speed
  }
}
-
Ach to Hell with that!!!
Just download:
www.freewebs.com/tunstals/superfastboat.pbo
and that does the trick!!!
A superfast Mark 2 PBR for you, and what's more, it's done in a single config.cpp
I thank you
(tunstals = me. My name is Scott Tunstall.)
-
Hi Prototype,
Heh - after we get the dawn of the dead guys in, all we'll need are a city full of zombies - then the fun can begin!
-
Hi Merc, check in addons and mods: discussion for the answer.
Also folks, MORE ANNOUNCEMENTS!!!
Scott
-
Oh yeah, while I'm at it:
ANNOUNCEMENT:
EVEN MORE ZOMBIES ON THE WAY!!!
Yes, before you've even had a chance to make your first zombie mission, I'm complicating the issue entirely by releasing a new set of ghouls. Well, why the hell not eh?
Anyway, in the forthcoming release:
* SOUNDS - Death rattle, moaning, chomping
* NEW ANIMATIONS - Staggering etc. These animations will be integrated into the addon itself, you will not need to animate the zombies yourself.
SECONDLY:
Do YOU need music for your zombie missions?
Get theme music from:
http://www.zombiejunkyard.com/movie_info_pics/
Regards,
Scott
-
DOWNLOAD A SAMPLE MISSION AT:-
http://www.freewebs.com/tunstals/killemall.noe.zip
YOU NEED TO PASTE THIS URL INTO THE ADDRESS BAR OF YOUR BROWSER.
Right
First things first:
To animate:
put in your script
zombie playmove <animation>
where <animation> is walkvar1, walkvar2, walkvar3. In the 2nd addon pack, you have extra walkvar4 and eat1 animations.
2. To make your zombie attack west + resistance soldiers, create two triggers:
a) Name the 1st trigger WESTSOLDIERS, activated by WEST, ONCE
b) Name the 2nd trigger RESISTANCESOLDIERS, activated by RESISTANCE, ONCE
Both triggers should cover your entire island.
Then, copy the large block of code below into a text file called movezombie.sqs. Put the .sqs file into your mission's directory.
Finally, go back into the flashpoint editor and select a zombie. Double click to bring up the zombie's properties.
In the zombie's initialisation field, enter:
<table border="0" align="center" width="95%" cellpadding="0" cellspacing="0"><tr><td>Code Sample </td></tr><tr><td id="CODE">
[this] exec "movezombie.sqs"
This will make your zombie attack the nearest west or resistance soldier.
movezombie.sqs
<table border="0" align="center" width="95%" cellpadding="0" cellspacing="0"><tr><td>Code Sample </td></tr><tr><td id="CODE">
_z = _this Select 0
_t = _this Select 1
#Main
~1
?(!alive _z) : goto "End"
?(_t != objNULL) && (alive _t) goto "move"
_tig = false
_t = player
_pt = (list resistancesoldiers) + (list westsoldiers)
_y = 0
_uc = count _pt
#loop
~2
? _y>= _uc: goto "premove"
_u = _pt select _y
? (!alive _u) : goto "nextunit"
? _z distance _u < _z distance _t: _t = _u
#nextunit
_y=_y+1
goto "loop"
#premove
? _t in units group player: _tig = true
_z dowatch _t
#move
_d = _z distance _t
? _d > 1000 : Goto "end"
? _d > 5 : goto "walk"
? _d < 6 : goto "engage"
Goto "Main"
#walk
_z domove getpos _t
~2
goto "Main"
#engage
? !alive _z: goto "end"
_z removeMagazines "StrokeFist"
_z setPos getpos _t
_z addMagazine "StrokeFist"
_z fire "StrokeFist"
~3
_t setDammage (getDammage _t + 0.2)
~1
goto "Main"
#End
~5
DeleteVehicle _z
exit
-
Oh Christ, I live in the South side of Glasgow!!! LOL!!!!
So I should be able to do that better than most - know? (if the last word was over your head, it's Glasgow speak OK. lol)
-
MrZig: the resistance equivalent to blackop is not spetsnaz lol - that's EAST
Anyway
1) It's easy enough to mod the boat. You don't need to edit any models or whatnot, just create a small config.cpp file and change the maxspeed variable (I forget exactly what it's called but I have the 1.90 config.cpp reference to hand so I can find it easily). I can help you with this if you wish.
