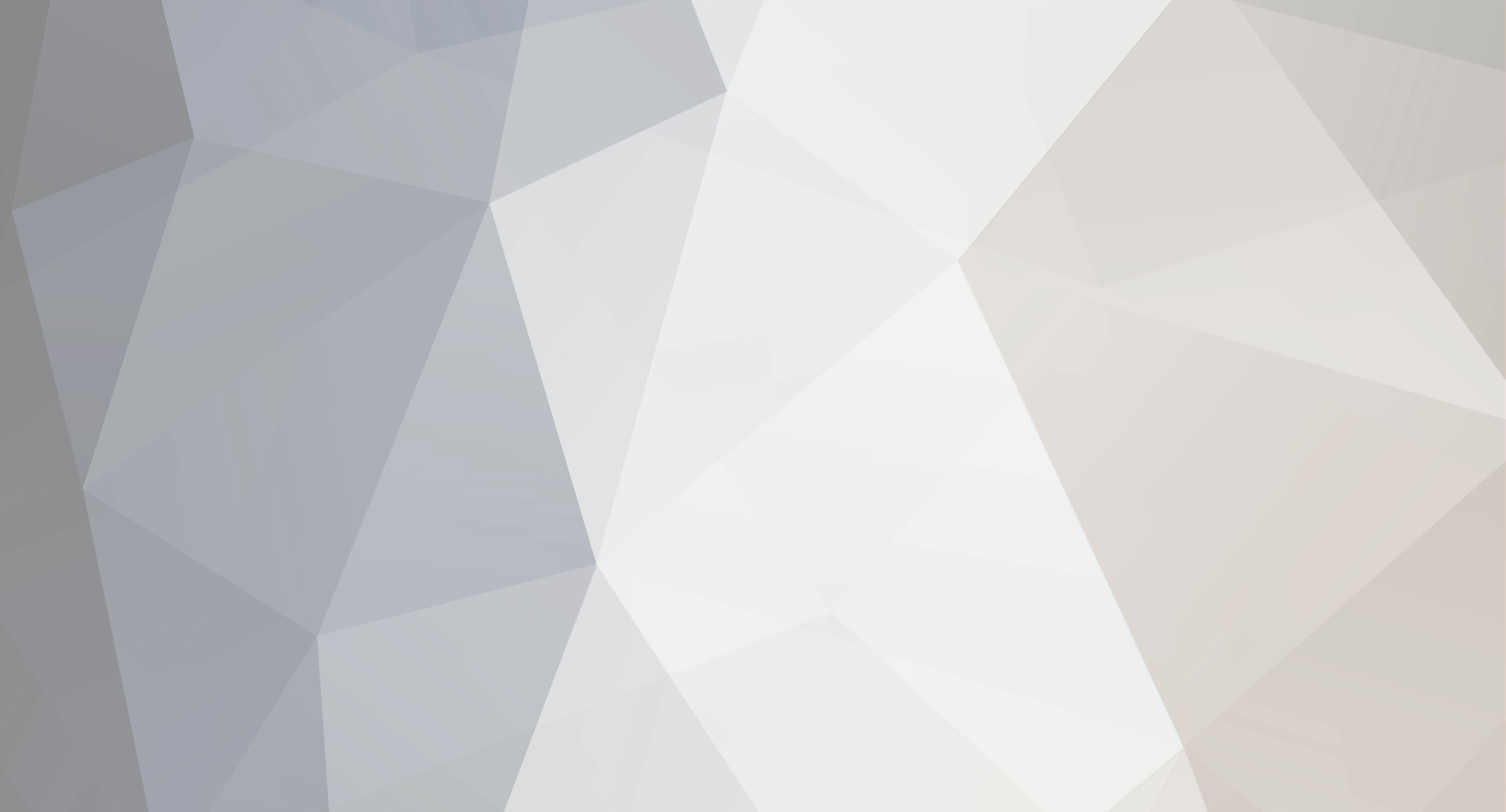

ZaellixA
Member-
Content Count
361 -
Joined
-
Last visited
-
Medals
Everything posted by ZaellixA
-
Spawning items at player corresponding to what random number they get?
