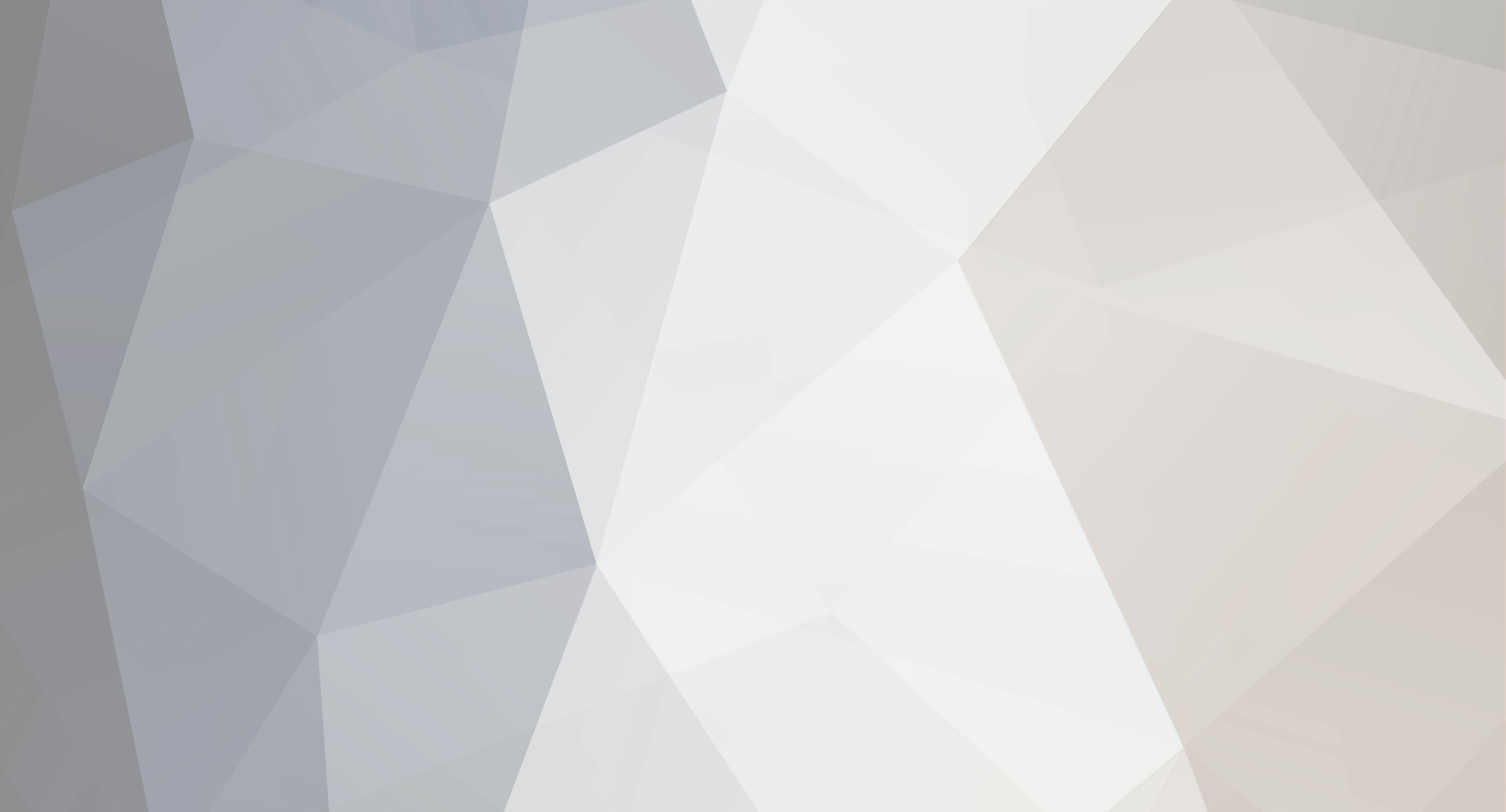

dwringer
-
Content Count
368 -
Joined
-
Last visited
-
Medals
Posts posted by dwringer
-
-
making an empty huey with just two pilots should still work, though. If they are ignoring a "Transport Unload" waypoint try putting "this assignAsDriver helo1;" in one of their inits, and "this assignAsCargo helo1;" in the other.
-
Hrm. I was almost certain the inconsistencies had been mostly worked out. There is just one thing that I neglected to mention - you MUST create a unit of side east in the editor for this script to work. If you do not, you have to do some extra steps to create the opfor group (stuff with which i'm not very familiar. it's much easier to just drop some random opfor guy somewhere hidden and far away.)
Also, keep in mind the way it is now, the civilians will all meander back to a point where they will regroup prior to running to the armWaypoint. Thus, it may seem to not be working, but eventually you should see the civs do their thing. I have been able to get it to function consistently using all three current versions of the scripts in my post above, although like with any other technique getting things to "make sense" in a mission is never quite so simple without a lot of trial and error to balance things.
So yeah, basically my only advice could be, make sure you have created at least SOME unit of side OPFOR in the editor before trying to use these scripts. Otherwise it will NOT work without properly setting up an OPFOR hq (something I don't really know how to do off the top of my head).:confused:
EDIT: As for the ALICE stuff, yeah, I can never get anything cool to work with that. Even giving them event handlers to tally how many i kill was something I never accomplished even after hours of trying (and fully developing scripts to do it with civs I placed manually). I am pretty sure some people have gotten that part working, not that I have seen a fleshed out working example of it, but I try not to use ambient civilians for that and myriad other reasons of convenience when it comes to having this level of control over their behaviour.