2) For creating a resistance blackop, do the same as 1, mod the WEST blackop except change the side to resistance (again, a rather easy process.)
If you want me to help, just PM me and I'll sort something out with ya.
Scott
-
Thanks again Bratty. Will do.
Scott.
-
Prototype,
I can make the zombies die only with head shots, but that will make the game useless with AI soldiers: they only target the body. End result is lots of AI soldiers being killed!
Besides, I am making super tough zombies for the addon, with significantly improved "armour"
If you change the hitpoints, does that mean the soldiers can shoot at the head?
-
Hello.
I created a CfgMovesMC class with various walking animations.
this PlayMove blabla
and
Switchmove blabla
call the animations OK, but I don't want to use them in any script. I want operation flashpoint to use my animations directly, called from the game engine itself.
Any idea on how I do that?
Help would be much appreciated.
EDIT: Even if you can point me a URL that tells me how to hook other standard OFP animations, I could work out the rest from there
TIA
Scott
-
Hmm,
what's the armor member variable set to in the config.cpp?
When I was writing my zombie addon, I set the armor of the zombie to 40.0.
Soldiers would not engage the zombie- they would say "negative" too 0- until I lowered the armor to 4.
Try lowering the armour?
-
You ain't seen, or should I say heard, nothing yet!
I have just bought a high quality microphone, and am now gonna start recording some zombie sounds. As I'm no squeaky voiced teenager, but a fully grown man (in some depts more than others
) I can assure you these sounds will enhance the atmosphere no end. Zombies with a Scotch accent? That'll rule ;)
Ain't it strange, that every zombie movie plays music when a horde of ravenous zombies are on screen? I used to think that it was for atmosphere, but now I believe its to stop the moaning sounds being sampled!!
In Dawn of the dead for example, there's hardly any zombie scenes without some background music. Even that shitty carnival music plays when the zombies are in the mall.
HAVING SAID THAT, if you find any really good music-less sound samples of zombies (male/ female) moaning and heavy breathing (no, not from THOSE kind of movies lol) would you find it in your heart to send me them?
TIA
Tracy
-
Prototype:
go to www.rayza.net - there are loads of trashed car pictures etc on the dawn remake link.
-
Hi there,
I would like to incorporate a custom walk animation for one of my units.
I do not want to use PlayMove or SwitchMove to activate it though, I want to override the standard "walk forward" animation so that the Flashpoint engine uses my animation instead of the default one.
So far, any classes I have made doesn't work.
Can anyone help, my brain hurts.
-
That's right... I was looking for the George Romero newspaper (which Resident evil blatantly ripped off) but couldn't find it.
No matter... GET INTO THOSE ZOMBIES!!!
-
CRAWLER AND HEADLESS ZOMBIE *FINAL* RELEASED!!!
Crawler has Dr Tongue's face.
Go to:
-
ZOMBIES ARE RELEASED ON SHELL SHOCKS PAGE!!!!
THEY'RE HERE.......
-
When I try to connect to freewebs, it works fine.
If you still have problems, email me and I'll send you the addons myself.
-
ANNOUNCEMENT: CRAWLING ZOMBIE *FINAL* SHOULD BE AVAILABLE FOR DOWNLOAD IN THE NEXT 5-10 MINUTES. PLEASE DOWNLOAD FROM
-
Timing issues with animations are now fixed. Everything is hunky dory in the tracy_t garden.
Meaning, I'm spending even more time on producing MORE zombies. I will release, in the next couple of hours, the CRAWLER zombie - it has no legs. And it rocks!
I will post when it is released; get the original addon from shellshocks page:
http://www.freewebs.com/shell_shocks_workshop/
Regards,
Scott
-
Erm,
I can't see me using it in my missions
Too cartoon like for my tastes!
But what do other people think?
Speed up boats?
in OFP : MISSION EDITING & SCRIPTING
Posted
Glad you liked it!!! Let me know if u use it in any of your missions