ZaellixA replied to _HXPE's topic in ARMA 3 - MISSION EDITING & SCRIPTING
I get it but keep in mind that despite the fact that you want to add 20 different outcomes of a dice the result can be identical if the distribution of the values returned by random is uniform in all cases. So, if you really intend to group the results then using only 5 (or as many as you see fit) cases will make your code shorter and more easily maintainable. Nevertheless, you are, of course, free to pick your preferred style as long as it provides the same results. Most probably no... There must be another issue as Harzach's code should work properly (unfortunately on Mac right now so I can't test). You should make sure you have access to the unit (if you run an MP mission you may have to remoteExec) and that everything is passed correctly to your script. Please let us know if you manage to find what the problem is (for the sake of other people's benefit in the future). -
Spawning items at player corresponding to what random number they get?
ZaellixA replied to _HXPE's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Well, first of all, since many cases are identical you could just narrow them down to only 5, which I believe is the number of different hints you provided. This could be done in one of the two ways (possibly more but I can only think of those two right now) 1. Use something like floor random 5 to get only 5 different numbers. Alternatively you could do something like floor ((random 1000) % 6), which I am not sure whether it provides better random results (also, I am not sure what the result of modulus command is when used with floats). 2. Do something like case 1; case 2; case 3: {blah blah blah}; case 4; case 5; case 6; case 7: {blah blah blah}; which effectively groups the cases. You can also have a look at the random command documentation for more info and random generation alternatives. Hope this helps somehow. P.S.: I'm sorry for the formatting but I'm on my phone and don't have all forums features available. -
Game option - difficulty - commands
ZaellixA replied to Migal's topic in ARMA 3 - QUESTIONS & ANSWERS
Well, if you are not sure what the explanation means, as an example think of the red squares that appear when the group leader assigns you a target (with the distance being shown underneath). Pretty much all commands assigned from the group leader will show up in a similar manner. This is what this option is about. If you disable it you will never see those indications on your GUI. Hope this clarifies it a bit 🙂 -
Check if player killed more than 2 friendly units
ZaellixA replied to SlovakianLynx's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hhhmmm, I am not really sure why this happens. I believe that your conditions shouldn't really have anything to do with the rating of the player... Nevertheless, you could possibly try to use the rating too, although as I just said I am not sure this is the issue. Try adding something like (rating player) > -2000 I am not sure this will achieve what you want to do though because in this way if you kill all those friendlies and then leave the area the trigger will not fire. This kinda breaks the code rather than fixes it. I would suggest you check what thisList contains and whether the player is in the list after the rating goes below -2000, or there is another condition (from the game engine point of view) that removes the player from thisList. -
Check if player killed more than 2 friendly units
ZaellixA replied to SlovakianLynx's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Yep 7erra is right about it. Most often, the reason of getting a "Generic Error" for a sleep command is because you use it in an unscheduled environment. And, once more, what 7erra suggests is the simplest and most direct solution to that. An alternative to that, in order to make your code a wee bit more generic (or not...) is to use canSuspend command like // Some code if (canSuspend) then { uiSleep 1; // Or you can use either sleep or waitUntil }; This way, you won't get any errors from your code and will still wait for the specified amount of time if this is possible. -
Check if player killed more than 2 friendly units
ZaellixA replied to SlovakianLynx's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hey 7erra, that's good to know. I apologise for not being able to provide that info in advance, I was not aware of that subtlety :(... You could easily verify the dead unit's side just by placing systemChat str (side _killed); in the event handler after acquiring the passed parameters. This way you don't even have to check the diag_log file. Please let us know if this worked for you somehow, it could possibly benefit more people in the future. -
Check if player killed more than 2 friendly units
ZaellixA replied to SlovakianLynx's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Oh, I am sorry, I didn't realise you were to use it on SP 😞. I am not sure initPlayerLocal.sqf runs on SP, but in this case you could place it in your init.sqf file. You should get the same results. But now that I think about it, most probably this won't be of much help, since you don't want to find out when the player shoots other players but when the player shoots other friendly AI units. I apologise for all that, I didn't correctly understand your intend. So, in this case, apart from checking the rating of the player, which is what 7erra very well suggested, you could use the mission event handler "EntityKilled", which runs when an entity is killed. This way you could check, and save in a variable like I suggested, the amount of kills of the player towards friendly