-
Oh man, would be a good troll if you hadn't hotlinked such a huge image. That's taking things too far.
-
Javascript, ugh, no, don't learn programming with that. Haha. Once you have a firm grasp on the basic operations and techniques of something like Java, which will get you pretty far in knowing your way around ArmA scripting, I recommend looking into something like Python or Lisp. I've been learning Common Lisp for the past few months and coming back to ArmA scripting with that background is like an entirely different world. Java will certainly teach you the basics of instances and objects, but Lisp can give you insights into how to look at programming tasks in a more abstract and universal way that can be applied with any programming language. Then you start to realize that .sqf is a ridiculously powerful tool. Or if you ever looked at Mando's MMA code I guess it was self-evident, not that mortals can grasp it to that degree ;p
-
Sorry, that script I posted before was pretty buggy and not very smart on my part. Since then I've worked it out to be a lot more detailed; here are the results:
First, rebel.sqf
_grpLdr = _this select 0; _wpnCache = _this select 1; _grpThis = group _grpLdr; _mgznMen = """10x_303"""; _wpnMen = """LeeEnfield"""; _mgznLdr = """75Rnd_545x39_RPK"""; _wpnLdr = """RPK_74"""; {_x allowFleeing 0;} forEach units _grpThis; {_x setVariable ["hasRebelled", true];} forEach units _grpThis; sleep (5 + (random 10)); _grpNew = createGroup (side _grpLdr); _wptRegroup = _grpNew addWaypoint [waypointPosition [_grpThis, 0], 0]; _wptArm = _grpNew addWaypoint [position _wpnCache, 0]; _wptDummy = _grpNew addWaypoint [position _wpnCache, 5]; _wptRegroup setWaypointType "MOVE"; _wptRegroup setWaypointBehaviour "SAFE"; _wptArm setWaypointCompletionRadius 2.5; _wptArm setWaypointType "MOVE"; _wptArm setWaypointBehaviour "STEALTH"; _wptArm setWaypointStatements ["true", format ["_grpOPFOR = createGroup east; _wptSecure = _grpOPFOR addWaypoint [position this, 2.5]; _wptSecure setWaypointCombatMode ""RED""; _wptSecure setWaypointBehaviour ""COMBAT""; _wptSecure setWaypointType ""SAD""; _grpOPFOR setCurrentWaypoint _wptSecure; (units group this) joinSilent _grpOPFOR; {_dude = _x; {_dude addMagazine %1;} forEach [1,2,3,4]; _x addWeapon %2;} forEach ((units _grpOPFOR) - [leader _grpOPFOR]); {(leader _grpOPFOR) addMagazine %3;} forEach [1,2,3,4]; (leader _grpOPFOR) addWeapon %4;", _mgznMen, _wpnMen, _mgznLdr, _wpnLdr]]; _wptDummy setWaypointType "SAD"; _wptDummy setWaypointCombatMode "RED"; {[_x] join _grpNew} forEach units (group _grpLdr); _grpNew setCurrentWaypoint _wptRegroup;
If you want, you can keep the civs in one area giving them a "DISMISS" waypoint to start and using "wrangle.sqf" in their groupleaders' init lines.
wrangle.sqf:
_man = _this select 0; _distMax = _this select 1; _distRet = _distMax * 0.85; _posStart = getPos _man; _man setVariable ["hasRebelled", false]; while {!(_man getVariable "hasRebelled")} do { _compX = abs ((position _man select 0) - (_posStart select 0)); _compY = abs ((position _man select 1) - (_posStart select 1)); _distCurr = (sqrt ((_compX * _compX) + (_compY * _compY))); if (_distCurr >= _distMax) then { _grpNew = createGroup (side _man); _wptReturn = _grpNew addWaypoint [_posStart, 0]; _wptDismiss = _grpNew addWaypoint [waypointPosition _wptReturn, 0]; _wptReturn setWaypointType "MOVE"; _wptReturn setWaypointSpeed "LIMITED"; _wptDismiss setWaypointType "DISMISS"; _wptDismiss setWaypointSpeed "NORMAL"; _wptReturn setWaypointCompletionRadius _distRet; _wptDismiss setWaypointCompletionRadius _distRet; _grpNew setCurrentWaypoint _wptReturn; {[_x] join _grpNew} forEach units (group _man); }; sleep (4 + (random 2)); };
Now to call you can make a trigger (with whatever conditions you want to spawn the ambush), and in its on-act put something like:
{nul = _x execVM "rebel.sqf"} forEach [[civReb, wpc_0_1], [civReb_1, wpc_0_1], [civReb_2, wpc_2_3], [civReb_3, wpc_2_3], [civReb_4, wpc_4]];
That would be assuming you have three caches, wpc_0_1, wpc_2_3, and wpc_4, serving the civilian groups referenced by number (civReb . . . civReb_4).
Then in each civReb leader's init box put
nul = [this, 125] execVM "wrangle.sqf";
(Where "125" would get replaced with whatever distance you want them to stay within).
Problems: Every time the civilians get farther than _distMax from their starting dismissed location under the effects of "wrangle.sqf", they ALWAYS return to their initial position before wandering again. Cannot for the life of me find away around this, because when the dismissal is cancelled (by reassigning them to a newly created group), they always return to that group's waypointPosition for the dismiss waypoint.