units. This could possibly look like /* * Inside init.sqf */ // Add the mission event handler addMissionEventHandler ["EntityKilled", { // Get the passed parameters of interest params ["_killed", "", "_instigator"]; // Check if instigator is player and the killed unit was on the same side with the instigator/player if ((_instigator isEqualTo player) && ((side _instigator) isEqualTo (side _killed))) then { // Get the amount of friendly kills of the player private _nKills = player getVariable ["YOU_nKills", 0]; // Check amount of kills if (_nKills >= 1) then { systemChat "Game Over..."; // DEBUGGING MESSAGE /* * Instead of the message finish the game or do whatever you intend to do */ }; // Set the variable with the new number of kills player setVariable ["YOU_nKills", _nKills + 1]; }; }]; Please keep in mind that, once more, the presented code is untested and should be treated with caution. Do not hesitate to send again if this does not work or needs further refinement and improvement. Best, Z. -
Check if player killed more than 2 friendly units
ZaellixA replied to SlovakianLynx's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hey there, 7erra gave you a good option there. Alternatively you could possibly use the "Killed" event handler to count how many friendly units one has killed. This could possibly look something like // In initPlayerLocal.sqf // Add the event handler to the player player addEventHandler ["Killed", { // Get some variables private _instigator = _this select 2; // The one who killed the player private _nKills = _instigator getVariable ["YOU_nKills", 0]; // Get the amount of kills of the instigator // Check if the player has killed at least one player before if (_nKills >= 1) then { // Do whatever you want to do }; // Increment the number of kills of the instigator _instigator setVariable ["YOU_nKills", _nKills + 1]; }]; Please keep in mind that this is a simple example and that you may have to do some more things to take care of the possibility of someone driving over someone else, or being a UAV operator and stuff like that. Additionally, you may have to use remoteExec if you want to do something to the instigator. Finally, note that this snippet is untested and has to be treated with caution. Do not hesitate to ask for more help and/or directions if you either require more help or want to extend the solution. -
How I Can Evaluate The Performance Of Team Members
ZaellixA replied to hshaban3's topic in ARMA 3 - GENERAL
You could save all the information in one or more variables. I would use an an array 'cause it makes more sense to me but feel free to use more than one variables if it suites your needs best. I don't really know what information you would like to evaluate so I can't really make more suggestions as to how you could possibly get that information but a demo variable assignment would look like the following // I put some dummy information in an array private _info = [3, // Kills 6, // Deaths 143, // Bullets fired 3/6]; // Kill-Death ratio // Save the information in the profile namespace profileNamespace setVariable ["Unit_Info", _info]; // Save the profile namespace saveProfileNamespace; This way you can access the information saved in the profile namespace and get the information from there. One detail here is that I am not sure whether you should first convert all the info in the variable to a string or not. This should be checked first. -
Please correct me if I am wrong but I believe that quotation marks can be omitted. I mean that you can actually create an array to hold the actual objects and not their names as strings. Of course, what is "best" depends on the intended behavior.
- 5 replies
-
- 1
-
-
- eden editor
- variable
-
(and 1 more)
Tagged with:
-
setBehaviour vs setCombatBehaviour
ZaellixA replied to Luft08's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Well, that's a reasonable guess. I believe testing is needed here :). I'll let you people know if I manage to get any meaningful results. -
setBehaviour vs setCombatBehaviour
ZaellixA replied to Luft08's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Aaaaahhhhhh OK... now it makes sense, thanks. Not sure what the difference is in effect if you set the behaviour to the group or all the units in the group but from a "technical" point of view it makes sense. Thanks for the clarification. -
setBehaviour vs setCombatBehaviour
ZaellixA replied to Luft08's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Thanks for the clarification. But I do have a question. How does setBehaviour affects the behaviour of the rest of the units in the group? Referring to MadRussian's comment below -
setBehaviour vs setCombatBehaviour
ZaellixA replied to Luft08's topic in ARMA 3 - MISSION EDITING & SCRIPTING
As Ibragim A states, the main difference, based on BIKI's entries, is that with setBehaviour you actually set the behaviour to the whole group regardless if it is used on a group or a unit in the group. As the comment of MulleDK13 states In contrast, with setCombatBehaviour you can either set the behaviour of a single unit or of the whole group. Similar to setBehaviour one can use setBehaviourStrong to enforce the behaviour of a unit and try to do the same to the whole group. To be honest, I am not sure what the difference between setBehaviour and setBehaviourStrong is but I assume that the latter is more "enforcing" than the former. Please keep in mind that my post is based on assumptions made based on the BIKI's entries, thus a wee bit of testing for your specific purpose could prove to be quite beneficial... -
How can I delete “extra” units after an Array has been Resized?