They're not particularly smart when they become hostile at first, perhaps scripting in some knowsAbout thisList stuff from the trigger would help that.
Finally, my script just gives all the civilian leaders an rpk with 4 mags, and the followers a lee enfield apiece with 4 mags. You can change this however you want, even make a switch statement or add more parameters to the script call. I left the definitions of these weapons/magazines as local variables declared at the top of the script, so you can do with them as you will.
PS Make certain you use the double quotes when they show up. They do that to tell the compiler not to break out of evaluating the string passed to format, and to interpret them as literal "'s. That goes also for the triple quotes at the top, being a string representation of the above :P
PPS As far as I can tell , you MUST set civilians to be friendly to 'everyone', or at the very least to 'opfor' in the editor. If you don't, they will not do what they're supposed to in any predictable fashion.
EDIT: Here's a way to use a list of civilians and a list of weapon caches to randomize which civilians rebel, and to which caches they run to arm themselves. It's very primitive, and there are tons of ways this could be made smarter or integrated into a broader mission setup. Instead of calling the "rebel.sqf" script yourself, just use your trigger to call "ambush.sqf". Then edit ambush.sqf to contain the list of your civilians and your weapon caches in the first two lines.
Ambush.sqf:
_civsAvail = [civ, civ_1, civ_2, civ_3, civ_4, civ_5]; _wpcsAvail = [wpc, wpc_1, wpc_2]; _chanceAmbush = 0.4; _parmArr = []; _xWpcFound = false; _countWpcs = count _wpcsAvail; _xWpc = nil; {if (_x getVariable "hasRebelled") then {_civsAvail = _civsAvail - [_x];}} forEach _civsAvail; {if ((random 1) < _chanceAmbush) then { while {!_xWpcFound} do { {if ((random _countWpcs) < 1) then { _xWpcFound = true; _xWpc = _x;}} forEach _wpcsAvail;}; _parmArr = _parmArr + [[_x, _xWpc]]; _xWpcFound = false;};} forEach _civsAvail; {_x execVM "rebel.sqf"} forEach _parmArr;
You can also set the chance any given group will rebel, by default here it is a 40% chance.
-
As we know,
There are known knowns.
There are things we know we know.
We also know
There are known unknowns.
That is to say
We know there are some things
We do not know.
But there are also unknown unknowns,
The ones we don't know
We don't know.
-
Hey all. I've read several informative posts on the subject after doing some searches, but I still have just a simple question about switching units out of a "DISMISS" waypoint to carry out further orders.
I saw that other forum-goers have found that a "Switch" trigger does not automatically switch units out of a "dismissed" waypoint unless said units detect hostiles. Once they have, they do appear to switch on to the next waypoint.
Whether or not this is true (I often rely on this working and am not usually let down, so IDK), there is one instance in which it does appear to function improperly.
I wrote a simple script to get units, once they move a certain distance from the point at which the script was spawned, to move back to a position within the predefined radius of their starting position. The only way I got this to function was by having the units either rejoin their current group, or join a newly created group (there is no difference in what happens either way). Unfortunately, the units always return to their EXACT starting position, rather than to a point "close enough" to it. The waypoints I give them SHOULD have a completion radius that prevents this from happening.
Here's the script if anyone is interested, it's still quite functional:
_man = _this select 0; _distMax = _this select 1; _distRet = _distMax * 0.85; _posStart = getPos _man; while {true} do { sleep 5; _compX = abs ((position _man select 0) - (_posStart select 0)); _compY = abs ((position _man select 1) - (_posStart select 1)); _distCurr = (sqrt ((_compX * _compX) + (_compY * _compY))); if (_distCurr >= _distMax) then { _grpNew = createGroup (side _man); _wptReturn = _grpNew addWaypoint [_posStart, 0]; _wptDismiss = _grpNew addWaypoint [waypointPosition _wptReturn, 0]; _wptReturn setWaypointType "MOVE"; _wptReturn setWaypointSpeed "LIMITED"; _wptDismiss setWaypointType "DISMISS"; _wptDismiss setWaypointSpeed "NORMAL"; _wptReturn setWaypointCompletionRadius _distRet; _wptDismiss setWaypointCompletionRadius _distRet; _grpNew setCurrentWaypoint _wptReturn; {[_x] join _grpNew} forEach units (group _man); }; };
Perhaps my question is simply this: When you switch units into another/their own group out of a "DISMISS" waypoint, do they always return to the exact position of the dismissal prior to executing further waypoints? Or am I just missing something?
And if so, does anyone know of a workaround to get them NOT to do so? i.e. something with a stop command or some such? Sorry I'm not more familiar with how to do a lot of things even after years of messing around with this game, lol.
-
As a quick followup to this, I have been thinking about using this in scenarios for some time since reading this thread and have quickly thrown together the following.