ZaellixA replied to Blitzen88's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Well, I guess that a combination of opusfmspol's and pierremgi's suggestions make a quite nice solution to your problem at hand. It could look something like // Your code private _trenchUnits = units group _Squadleader; // Pierremgi's code private _units = units group leader; private _trenchPositions = getpos _SquadLeader nearObjects [_TrenchPosObject, _Radius]; private _trenchPositions = _trenchPositions call BIS_fnc_arrayShuffle; // Pierremgi's code adapted based on opusfmspol's code { deleteVehicle _x; } forEach (_trenchUnits - ((+_trenchUnits) resize _trenchPositions)); Not sure this is a good or bad solution but, at least to me, it looks like a quite compact one. It's up to you to decide whether it is OK for you or not and of course the rest of the contributors might have something to add or suggest. -
How can I delete “extra” units after an Array has been Resized?
ZaellixA replied to Blitzen88's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hey there @pierremgi. Nice piece of code there but I have a question... I don't really get the _x setPos getPos _pos; line. Isn't _pos already a position? If yes, why should you use getPos again? If not, then what does it contain (since in the previous line you initialise _pos with the result of a getPos command)? This part got me kinda confused... Could you please clarify that? Thanks in advance :). -
getPlayerScores -- has me totally baffled.
ZaellixA replied to ROGER_BALL's topic in ARMA 3 - MISSION EDITING & SCRIPTING
I believe the issue here is that the part of the code that increments the numDeaths parameter never runs. Most probably this happens because instead of passing the object to the function you call you pass the name of the object. Thus, the commad isPlayer does not return true and the part of the code that increments the numDeaths parameter never runs. See the BIKI pages of isPlayer and name commands and you will see that one returns a string (the name command that is) while the other expects an object (the isPlayer command). There is a high probability that you haven't enabled the option to be shown the errors that occur during gameplay and if this is the case you wouldn't be able to "catch" that. Now, apart from that I will show you one more way to perform the checks which I personally prefer. This being said, I don't claim that this is neither cleaner nor more efficient code than what you have presented here. I just prefer to do it in the way I present below. Instead of making all those checks I prefer to put the names of the objects/units in an array and use findIf to directly find the position of the object in the array. This is good only for the checks you do for the players. So, copying and adapting your code, I would do like that (showing only the event handler part) addMissionEventHandler ["EntityKilled", { params ["_killed", "_killer", "_instigator"]; if (isNull _instigator) then {_instigator = UAVControl vehicle _killer select 0}; // UAV/UGV player operated road kill if (isNull _instigator) then {_instigator = _killer}; // player driven vehicle road kill /* * BELOW IS THE PART I CHANGED */ // Create an array with the names of the players. This could also be a global variable private _playas = [ROGER_BALL, EL_GUAPO, CRESTRUCTOR, TYRONE, DOC_1]; private _check = _playas findIf {_x isEqualTo _killed}; // Check if the dead was in the array and get the loadout if (!(_check isEqualTo -1)) then { _killed setVariable ["PATROL_LOADOUT_" + (str _killed), getUnitLoadout, _killed]; }; // Check if the killer was one of those that we care about (reuse variable) _check = _playas resize ((count _playas) - 2); // Discard DOC_1 _check = _check findIf {_x isEqualTo _instigator}; // Check for instigator /* * The above could be one-lined like this * _check = (_playas resize ((count _playas) - 2)) findIf {_x isEqualTo _instigator}; */ // Print "hint" to all players as to who killed whom if (!(_instigator isEqualTo -1)) then { hint format ["%2 eliminated %1.", name _killed, _instigator]}; //########################################################## //########################################################## //################## Bohemia Forum ################## //########################################################## //########################################################## // finally, track nr kills by calling code routine below. [_killed] call incDeath; // REMOVED THE "NAME" COMMAND HERE IN ORDER TO PASS THE OBJECT AND NOT ITS NAME TO THE CODE systemChat str numDeaths + " " + name _killed; //########################################################## //########################################################## //########################################################## //########################################################## //########################################################## }; ]; // end addMissionEventHandler "EntityKilled" Not sure this seems better or worse to you, but I thought I should just show you a different way to achieve what you had already achieved. To me, it's good to see how other people do things in order to "broaden my view" and learn new things and commands. If it seems weird and/or you don't want to see another way just ignore it. Hope this whole thing helps somehow. If there's anything else you would like clarified or you want further help please don't hesitate to ask. Finally, please keep in mind that this code snippet is untested so please treat it with extra caution. -
Extend/reset sleep timer?
ZaellixA replied to GaryTheNoTrashCougar's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hhhhmmmm, it seems that, since the variable killType is set inside the EntityKilled event handler, but is done so after its first call, which is somewhere in the first lines of the code in the event handler. This means that the first time it is called is nil while the second time you call it has the information of the first call. I haven't tested it but I believe that this is the issue here... One additional comment is that you could set the killType = "HEADSHOT"; right after the "head check". This could look like (copying your code) if (head) then { killType = "HEADSHOT"; // Here go all the other checks you do }; The same goes for the "EXPLOSIVE KILL" which could go right after the "frag check". Neither the result nor the efficiency of the code will change but it is good coding practice to avoid redundancy whenever possible. Most often than not this makes code more readable and easier to debug/maintain. This way, if you decide to change the "title" of the kill you will have to do it only in one place thus avoiding "bugs" (well, this may not qualify as a bug since it won't affect the behavior of the code but I believe you get the idea). Please let us know if this solves your issue or you may need further assistance. -
Extend/reset sleep timer?
ZaellixA replied to GaryTheNoTrashCougar's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Not sure this helps or not because I am not sure I have completely understood the purpose of your code. Nevertheless, one way to reset the counter on every kill is to use the Bohemia's function BIS_fnc_countDown like you found out yourself in conjunction with the "EntityKilled" mission event handler. This could possibly look like the following // Declare some global parameters score = 0; // Add event handler to reset timer and increment the score addMissionEventHandler ["EntityKilled", { params ["_unit"]; // Check if killed unit is the player if(!(_unit isEqualTo player)) then { score = score + 1; // Increment score [5 false] call BIS_fnc_countDown; // Reset counter } else { // If player was killed score = 0; // Reset score }; }]; // "Spawn" code to run [] spawn { // Run "forever" while{true} do { // Check if timer has reached 0 if (!([true] call BIS_fnc_countDown)) then { score = 0; // Reset score }; // Sleep to spare some CPU cycles uiSleep 1; }; }; You could place the presented code in init.sqf or use as you see fit. Please note that this is good for single player. If you try to use it on multiplayer (place the script in initPlayerLocal.sqf) the event handler will be called even if another player kills a unit which I am not sure whether it constitutes intended behavior or not... Just to make sure everything is understood I will try to briefly explain all parts of the code... 1) Create a variable to hold the score (you already have that)... 2) Add an event handler that will be automatically called by the engine when an entity is killed. In the event handler you check that the unit killed is not the player and if this is the case you increase the score and reset the counter. Otherwise you reset the score (player died). 3) Then you spawn some code that runs continuously and in there you check if the timer has reached 0. In this case you reset the score. Finally, you can change the sleep duration if you see that the code is very slow to respond. Hope this provides some insight in a different solution to your problem. Not sure if this is optimal in any sense as it constantly (every roughly one second) checks the timer. Additionally, please keep in mind that this snippet is not tested so it should be treated with caution. Oh, and you should also add all the "print" calls in the mission event handler. -
getPlayerScores -- has me totally baffled.