// Paste the following in "rebel.sqf" _grpLdr = _this select 0; _wpnCache = _this select 1; _grpThis = group _grpLdr; sleep (5 + (random 10)); _armWaypoint = _grpThis addWaypoint [getPos _wpnCache, 0]; _armWaypoint setWaypointCompletionRadius 2.5; _armWaypoint setWaypointType "MOVE"; _armWaypoint setWaypointBehaviour "COMBAT"; _armWaypoint setWaypointSpeed "FULL"; _armWaypoint setWaypointStatements ["true", "_grpOPFOR = createGroup east; (units group this) joinSilent _grpOPFOR; {_x addMagazine ""10x_303""; _x addWeapon ""LeeEnfield"";} forEach units _grpOPFOR;"]; _grpThis setCurrentWaypoint _armWaypoint;
Instead of my addweapon nonsense, you can just throw in a call to that armament script you linked and voila. Now whatever you want to give the civilians cause to arm themselves should just declare:
nul = [nameOfCivGroupLeader, nameOfObjectYouWantThemToGoStandNextTo] execVM "rebel.sqf";
Of course mine does it a group at a time, and arms them all identically. Just demonstrating the technique since I got it working as such ;p
Edit:
To elaborate, the first parameter sent to the sqf will name the leader of a group of civilians. When the script is called, the group led by that man will then run full speed to within 2.5 meters of whatever object is named in the second parameter sent to the script. Then, they will arm themselves as prescribed. Finally, since they're all still residually in "combat" mode, they will begin taking cover and engaging known hostiles (BLUFOR).
-
I am still almost positive I have seen AI identify other friendly AI as "unknown", just make a big DAC zone of friendly groups and walk around with a squad and see what they say.
EDIT: Sorry, didn't see this second page. I was a few hours late hahah.
-
That or perhaps a fix on the part of BIS. After all, what's the use of having an "Unknown" status anyway, if you automatically know they are all really Enemy?After reflection that would have to be my guess.
I am almost positive I have seen AI squad mates of mine refer to other friendlies as "Unknown" when I have been messing around in the editor on past occasions. Though that isn't scientific by any means, if that's what they're doing then it would indicate at least some of what you have tested and found is unique to the player.
I guess that way the player is only allowed to call out unknown enemy targets to avoid situations in which someone is spotting an enemy far away in a group of civilians, the radio message plays, and the player decides that the target has been revealed and no further spotting is necessary, but in reality the unit that got spotted was one of the civilians. The way it is now, that can't happen - you won't know you spotted the target until the message plays, which guarantees that you've spotted an enemy.
-
Can you not, then, simply script the civs to join a group of side east (assuming the player is side west) in order to avoid having to change the allegiance of the resistance faction?
-
One other thing you might try is putting the guys on the map as civilians, but then right before you use addWeapon to arm them, use commands to assign the units to (an) OPFOR group(s). That way they can be nice and docile until such time as they decide to arm themselves, when they will suddenly coordinate an attack on Western soldiers. :P
-
***UPDATED: 2/12/2011***
'mode' variable now takes up to three positions to the right of the decimal place. Script is MUCH more predictable and configurable; zones can be initialized to guarantee the same building placement as far as has been tested.
Since I was always confounded by the unintuitive process of setting up and debugging a Town Construction Set module every time I wanted to make a certain kind of random town, I figured some of the process could be automated within reasonable constraints to generate reasonably appropriate towns for a variety of situations.
Therefore I came up with, and present to you all, a very rudimentary script to set the initialization variables for Town Construction Set modules with only a single, higher-level line of code within the editor. To do this, make a file called "tginit.sqf" and paste the following code into it:
////////////////////////////////////// /// Town Generator Initializer /// /// Author: Darren Ringer /// ////////////////////////////////////////////////////////////////// // // // Instructions: place this file // // into your missions folder. // // Place a Town Construction set // // module on the map, and in its // // initialization field, put: // // // // nul = [this,<mode><.xyz>,<radius>] execVM "tginit.sqf" // // // // Where <mode> is determined as follows: // // 0 = sparse buildings // // 1 = loose suburban arrangement // // 2 = village-like arrangement // // 3 = dense, city-like arrangement // // This integer can optionally be followed by up to three // // decimal places (x, y, and z above), as follows: // // x :: if nonzero, scales the number of buildings, so // // mode 0.5 would make half as many buildings as // // would mode 0. // // y :: if nonzero, specifies a proportion of the number // // of buildings to be randomized, so mode 2.03 would // // generate 70 to 100% as many buildings as mode 2. // // z :: specifies proportion of random deviation from 1.5 // // (the default) as a multiplier to make trash and // // misc objects based on the total number of // // buildings created. // // and <radius> is simply the maximum distance from // // your Town Construction Set module at which you want // // buildings to be created. // // // // NOTE: Certain modes will not work below a certain radius // // size. In these cases, the mode will automatically // // get set to a compatible (denser) mode. No mode has // // been tested to be effective below a 50m radius. // // // ////////////////////////////////////////////////////////////////// // For example, // nul = [this, 1.735, 350] execVM "tginit.sqf" // in the init field of a Town Construction Modle // will create a town of radius 350 with mode 1 (scattered // buildings), 70% as many buildings as would normally be // in a mode 1 r 350 zone, 30% of which may or may not // exist, and 0.75-2.25x as many misc objects as // buildings. _tcs = _this select 0; _mode = _this select 1; _radius = _this select 2; _size = 2 * _radius; _miscrand = ((100 * _mode) % 1); _mode = _mode - (_miscrand * 0.01); _houserand = ((10 * _mode) % 1); _mode = _mode - (_houserand * 0.1); _scalar = (_mode % 1); _mode = _mode - _scalar; if (_radius < 100) then { if (_mode < 3) then { _mode = 3; }; }; if (_radius < 200) then { if (_mode < 2) then { _mode = 2; }; }; if (_radius < 400) then { if (_mode < 1) then { _mode = 1; }; }; switch (_mode) do { case 0: { tgdivisor = 90000; tgspac = 22; }; case 1: { tgdivisor = 46181; tgspac = 7.6; }; case 2: { tgdivisor = 22500; tgspac = 4; }; case 3: { tgdivisor = 2400; tgspac = 1.3; }; }; sCoeff = _scalar; if (_scalar == 0) then {sCoeff = 1;}; _count = (ceil (sCoeff * ((3.14159 * (_radius * _radius)) / tgdivisor))); _vals = [ _size, (ceil ((_count - (_count * _houserand)) + (random (_count * _houserand)))), 28, tgspac, (1.5 - (1.5 * _miscrand) + (3 * _miscrand)) ]; _vars = [ "townSize", "houseCount", "houseRotation", "houseSpacing", "coefMisc" ]; {_tcs setVariable [_x, (_vals select _forEachIndex)]} forEach _vars; tgdivisor = nil; tgspac = nil; sCoeff = nil;