ZaellixA replied to ROGER_BALL's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hehe, Harzach is absolutely right, didn't spot that 8|...!!! Additionally, when I mentioned the initPlayerLocal.sqf I meant the one that is mentioned in this page. initPlayerLocal.sqf is, along with init.sqf and a whole bunch other scripts (you can find most of them at the provided link), is a script that is automatically called by the engine locally (in each player's machine and not on the server) when a player joins the game and the "player" is initialised. All you have to do is create such a script and place it in the same folder your mission .sqm is and it will be automatically called. Thus, if you place the provided code in this script it will be automatically called for each player that joins the game, saving you the burden to manually add it to all the playable units' init box in the editor. Not sure you already know that but from your last post I understood that it is not very clear to you. Apologies if I am wrong there... Finally, if you want to put the code anywhere but the event handler you could do something like // Keep score of deaths numDeaths = 0; // Put the code into a variable incDeath = { // Get the passed parameter (normally the unit) _unit = _this select 0; // Check if the unit is a player if (isPlayer [_unit]) then { numDeath = numDeath + 1; // Increament the number of deaths }; }; // Call the code inside the even handler addMissionEventHandler ["EntityKilled", { params["_killed"]; [_killed] call incDeath; }]; Hope this helps somehow and I haven't complicated things unnecessarily...!!! -
First time poster require Popup target scripting help
ZaellixA replied to TGxBrK's topic in ARMA 3 - MISSION EDITING & SCRIPTING
The guy in the video seems to be using a trigger to do the work. Additionally, he shows exactly the syntax you have to use to call the script. Would you care to pinpoint which part of the video you didn't understand so that we may help you clarify things? -
getPlayerScores -- has me totally baffled.
ZaellixA replied to ROGER_BALL's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hello ROGER_BALL and welcome to the forums. As Harzach mentioned per the BIKI, when used in single player getPlayerScores returns an empty array, which, whether it is turned to '0' or '[]' when converted to string, it is definitely not what you want. I haven't tested it but I believe your code should work when used in multiplayer. In case it doesn't, an alternative would be to create a global (whether you will make it public or not depends on your needs) variable to hold the number of deaths. This could possibly look like (I present the event handler with just this part of the code as I do not know what else you have included in yours). // Create the global variable to hold number of deaths numDeath = 0; addMissionEventHandler ["EntityKilled", { // Get the killed unit private _killed = _this select 0; // Check if it is a player who died if (isPlayer [_killed]) then { numDeath = numDeath + 1; // Increament the number of deaths }; }]; Some comments on the code presented above... 1) The mission event handler will run locally where it was added. This means that you will have to add it to every player, making it a very good candidate for the initPlayerLocal.sqf. I assume you already know that. 2) Please note the syntax of isPlayer. I have placed the killed unit in an array. This is per the alternative syntax presented in the BIKI of the isPlayer command. When this syntax is used, it doesn't matter whether the unit is alive or not. I have used it because I haven't tested the code and I am not sure whether in the "original" syntax the state (alive or dead) of the unit matters. 3) Finally, as per the BIKI again, please note that This means that if you intend to call the command from another client (or the server) you may have to do some extra checks. Some final notes. The code presented here is not tested, thus it should be treated with care and run some tests with it. Not sure this provides a solution to your problem as I don't have enough information on how you intend to use the commands and what exactly you are trying to achieve (present the total deaths to everyone, present each player with their own deaths, something else?). I do hope this helps somehow. Please don't hesitate to ask for more help, clarification or throw some more info and "re-initiate" the conversation. Best... -
Enable dynamic simulation for a script-spawned unit
ZaellixA posted a topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hey all... According to BIKI, one can use the enableDynamicSimulation command to turn on/off the dynamic simulation of an object. It seems that one can use a group or object as the target of the command but the comments above dictate that the object cannot be an AI. If this is true, how can one set the dynamic simulation on or off for a single AI entity? Thanks in advance... 🙂 -
Enable dynamic simulation for a script-spawned unit
ZaellixA replied to ZaellixA's topic in ARMA 3 - MISSION EDITING & SCRIPTING
Hhhhmmm, probably you were right... I was confused with the photo of the BIKI page that has "enable dynamic simulation" option for a HEMTT, which I suppose is considered to be an object and not a unit. Thanks for the clarification...!!! -
Enable dynamic simulation for a script-spawned unit
ZaellixA replied to ZaellixA's topic in ARMA 3 - MISSION EDITING & SCRIPTING
So that means that there's no choice to apply it to specific units? Of course all units belong to a group (even if they solo it) which would allow for enabling/disabling dynamic simulation but it seems quite counter-intuitive to be able to enable/disable dynamic simulation on specific units in 3den but not through script. I guess that going for group dynamic simulation is a rather OK "workaround" but still seems quite "not normal". Thanks for the suggestion