Then in the init of each town generator you want to set up with the script, just put:
[this, <mode>, <radius>] execVM "tginit.sqf"
Where <mode> is an int [0-3] defining the density of the buildings (3 being the most dense), and <radius> defines the radius (NOT the "size") of the town. The module's internal variable appears to operate as a diameter and not a radius as indicated by the wiki. My script lets you think in terms of radii. :p
-------------------------------
Feature added 2/12/2011:
Instead of setting mode to a single integer like 2, you can now do something like:
[this, 2.905, 500] execVM "tginit.sqf"
This gets a little tricky, so let me break it down. If mode is represented as A.XYZ,
A :: [0-3] (general zone density, 3 being the densest of the four options)
X :: [0-9] (if zero, does nothing. if 1-9, scales the number of buildings 10% to 90%)
Y :: [0-9] (determines what percentage of the number of buildings in zone may or may not be generated. A 4 here makes between 60% and 100% as many buildings as a 0, for instance. this precludes the zone being generated the same every time.)
Z :: [0-9] (by default, misc objects are created as a quantity 1.5x that of the number of buildings. this num determines what percentage above or below 1.5x will be randomized in the same way houses are randomized by variable Y. a 5 here would use a random coefficient: from 0.75 to 2.22x the number of buildings, for instance.)
Thus, the example above will make a 500 radius zone with mode 2 density, 90% the typical number of buildings in such a zone, zero randomness as far as the number of buildlings, and 50% random deviance from the 1.5x multiplier to create misc objects.
The primary motivation for this change was so that zones will consistently load once you have them set the way you want them (assuming you use a 0 for the tenths place), and if you can't get a zone to load (too many rocks or mountains or something) you can just scale back the number of buildings in it until it WILL load.
-------------------------------
Suggestions, comments, flames, etc are more than welcome.
I understand that a lot of this could be cleaned up, improved, etc. It is very much a work in progress. But I have found it very useful already, and thought it might be of interest to others.
I would like to better understand the spacing variable and its effect, as well as potentially find a more elegant mathematical solution to scaling the different modes. The values right now were obtained through nothing but trial and error, other than the slightly less rational number 46181 that I got by working from a desired number of buildings in a specific size zone (75 in a zone of radius 1050, don't ask, haha).
The randomization elements cause the building placement to be slightly random, if you remove all the randomness you can consistently generate the same zone over and over. That could be handy if you want to populate some of the buildings with some exhausting use of the setpos command.
One thing to keep in mind here is that you can use some very large radii. Mode 0 on a 2400m radius circle sprinkles 200 buildings over a 7 square mile area. That's great for diversifying otherwise empty landscapes, especially maps that don't have many, or any, villages on them.
You can see below (hopefully, despite the terrible image quality thanks to MS Paint), an example of how this can be used. The big zone on the left is density 0; the medium red one is density 2, and the small green ones are density 3. Layering zones like this can cause errors, so you have to be careful with placement. (The colored zones are meaningless markers added for demonstration purposes only)
-
Those of you getting the problem with limited numbers of vehicle groups spawning compared to the number specified in the function call, I have a suggestion that has worked for me when I encountered that problem. I don't know about using the script only version of DAC (hadn't realized it was out yet, haha), but in the addon version this problem was solved for me by ensuring every DAC zone trigger had "time > 1" as its condition line. Any other value (such as times higher than 1, or the simple default condition "true") produced inconsistent results and errors like you describe.
-
Has anybody recently looked into adapting the fire control system from this mod into Arma2/OA? I'm not sure what exactly would go into doing something like that, but I'd imagine it would be possible (even if one were to stop short of converting any of the other modifications contained in this addon). This was probably my favorite mod from ArmA, and it would breathe so much more life into Arma 2.
-
Hrm, I'm noticing the lock effect SLIGHTLY, but when at high levels of zoom it's not even close to staying fixed on a single location. Whenever the UAV banks, the camera gets skewed in relation to the tilted axis. Also, unless I leave the UAV piloting AI enabled so that the UAV can dive bomb targets I'm "ordered" to fire upon, I am basically completely unable to target vehicles that I want to target. I'm not sure if the weapon system locks onto targets in front of the UAV itself or what.
I remember a UAV addon for the original ArmA that included a camera lock feature that worked PERFECTLY, unfortunately we didn't have the rest of a working UAV insfrastructure in place at the time to allow for complex UAV missions without elaborate amounts of scripting to along with it.
With the changes brought in Arma2 and OA, we are so close now I find it rather frustrating that basic functionality like this cannot be realized.
-
Well, since this thread is the most complete source of unit configs that I've been able to find, I figured I'd post a cleaned up version of all the OA units mentioned above, in a form that can be pasted directly into DAC_Config_Units.sqf. Since the code is just copied, I didn't translate anything, only adjusted formatting and syntax.
//-------------------------------------------------------------------------------------------------- case 9: { _Unit_Pool_S = [ "US_Soldier_Crew_EP1","US_Soldier_Pilot_EP1","US_Soldier_SL_EP1","US_Soldier_EP1","US_Soldier_GL_EP1", "US_Soldier_AR_EP1","US_Soldier_EP1","US_Soldier_LAT_EP1","US_Soldier_MG_EP1","US_Soldier_Sniper_EP1", "US_Soldier_AA_EP1","US_Soldier_HAT_EP1","US_Soldier_Medic_EP1","US_Soldier_Marksman_EP1","US_Soldier_AR_EP1", "US_Soldier_GL_EP1","US_Soldier_AR_EP1" ]; _Unit_Pool_V = [ "HMMWV_DES_EP1","HMMWV_M998_crows_M2_DES_EP1","HMMWV_MK19_DES_EP1","HMMWV_Terminal_EP1","HMMWV_TOW_DES_EP1", "HMMWV_Avenger_DES_EP1","MTVR_DES_EP1","M1030_US_DES_EP1"]; _Unit_Pool_T = [ "M2A2_EP1","M2A3_EP1","M1A1_US_DES_EP1","M1A2_US_TUSK_MG_EP1","M1126_ICV_mk19_EP1","M1126_ICV_M2_EP1"]; _Unit_Pool_A = [ "MH60S","UH1Y","AH1Z","MV22","AH64D_EP1","AH6J_EP1","AH6X_EP1"]; }; //------------------------------------------------------------------------------------------------- case 10: //Takistan_Bevoelkerung { _Unit_Pool_S = [ "TK_GUE_Soldier_TL_EP1","TK_GUE_Soldier_TL_EP1","TK_GUE_Warlord_EP1","TK_GUE_Soldier_EP1","TK_GUE_Soldier_2_EP1","TK_GUE_Soldier_3_EP1", "TK_GUE_Soldier_4_EP1","TK_GUE_Soldier_EP1","TK_GUE_Soldier_5_EP1","TK_GUE_Soldier_HAT_EP1","TK_GUE_Soldier_AR_EP1","TK_GUE_Soldier_MG_EP1", "TK_GUE_Soldier_AT_EP1","TK_GUE_Soldier_EP1","TK_GUE_Soldier_AAT_EP1","TK_GUE_Soldier_AA_EP1","TK_GUE_Soldier_Sniper_EP1", "TK_GUE_Bonesetter_EP1","TK_GUE_Soldier_MG_EP1","TK_GUE_Soldier_EP1","TK_GUE_Soldier_Sniper_EP1" ]; _Unit_Pool_V = [ "Offroad_DSHKM_TK_GUE_EP1","Offroad_SPG9_TK_GUE_EP1","Pickup_PK_TK_GUE_EP1","V3S_TK_GUE_EP1","Ural_ZU23_TK_GUE_EP1"]; _Unit_Pool_T = [ "BRDM2_TK_GUE_EP1","BRDM2_HQ_TK_GUE_EP1","BTR40_TK_GUE_EP1","BTR40_MG_TK_GUE_EP1","T34_TK_GUE_EP1","T55_TK_GUE_EP1"]; _Unit_Pool_A = [ "UH1H_TK_GUE_EP1"]; }; //------------------------------------------------------------------------------------------------- case 11: //Takistan_Miliz { _Unit_Pool_S = [ "TK_INS_Soldier_TL_EP1","TK_INS_Soldier_TL_EP1","TK_INS_Warlord_EP1","TK_INS_Soldier_EP1","TK_INS_Soldier_2_EP1","TK_INS_Soldier_3_EP1","TK_INS_Soldier_4_EP1", "TK_INS_Soldier_AT_EP1","TK_INS_Soldier_2_EP1","TK _INS_Soldier_EP1","TK_INS_Soldier_AAT_EP1","TK_INS _Soldier_AR_EP1","TK_INS_Soldier_MG_EP1", "TK_INS_Soldier_AA_EP1","TK_INS_Soldier_Sniper_EP1 ","TK_INS_Soldier_2_EP1","TK_INS_Soldier_EP1","TK_ INS_Soldier_MG_EP1","TK_INS_Bonesetter_EP1" ]; _Unit_Pool_V = [ "LandRover_MG_TK_INS_EP1","LandRover_SPG9_TK_INS_E P1","Old_bike_TK_INS_EP1"]; _Unit_Pool_T = [ "BTR40_MG_TK_INS_EP1","BTR40_TK_INS_EP1","BTR40_MG_TK_INS_EP1"]; _Unit_Pool_A = [ ]; }; //------------------------------------------------------------------------------------------------- case 12: //Takistan_Army { _Unit_Pool_S = [ "TK_Soldier_Crew_EP1","TK_Soldier_Crew_EP1","TK_Commander_EP1","TK_Soldier_SL_EP1","TK_Soldier_EP1","TK_Soldier_Medic_EP1","TK_Soldier_LAT_EP1", "TK_Soldier_B_EP1","TK_Soldier_MG_EP1","TK_Soldier_HAT_EP1","TK_Soldier_EP1","TK_Soldier_AMG_EP1","TK_Soldier_AAT_EP1","TK_Soldier_AR_EP1","TK_Soldier_AA_EP1", "TK_Soldier_GL_EP1","TK_Soldier_Engineer_EP1","TK_Soldier_Officer_EP1","TK_Soldier_Medic_EP1","TK_Soldier_Sniper_EP1","TK_Soldier_MG_EP1", "TK_Soldier_EP1","TK_Soldier_SniperH_EP1","TK_Soldier_Spotter_EP1","TK_Soldier_EP1" ]; _Unit_Pool_V = [ "LandRover_MG_TK_EP1","MAZ_543_SCUD_TK_EP1","GRAD_TK_EP1","LandRover_SPG9_TK_EP1","TT650_TK_EP1","V3S_TK_EP1","SUV_TK_EP1","UAZ_Unarmed_TK_EP1", "UAZ_AGS30_TK_EP1","UAZ_MG_TK_EP1","Ural_ZU23_TK_EP1","V3S_Open_TK_EP1"]; _Unit_Pool_T = [ "BTR60_TK_EP1","BMP2_TK_EP1","BMP2_HQ_TK_EP1","BRDM2_TK_EP1","BRDM2_ATGM_TK_EP1","BTR60_TK_EP1","M113_TK_EP1", "T34_TK_EP1","T55_TK_EP1","ZSU_TK_EP1","BTR60_TK_EP1","T72_TK_EP1"]; _Unit_Pool_A = [ "Mi24_D_TK_EP1","Mi17_TK_EP1","UH1H_TK_EP1"]; }; //------------------------------------------------------------------------------------------------- case 13: //Takistan_Army (+ Night_Soldiers) { _Unit_Pool_S = [ "TK_Soldier_Crew_EP1","TK_Soldier_Crew_EP1","TK_Commander_EP1","TK_Soldier_SL_EP1","TK_Soldier_EP1","TK_Soldier_TWS_EP1","TK_Soldier_Medic_EP1", "TK_Soldier_LAT_EP1","TK_Soldier_B_EP1","TK_Soldier_MG_EP1","TK_Soldier_Sniper_Night_EP1","TK_Soldier_HAT_EP1","TK_Soldier_EP1","TK_Soldier_AMG_EP1", "TK_Soldier_AAT_EP1","TK_Soldier_AR_EP1","TK_Soldier_Night_1_EP1","TK_Soldier_Night_2_EP1","TK_Soldier_AA_EP1","TK_Soldier_GL_EP1","TK_Soldier_Engineer_EP1", "TK_Soldier_Officer_EP1","TK_Soldier_Medic_EP1","TK_Soldier_Sniper_EP1","TK_Soldier_MG_EP1","TK_Soldier_EP1","TK_Soldier_SniperH_EP1", "TK_Soldier_Spotter_EP1","TK_Soldier_Night_2_EP1","TK_Soldier_EP1" ]; _Unit_Pool_V = [ "LandRover_MG_TK_EP1","MAZ_543_SCUD_TK_EP1","GRAD_TK_EP1","LandRover_SPG9_TK_EP1","TT650_TK_EP1","V3S_TK_EP1","SUV_TK_EP1","UAZ_Unarmed_TK_EP1", "UAZ_AGS30_TK_EP1","UAZ_MG_TK_EP1","Ural_ZU23_TK_E P1","V3S_Open_TK_EP1"]; _Unit_Pool_T = [ "BTR60_TK_EP1","BMP2_TK_EP1","BMP2_HQ_TK_EP1","BRDM2_TK_EP1","BRDM2_ATGM_TK_EP1","BTR60_TK_EP1","M113_TK_EP1","T34_TK_EP1", "T55_TK_EP1","ZSU_TK_EP1","BTR60_TK_EP1","T72_TK_EP1"]; _Unit_Pool_A = [ "Mi24_D_TK_EP1","Mi17_TK_EP1","UH1H_TK_EP1"]; }; //------------------------------------------------------------------------------------------------- case 14: //Takistan_Civilians { _Unit_Pool_S = [ "TK_CIV_Worker02_EP1","TK_CIV_Takistani02_EP1","TK_CIV_Worker02_EP1","TK_CIV_Woman01_EP1","TK_CIV_Worker01_EP1", "TK_CIV_Takistani03_EP1","TK_CIV_Takistani01_EP1","TK_CIV_Woman03_EP1","TK_CIV_Takistani05_EP1","TK_ CIV_Takistani04_EP1", "TK_CIV_Takistani06_EP1","TK_ CIV_Woman02_EP1" ]; _Unit_Pool_V = [ "Ikarus_TK_CIV_EP1","Old_moto_TK_Civ_EP1","Old_bike_TK_CIV_EP1","Lada1_TK_CIV_EP1","Lada2_TK_CIV_EP1","LandRover_TK_CIV_EP1", "TT650_TK_CIV_EP1","VolhaLimo_TK_CIV_EP1","hilux1_civil_3_open_EP1","S1203_TK_CIV_EP1","SUV_TK_CIV_EP1", "Ural_TK_CIV_EP1","V3S_Open_TK_CIV_EP1","Volha_1_TK_CIV_EP1","Volha_2_TK_CIV_EP1"]; _Unit_Pool_T = [ ]; _Unit_Pool_A = [ ]; }; //-------------------------------------------------------------------------------------------------
If you just have the stock DAC_Config_Units.sqf from the package, this can get pasted directly after case 8 and more or less seamlessly activate all the OA units for selection.
EDIT: Wouldn't you know it, there were typos in the code I originally posted... :j: Aside from correcting the typos I had above, I also noticed that the following needs to be put in your DAC_Config_Creator.sqf file, assigned to the variable DAC_SP_Soldiers (basically, replace the DAC_SP_Soldiers line in the file with the following line):
DAC_SP_Soldiers = ["RU_Soldier_MG","USMC_Soldier_MG","GUE_Soldier_MG","CDF_Soldier_MG","Ins_Soldier_MG","US_Soldier_MG_EP1","TK_GUE_Soldier_MG_EP1","TK_INS_Soldier_MG_EP1","TK_Soldier_MG_EP1","TK_Soldier_AMG_EP1"];
-
I would have to say that the improvements to infantry AI, scripting innovations like all the new modules and functions we get, and the new briefing system that all come with ArmA 2 put that game squarely in first place (for me, by a fair margin). I never used any mods with OFP, but let's face it, modding possibilities in ArmA 2 vastly surpass those of OFP, whether or not they are fully realized yet. ArmA 1 is a different matter; it felt very "forced" by the devs IMO, and rather unfinished (certainly unpolished even after myriad mods and patches). ArmA 2 has a lot of polish compared to that, and frankly OFP was a bit crude itself.
A lot of apprehension I had about ArmA and ArmA 2 at first definitely came from poor system performance. Automatic weapons, when a system is bogged down, simply feel clunky, broken, and unreliable. Firing rates are quite variable from what I've seen (even though I am sure this would take on a deeper significance in multiplayer; I only play with the editor, SP). Drop your monitor resolution way down, or get a new video card, and make sure your processor is keeping up with everything, and the game instantly feels a LOT more "fun", "immersive", "polished", etc. It's amazing how those extra frames per second increase the realism factor.
-
wouldn't you just want that highlighted line to read
EXLZ2 setpos _EXLZ2pos;
? If I'm reading this correctly, _LZpos is never changed by your code, and the _EXLZ2pos variable is the one you have modified to give the position you want.
-
Hmm, it would be pretty awesome to have some kind of reference for that kind of thing, but as far as I know there isn't one. What I generally do is put down a 2-man group with myself as the leader and just go around seeing what positions I can tell my squadmate to move into. Then I make a note of the position numbers that look useful and use those in the editor. Not very efficient, I'll give you that :(
-
Hmm, from looking at the other post:
(group player) setvariable ["MARTA_CUSTOMMARKER","b_recon"];
I would take that to mean that every group has a variable called "MARTA_CUSTOMMARKER" that stores any custom icon to use in, for instance, the High Command interface. The above example would, thus, be pretty limited based on my understanding, and might be better replaced with
(group this) setvariable ["MARTA_CUSTOMMARKER", "b_recon"];
into the Initialization field of any unit (by convention, the leader) of the group you want represented by said custom icon. "b_recon" would of course be replaced by the marker icon of your choice (don't ask me where to find the names, haha)
-
One problem I've been having with the High Command module arises from creating playable units in charge of subordinate groups.
Is there a way to reliably team switch from the commander to a playable leader of a subordinate group, and then back again, and continue to issue commands to the group containing the playable man?
Take, for example, the following setup:
(Squad Leader + Fireteam) PLAYER AS LEADER | [HC:C Module] | [HC:SUB Module] / \ (Sniper Team) (Sniper Team) PLAYABLE LEADER PLAYABLE LEADER
(other groups omitted to save space)
Now, this gives me (the squad leader) precise control over the members of my fire team, and as long as I remain as the squad leader I can order around my sniper teams however I want using the High Command interface.
At any time I can also switch to one of the snipers to personally control him. The problem is that once I have done this, and then switch back to the commander, the sniper team no longer follows my orders. The waypoint will show up on the map, but the team will not move to it. Members of the team other than the leader will move so that the group formation faces the next waypoint, but they will not break formation to go there, and the leader stands perfectly still.
There is one thing I have to add to this which may help clarify what is going on here. When i set the "chainofcommand" variable on the HC:C module to TRUE, then the group members (NOT the leader) WILL continue to follow waypoints that I assign. The leader who I had controlled still will not move, however, and the group icon in the HUD/Map remains centered on the leader with no indication that my orders are being carried out by his subordinates.
-
Perhaps there's a driver update you could download for your memory controller; other than that it could be any number of drivers that could be causing such a problem. Try without the overclocking as above, and if that doesn't work search for all the drivers you can find to update paying special attention to drivers for your chipset/motherboard/etc.
Of course I've had memory controller issues in the past that never got resolved until I upgraded to Windows Vista, but that's neither here nor there, just an indicator of how frustrating it is ;p
-
On a tangent, getting AI snipers to fire and move, fire and move, would be a nightmare of scripting and editing IMO. It took me long enough just to get working AI snipers at all
Of course, you could always have them randomly move around and just "vanish" in between spots where they're shooting from, but that's a little bit unfair in terms of realism or game mechanics....
Vehicle Weapon
in ARMA 2 & OA : MISSIONS - Editing & Scripting
Posted
Well if all you want to do is fire the missile, you can use the "addaction" command to create an option that runs a script to create the missile. You'll have to do some math but you should be able to get it to create the missile somewhere just in front of your bike (NOT on the ground.. you will probably need getDir, getVelocity, setVelocity, getPos, setPos, and a working knowledge of the pythagorean theorem). Just give the missile a realistic velocity based on how you want it to go (probably just a good sized vector in whatever direction the bike is facing). If you can actually work out how to do this given the above information, you're well on your way to scripting just about anything. If not, keep searching the forums until A) you find an alternative solution, or B) you figure out how exactly to do it that way. haha. Good luck to you.